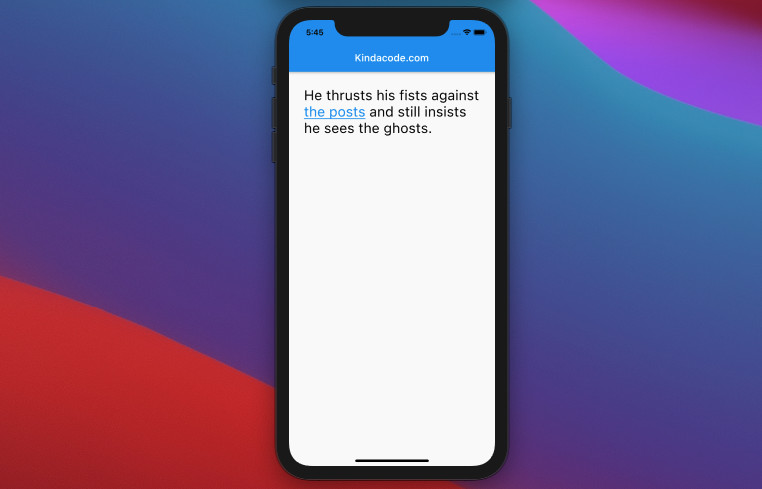
If you have worked a bit with HTML and web frontend then you should be not unfamiliar with hyperlinks, which are added with <a> tags and are clickable to perform some action like opening a URL, displaying a modal box, showing a hidden area, etc.
In Flutter, you can make text clickable (pressable or tappable as many mobile developers say) like hyperlinks by using some techniques shown and explained below.
Using GestureDetector widget
In this method, we will use a combination of different Text widgets and put them in a Wrap widget. For which Text widgets you want to be clickable, wrap them in GestureDetector widgets. We’ll also use a package named url_launcher to open a web URL.
Example Preview

The Code
The full source code in main.dart:
// main.dart
import 'package:flutter/material.dart';
import 'package:url_launcher/url_launcher_string.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: DefaultTextStyle(
style: const TextStyle(fontSize: 24, color: Colors.black87),
child: Wrap(
children: [
const Text('Welcome to '),
GestureDetector(
child: const Text(
'KindaCode.com',
style: TextStyle(
color: Colors.blue, decoration: TextDecoration.underline),
),
onTap: () async {
try {
await launchUrlString('https:/www.kindacode.com');
} catch (err) {
debugPrint('Something bad happened');
}
},
),
const Text(' - a website about programming.')
],
),
),
),
);
}
}
Using TextSpan recognizer
We will use a RichText widget with multiple TextSpan widgets. Some TextSpan widgets are used to present clickable text, and others are used to display normal text. Note that the TextSpan widget has a property named recognizer and we can add a gesture recognizer there to make it clickable. In order to do so, we need to import this:
import 'package:flutter/gestures.dart';
See the example below for more clarity.
Example Preview
This sample app contains a paragraph with clickable text. When the clickable text is clicked (or tapped), a dialog will show up. This action can be replaced with behavior like launching a web URL.
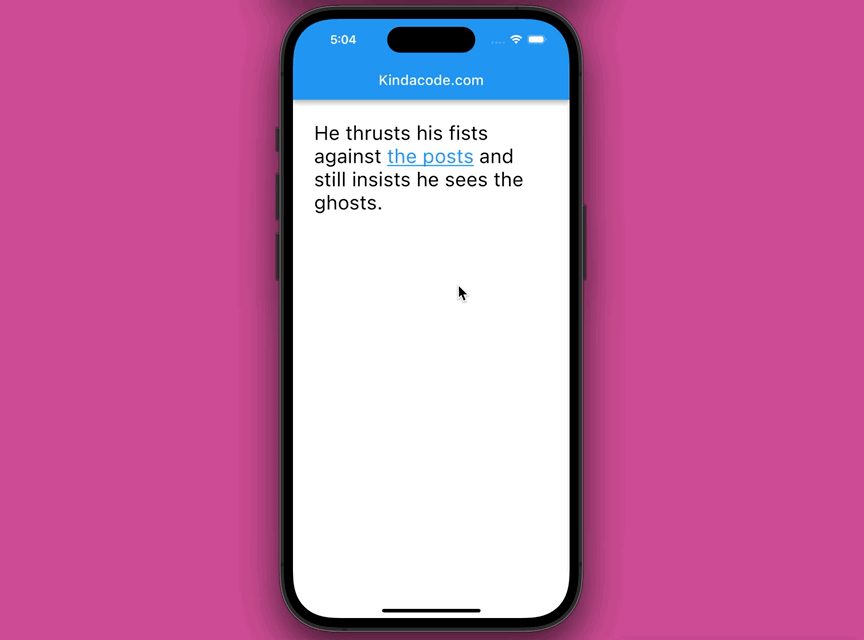
The Code
Here’s the complete code in main.dart:
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/gestures.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: RichText(
text: TextSpan(
text: 'He thrusts his fists against ',
style: const TextStyle(fontSize: 28, color: Colors.black),
children: [
TextSpan(
text: 'the posts',
recognizer: TapGestureRecognizer()
..onTap = () => showDialog(
context: context,
builder: (BuildContext _) {
return const AlertDialog(
title: Text('Alert Dialog'),
content: Text('Hi there. Have a nice day!'),
);
}),
style: const TextStyle(
color: Colors.blue,
decoration: TextDecoration.underline,
)),
const TextSpan(text: ' and still insists he sees the ghosts.'),
],
),
),
),
);
}
}
Another Solution
You can also show hyperlinks interwoven with text by rendering HTML content in your Flutter apps. This method is detailedly described in this article: How to render HTML content in Flutter.
Conclusion
We’ve walked through some techniques to make text clickable like hyperlinks. The scenarios you will need them aren’t rare when building web apps or mobile apps with Flutter, and you’re totally free to choose which approach you like to get your tasks done.
Flutter’s awesome, and there’re many things to learn about it. If you want to explore more interesting stuff about it, take a look at the following articles:
- Flutter and Firestore Database: CRUD example
- Working with ElevatedButton in Flutter
- How to make Circular Buttons in Flutter
- Flutter: Make a simple Color Picker from scratch
- Flutter Cupertino Button – Tutorial and Examples
- How to use Cupertino icons in Flutter
- Flutter CupertinoSegmentedControl example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.