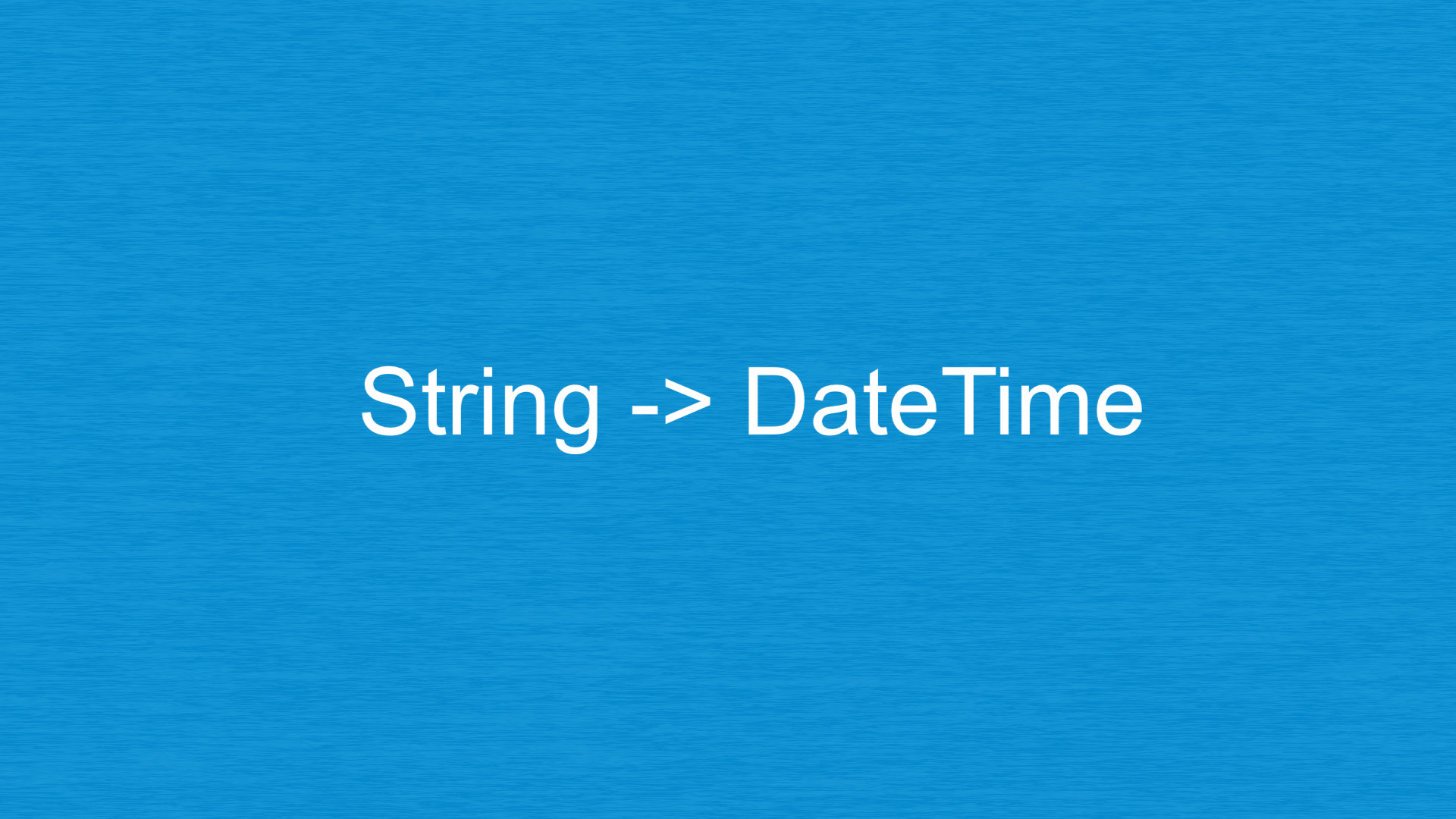
Table of Contents
Overview
The DateTime class of the dart:core library provides 2 methods that can help you convert a string to DateTime:
- DateTime.parse(String input): Constructs a new DateTime instance if the input is valid. Otherwise, throws a FormatException (Uncaught Error: FormatException: Invalid date format) when the input is not accepted.
- DateTime.tryParse(String input): Constructs a new DateTime instance if the input is valid. Otherwise, returns Null instead of throwing a FormatException when you pass an unaccepted string to it.
What Are Accepted Strings
Not all strings can be converted to DateTime. A common pitfall that developers may face is that many strings that look like a valid date are NOT accepted, such as:
18-11-20222
Nov, 20 2022
19/11/2022
12/30/2023
...
According to the Flutter official docs, the accepted inputs are:
- A date: A signed four-to-six-digit year, two-digit month, and two-digit day, optionally separated by – characters. Examples: “19700101”, “-0004-12-24”, and “81030-04-01”.
- An optional time part, separated from the date by either T or space. The time part is a two-digit hour, then optionally a two-digit minutes value, then optionally a two-digit seconds value, and then optionally a ‘.’ or ‘,’ followed by at least a one-digit second fraction. The minutes and seconds may be separated from the previous parts by a ‘:’. Examples: “12”, “12:30:24.124”, “12:30:24,124”, “123010.50”.
- An optional time-zone offset part, possibly separated from the previous by a space. The time zone is either ‘z’ or ‘Z’, or it is a signed two-digit hour part and an optional two-digit minute part. The sign must be either “+” or “-“, and can not be omitted. The minutes may be separated from the hours by a ‘:’. Examples: “Z”, “-10”, “+01:30”, and “+1130”.
Examples of accepted strings:
"2022-02-27"
"2022-02-27 13:27:00"
"2022-02-27 13:27:00.123456789z"
"2022-02-27 13:27:00,123456789z"
"20220227 13:27:00"
"20120227T132700"
"20220227"
"+20220227"
"2002-02-27T14Z"
"2002-02-27T14+00:00"
"-123450101 00:00:00 Z": in the year -12345.
"2002-02-27T14:00:00-0500": Same as "2002-02-27T19:00:00Z"
A Practical Example
The code:
void main() {
const String input1 = '2012-02-27 13:27:00.123456789z'; // this is valid
const String input2 = '2002-02-27T14+00:00'; // this is valid
const String input3 = "2022 Feb 12"; // This will not be accepted
final dt1 = DateTime.tryParse(input1);
print(dt1);
final dt2 = DateTime.tryParse(input2);
print(dt2);
final dt3 = DateTime.tryParse(input3);
print(dt3);
}
Output:
2012-02-27 13:27:00.123456Z
2002-02-27 14:00:00.000Z
null
Conclusion
You’ve learned how to turn a string (an appropriate one) into a DateTime instance. If you’d like to explore more new and exciting about modern Flutter development, take a look at the following articles:
- Flutter: Convert UTC Time to Local Time and Vice Versa
- Dart: Convert Timestamp to DateTime and vice versa
- 2 ways to convert DateTime to time ago in Flutter
- Flutter & Dart: 3 Ways to Generate Random Strings
- Flutter: Creating Custom Back Buttons
- Ways to Format DateTime in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.