
A confirm dialog is a dialog box that asks users to approve the requested operation. It usually appears with button pairs like Yes/No, OK/Cancel, Agree/Disagree. Confirm dialogs help prevent accidental actions such as deleting content, closing an account, leaving a page when the form content on that page has not been saved, etc.
This article shows you how to create confirm dialogs in Flutter. We will go over 2 examples: the first one demonstrates a material confirm dialog and the second one displays a Cupertino (iOS-style) confirm dialog.
Material Confirm Dialog
If you are developing an app for both Android and iOS and don’t need an iOS-exclusive experience, using material is a good way to go.
Preview
Our sample app has a button and a red box. When the button is pressed, a confirmation dialog will show up.
- If the user selects “Yes”, the box will be removed then the dialog will go away. The button then becomes disabled.
- If the user selects “No”, the dialog will be closed, and nothing else happens.
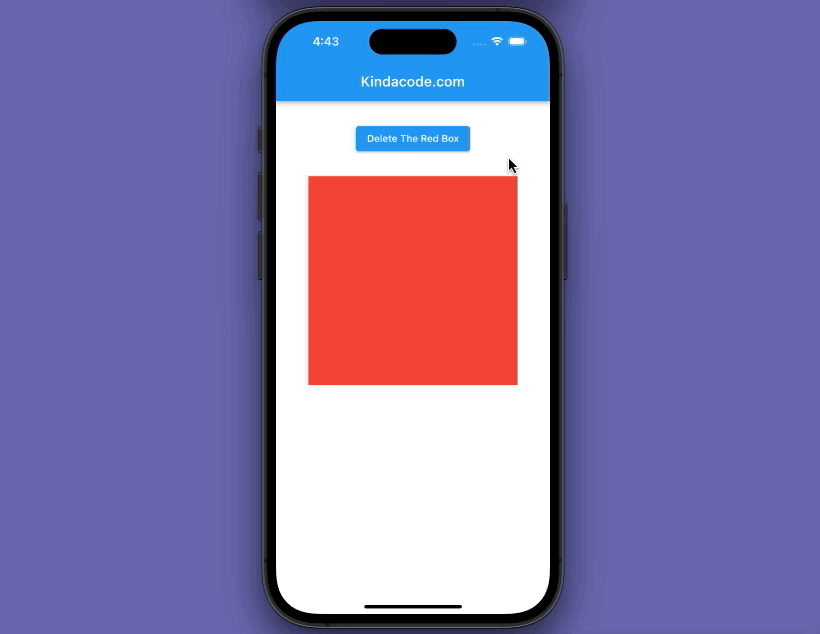
The code
The complete code with explanations:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// Determine whether the red box is shown or not
bool _isShown = true;
void _delete(BuildContext context) {
showDialog(
context: context,
builder: (BuildContext ctx) {
return AlertDialog(
title: const Text('Please Confirm'),
content: const Text('Are you sure to remove the box?'),
actions: [
// The "Yes" button
TextButton(
onPressed: () {
// Remove the box
setState(() {
_isShown = false;
});
// Close the dialog
Navigator.of(context).pop();
},
child: const Text('Yes')),
TextButton(
onPressed: () {
// Close the dialog
Navigator.of(context).pop();
},
child: const Text('No'))
],
);
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Column(
children: [
const SizedBox(
height: 30,
),
ElevatedButton(
// This button is enabled only if _isShown = true
onPressed: _isShown == true ? () => _delete(context) : null,
child: const Text('Delete The Red Box')),
const SizedBox(
height: 30,
),
if (_isShown == true)
Container(
width: 300,
height: 300,
color: Colors.red,
)
],
),
),
);
}
}
Cupertino (iOS-style) Confirm Dialog
Preview
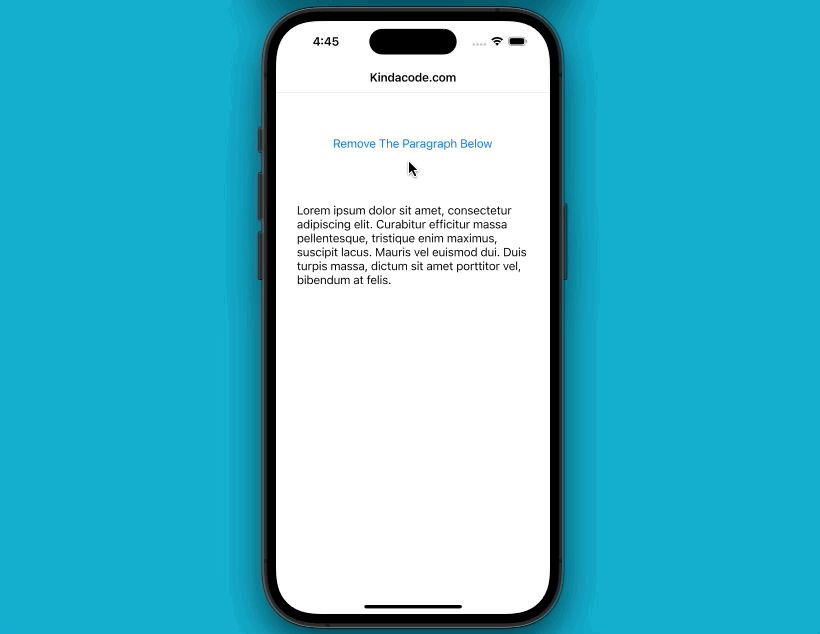
The code
Don’t forget to import the Cupertino library:
import 'package:flutter/cupertino.dart';
The full code for the example:
// main.dart
import 'package:flutter/cupertino.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const CupertinoApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// Determine whether the red box is shown or not
bool _isShown = true;
void _delete(BuildContext context) {
showCupertinoDialog(
context: context,
builder: (BuildContext ctx) {
return CupertinoAlertDialog(
title: const Text('Please Confirm'),
content: const Text('Are you sure to remove the text?'),
actions: [
// The "Yes" button
CupertinoDialogAction(
onPressed: () {
setState(() {
_isShown = false;
Navigator.of(context).pop();
});
},
isDefaultAction: true,
isDestructiveAction: true,
child: const Text('Yes'),
),
// The "No" button
CupertinoDialogAction(
onPressed: () {
Navigator.of(context).pop();
},
isDefaultAction: false,
isDestructiveAction: false,
child: const Text('No'),
)
],
);
});
}
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('Kindacode.com'),
),
child: Center(
child: Column(
children: [
const SizedBox(
height: 150,
),
CupertinoButton(
onPressed: _isShown == true ? () => _delete(context) : null,
child: const Text('Remove The Paragraph Below')),
const SizedBox(
height: 30,
),
if (_isShown == true)
const Padding(
padding: EdgeInsets.all(30),
child: Text(
'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Curabitur efficitur massa pellentesque, tristique enim maximus, suscipit lacus. Mauris vel euismod dui. Duis turpis massa, dictum sit amet porttitor vel, bibendum at felis. '),
)
],
),
));
}
}
Conclusion
This article walked you through more than one example of implementing confirm dialogs in Flutter applications. Continue learning more new and interesting stuff about mobile development by taking a look at the following articles:
- Using GetX (Get) for State Management in Flutter
- Most Popular Packages for State Management in Flutter
- Flutter CupertinoSegmentedControl example
- Working with Cupertino Bottom Tab Bar in Flutter
- Working with Time Picker in Flutter
You can also take a tour around our Flutter topic page or Dart topic page for the latest tutorials and examples.