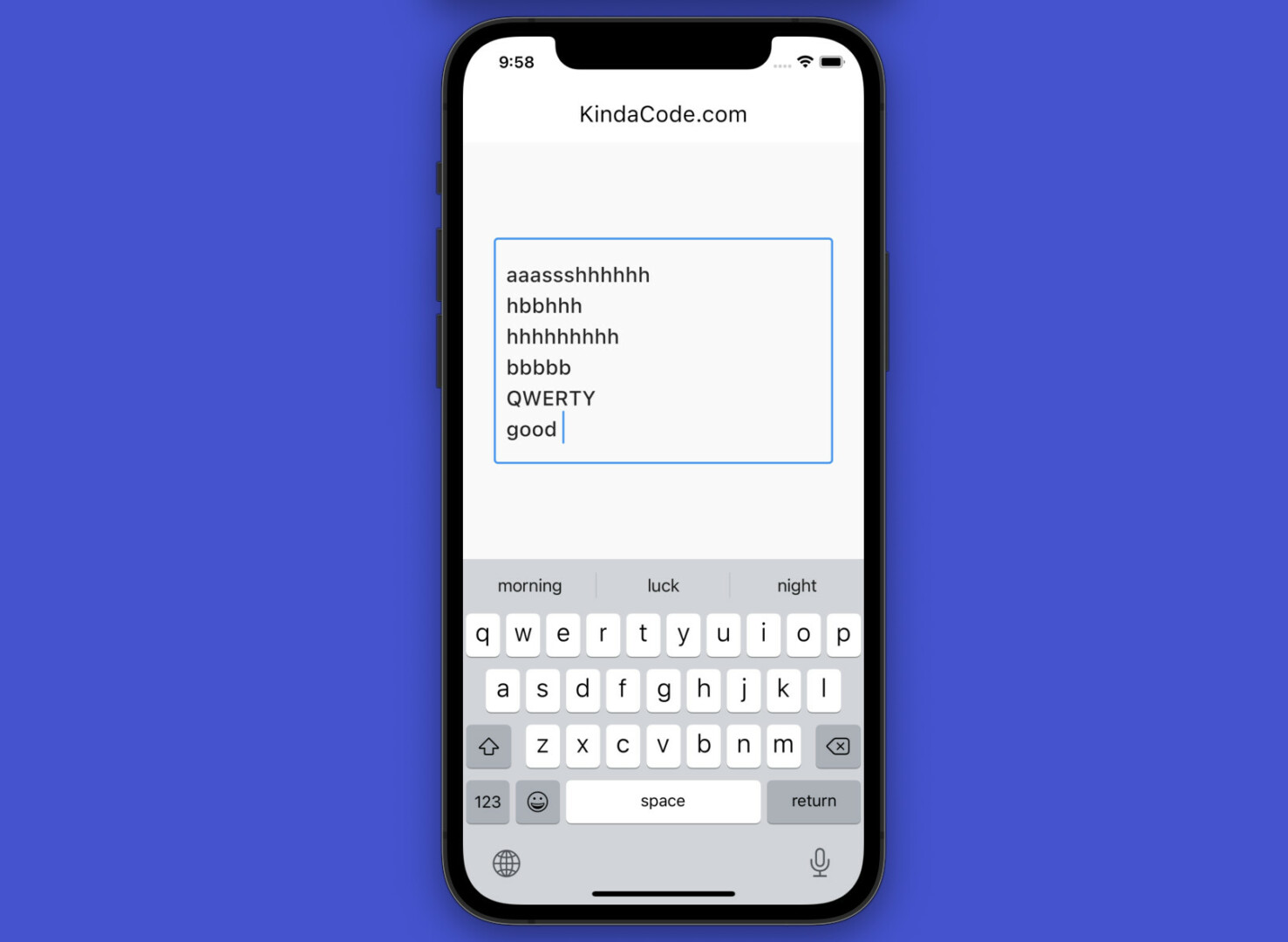
In Flutter, you can create an auto-resize (as needed) TextField (or TextFormField) in one of the following ways:
- Set the maxlines argument to null. The text field can expand forever if a lot of text is entered.
- Set minLines to 1 and maxLines to N (a positive integer). The text field will automatically expand or shrink based on the length of the entered text. It will stop expanding and start scrolling if it reaches N lines.
The example below will demonstrate the second approach.
Example Preview:
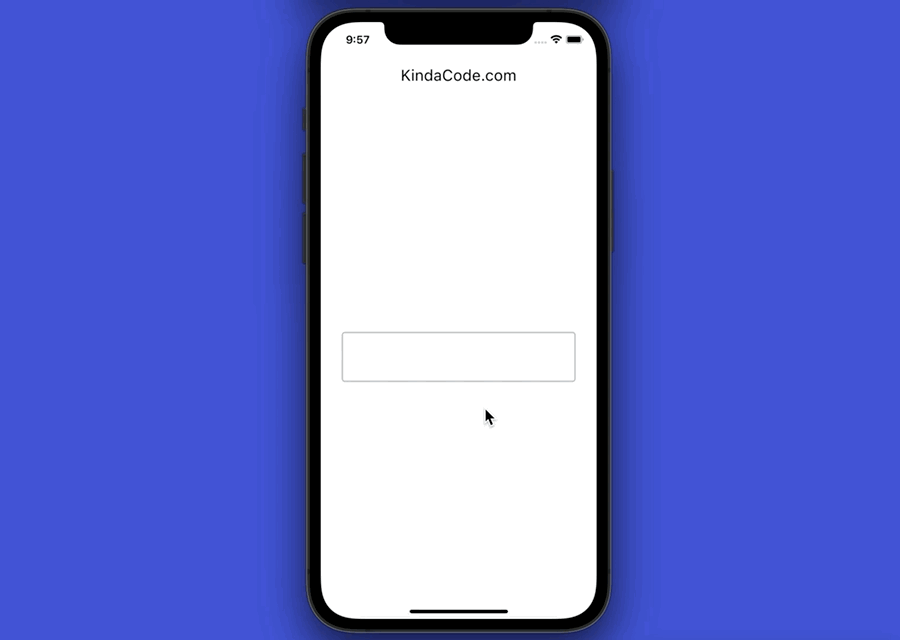
The code:
// kindacode.com
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
// hide debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
// enable Material 3
useMaterial3: true,
primarySwatch: Colors.blue,
),
home: const KindaCodeDemo(),
);
}
}
class KindaCodeDemo extends StatelessWidget {
const KindaCodeDemo({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: const Padding(
padding: EdgeInsets.all(30),
child: Center(
// implement the text field
child: TextField(
minLines: 1,
maxLines: 10,
style: TextStyle(fontSize: 20),
decoration: InputDecoration(border: OutlineInputBorder()),
),
),
),
);
}
}
Further reading:
- Flutter & Hive Database: CRUD Example
- Flutter: Customizing the TextField’s Underline
- Flutter: Make a TextField Read-Only or Disabled
- Flutter: Adding a Clear Button to a TextField
- How to make rounded corners TextField in Flutter
- Flutter: Show/Hide Password in TextField/TextFormField
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.