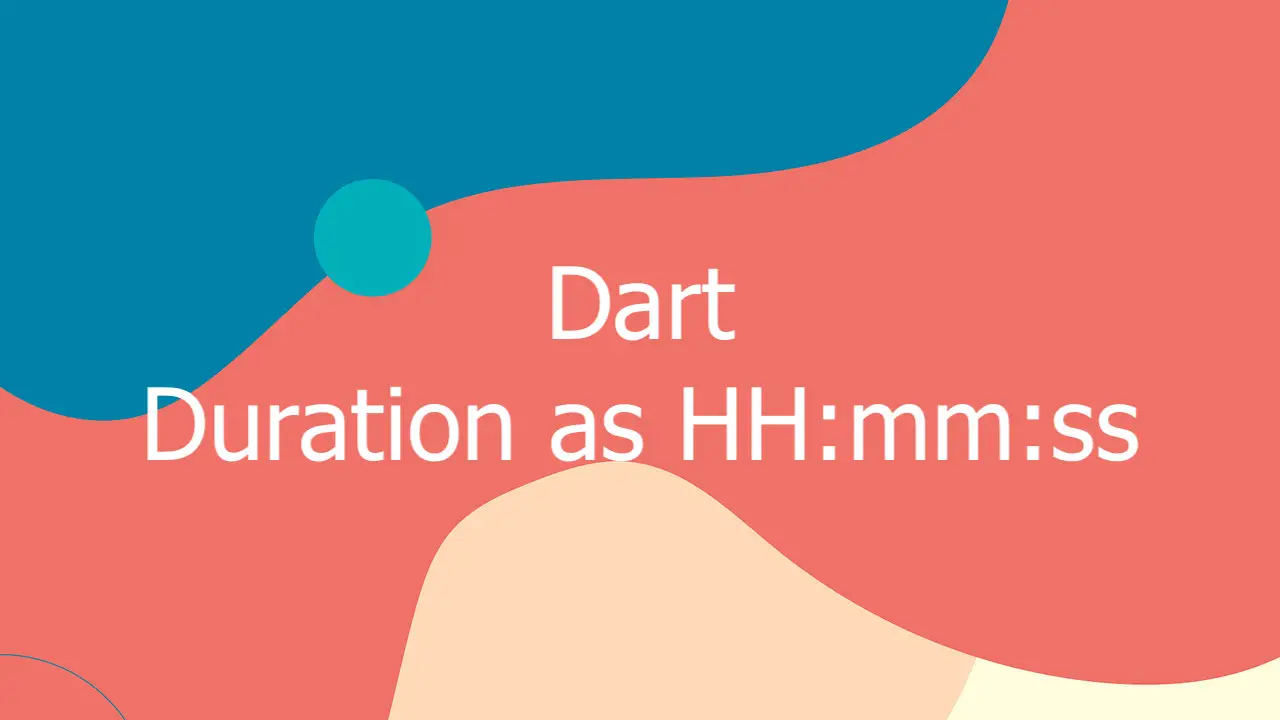
When working with Dart and Flutter, there might be cases where you want to turn a Duration into a string of HH:mm:ss format:
- HH: hours (If it is less than 10, there will be a preceding zero)
- mm: minutes as 2 digits from 00 to 59
- ss: seconds as 2 digits from 00 to 59
Though Dart doesn’t provide an out-of-the-box API that can help us get the job done, we can do it ourselves with only a few lines of code. Let’s create a reusable function called formatDuration as follows:
// Define the function
String formatDuration(Duration duration) {
String hours = duration.inHours.toString().padLeft(0, '2');
String minutes = duration.inMinutes.remainder(60).toString().padLeft(2, '0');
String seconds = duration.inSeconds.remainder(60).toString().padLeft(2, '0');
return "$hours:$minutes:$seconds";
}
And we can call it like this:
// Try it
void main() {
const durationOne = Duration(minutes: 900, seconds: 3);
const durationTwo = Duration(seconds: 19999);
const durationThree = Duration(days: 2, seconds: 1234);
print(formatDuration(durationOne));
print(formatDuration(durationTwo));
print(formatDuration(durationThree));
}
Output:
15:00:03
5:33:19
48:20:34
That’s it. Further reading:
- Flutter: Creating Transparent/Translucent App Bars
- Ways to Cancel a Future in Flutter and Dart
- Flutter & Dart: Convert a String into a List and vice versa
- Dart: Extract a substring from a given string (advanced)
- Inheritance in Dart: A Quick Example
- Text Overflow in Flutter: Tutorial & Examples
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.