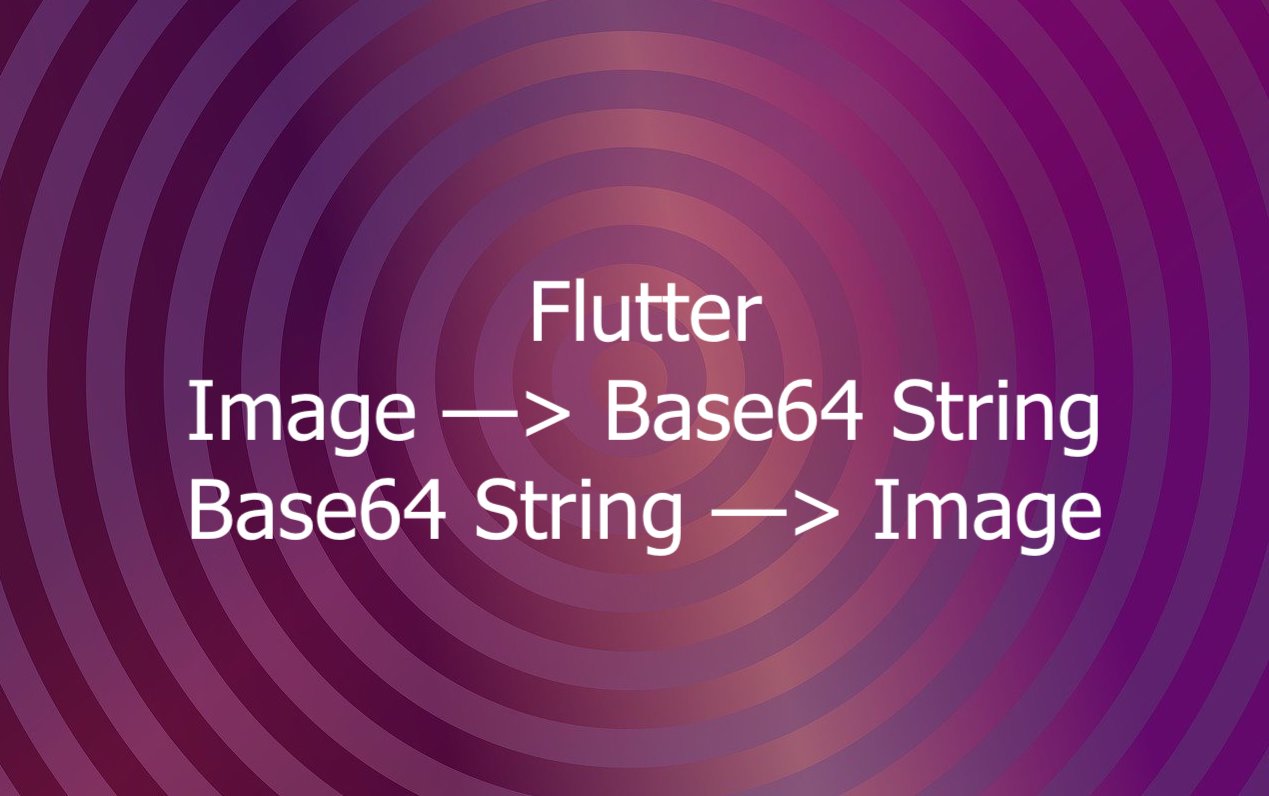
In Flutter, you can encode a local or network image (or another kind of file) to a base64 string like this:
import 'dart:io';
import 'dart:convert';
import 'dart:typed_data';
/* ...*/
//Create a file object from a local image file
String _imagePath = "/* Path to your image */";
File _imageFile = File(_imagePath);
// You can also create a file object from a URL like this:
// File _imageFile = File.fromUri('https://www.kindacode.com/non-existing-image.png');
// Read bytes from the file object
Uint8List _bytes = await _imageFile.readAsBytes();
// base64 encode the bytes
String _base64String = base64.encode(_bytes);
// Do something with the base64 string
You can decode a base64 string to an image file like this:
import 'dart:io';
import 'dart:convert';
import 'dart:typed_data';
/* ... */
final base64String = '/* your base64 string here */';
Uint8List _bytes = base64.decode(base64String);
File _myFile = File.fromRawPath(_bytes);
// Do something with the file object
In case you want to write a file to the device disk, do as follows:
import 'dart:io';
import 'dart:convert';
import 'dart:typed_data';
// Run "flutter pub add path_provider" to install the path_provider package
import 'package:path_provider/path_provider.dart';
/* ... */
final base64String = '/* your base64 string here */';
Uint8List _bytes = base64.decode(base64String);
final _directory = await getTemporaryDirectory();
File file = await File('${_directory.path}/my-image.jpg').create();
file.writeAsBytesSync(_bytes);
That’s the fundamentals. If you want to learn more details about file stuff in Flutter, take a look at the following articles:
- How to implement an image picker in Flutter
- Flutter: Reading Bytes from a Network Image
- Flutter: Rendering an Image from Byte Buffer/Int8List
- Base64 encoding and decoding in Dart (and Flutter)
- Flutter: Uploading Files with GetConnect (GetX)
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.