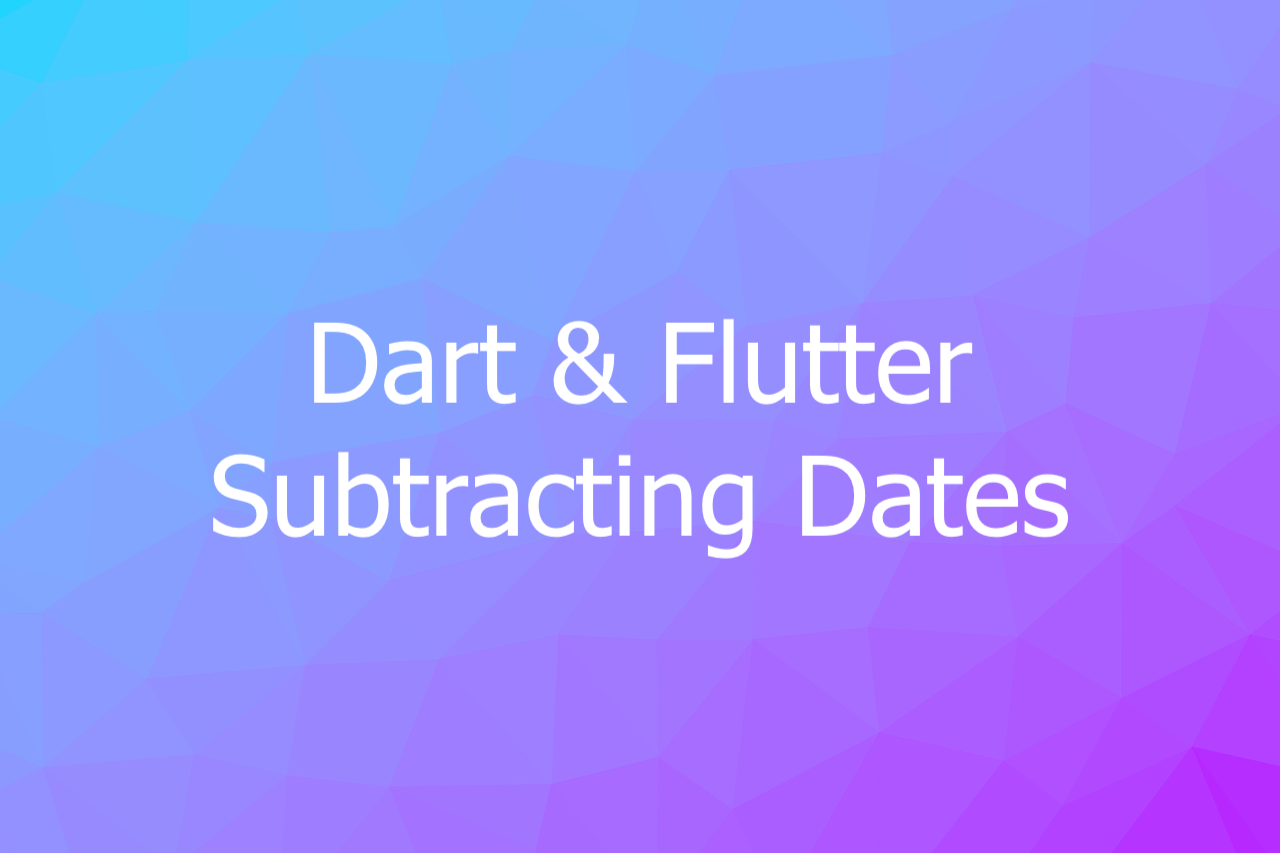
In Flutter and Dart, you can subtract two dates by using the DateTime.difference method. The result is a duration.
Example:
void main() {
final DateTime dateOne = DateTime(2022, 8, 30, 16, 59, 59);
final DateTime dateTwo = DateTime(1980, 4, 29, 13, 45, 45);
final Duration duration = dateOne.difference(dateTwo);
print("${duration.inHours} hours");
}
Output:
371115 hours
If you want to get rid of the blue line under the print() function, you can add a kDebugCheck like so:
import 'package:flutter/foundation.dart';
void main() {
final date = DateTime.now();
final result =
date.subtract(const Duration(days: 1001, hours: 10, minutes: 48));
if (kDebugMode) {
print(result);
}
}
The DateTime class also has a method named subtract. However, this one isn’t used for subtracting two dates but used for subtracting a duration from a given date. The result is a date.
Example:
void main() {
final date = DateTime.now();
final result =
date.subtract(const Duration(days: 1001, hours: 10, minutes: 48));
print(result);
}
Output:
2020-07-03 12:30:37.857438
Note: You’ll get a different result from mine because DateTime.now gives you a different date each time you execute the code.
Further reading:
- 4 Ways to Format DateTime in Flutter
- Dart & Flutter: Convert a Duration to HH:mm:ss format
- How to convert String to DateTime in Flutter and Dart
- Flutter: Giving a Size to a CircularProgressIndicator
- Flutter: Creating Transparent/Translucent App Bars
- Flutter & Hive Database: CRUD Example
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.