This article shows you how to use Cupertino icons (iOS-style icons) in a Flutter application using the CupertinoIcons class.
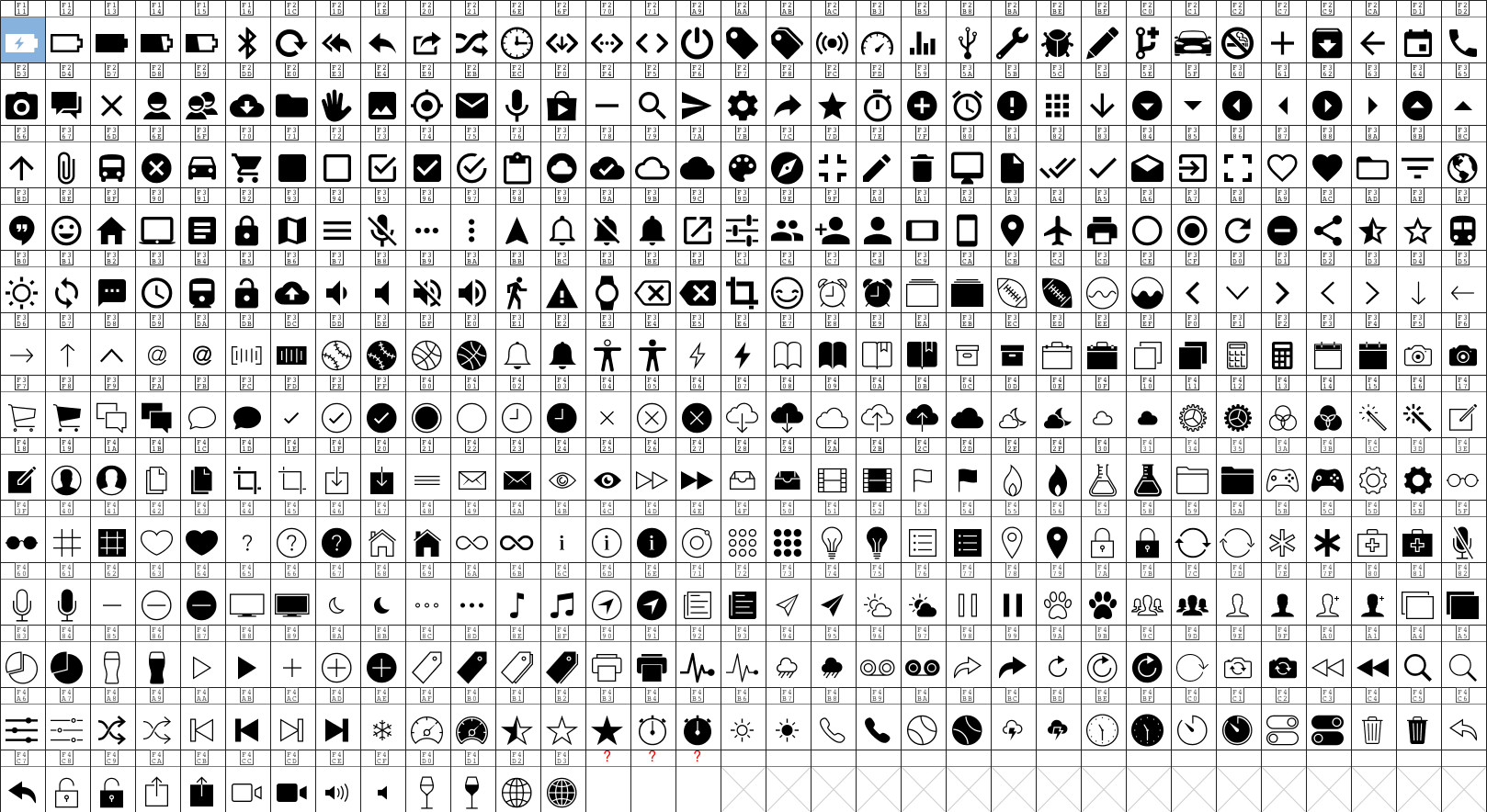
Table of Contents
How to use Cupertino icons?
When you create a new Flutter project, cupertino_icons is added to the dependencies section of the pubspec.yaml file by default, like this:
dependencies:
flutter:
sdk: flutter
# The following adds the Cupertino Icons font to your application.
# Use with the CupertinoIcons class for iOS style icons.
cupertino_icons: ^1.0.2
Make sure you don’t remove it.
1. Import the Cupertino package into your Dart code:
import 'package:flutter/cupertino.dart';
2. Usage:
Icon(CupertinoIcons.<icon-name>,)
You can set the size and the color for a Cupertino icon through the size and color properties, like this:
Icon(
CupertinoIcons.heart_fill,
color: Color.fromARGB(255, 255, 15, 15),
size: 50,
),
See the full list of available Cupertino icons here.
Example
Screenshot:
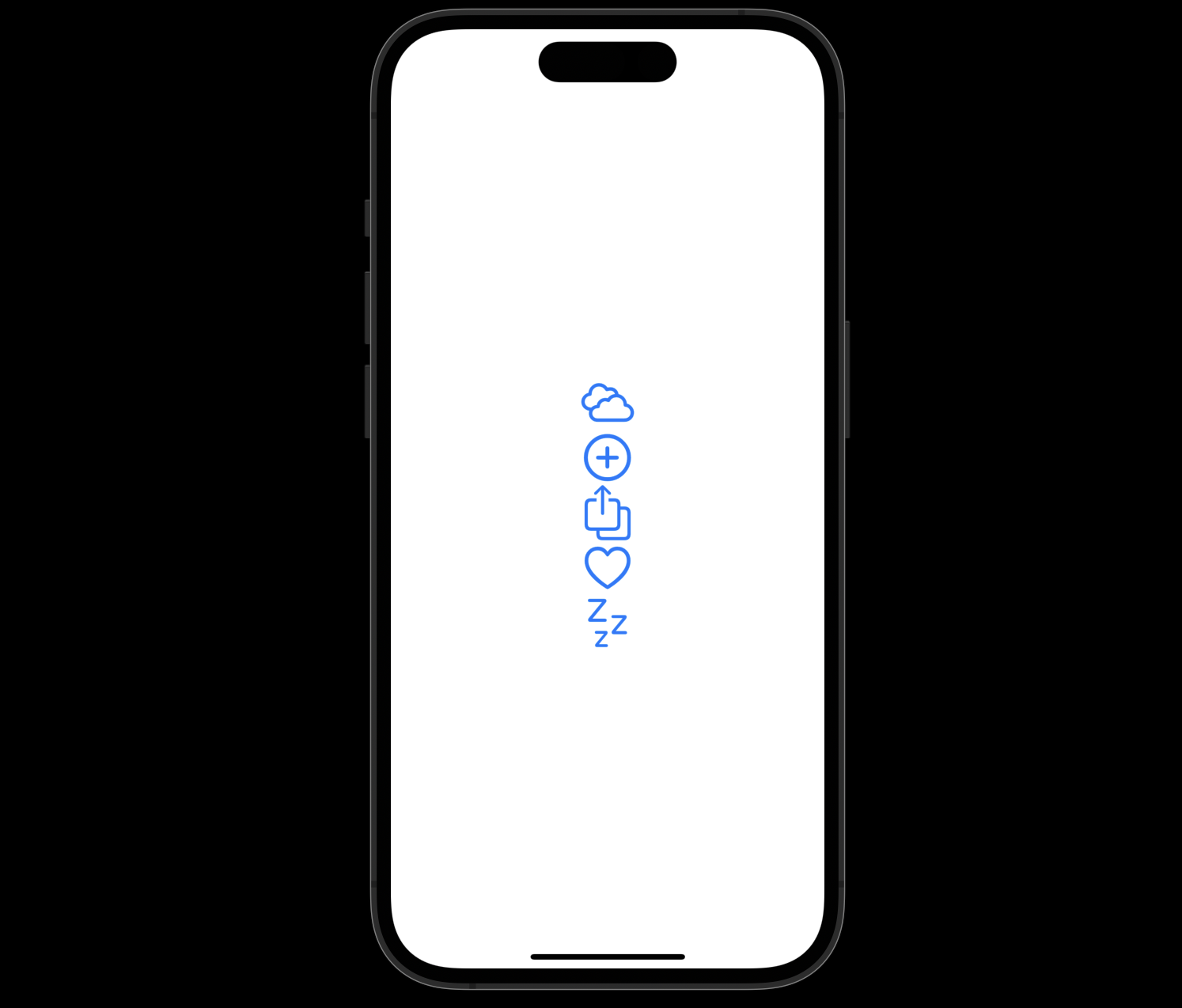
The full code:
// KindaCode.com
import 'package:flutter/cupertino.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const CupertinoApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const CupertinoPageScaffold(
child: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon(
CupertinoIcons.smoke,
size: 50,
),
Icon(
CupertinoIcons.add_circled,
size: 50,
),
Icon(CupertinoIcons.square_arrow_up_on_square, size: 50),
Icon(
CupertinoIcons.suit_heart,
size: 50,
),
Icon(
CupertinoIcons.zzz,
size: 50,
)
],
),
),
),
);
}
}
What’s Next?
We’ve gone over an example of implementing Cupertino icons in Flutter. If you’d like to explore more iOS-style things in Flutter, take a look at the following articles:
- Flutter Cupertino Button – Tutorial and Examples
- Flutter CupertinoSegmentedControl Example
- Example of CupertinoSliverNavigationBar in Flutter
- Working with Cupertino Bottom Tab Bar in Flutter
- Working with Cupertino Date Picker in Flutter
- Flutter CupertinoAlertDialog Example
You can also take a tour around our Flutter topic page or Dart topic page for the latest tutorials and examples.