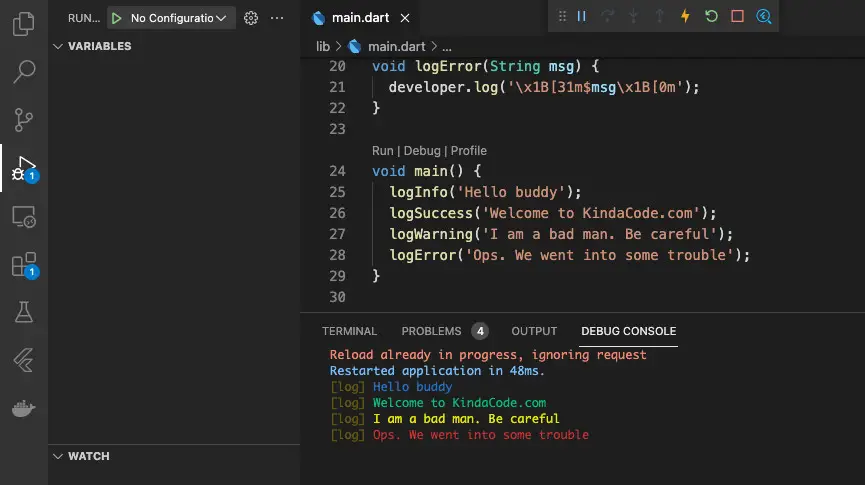
Introduction
Logging messages in different colors makes fixing bugs and developing apps with Flutter more fun and enjoyable. This article will show you how to do that without using any third-party plugins.
Overview
To output colored logs in the debug console without 3rd packages, we need to use ANSI escape codes and the log() function from the built-in dart:developer module.
Some commonly used ANSI escape codes:
Reset: \x1B[0m
Black: \x1B[30m
White: \x1B[37m
Red: \x1B[31m
Green: \x1B[32m
Yellow: \x1B[33m
Blue: \x1B[34m
Cyan: \x1B[36m
Logging text with custom colors to the debug console can be done like this:
import 'dart:developer' as developer;
/*...*/
developer.log('[ANSI color code][your text][ANSI reset code]');
You can wonder why we don’t use the print() function. The reason here is that ANSI color codes are ignored when using it (at least it doesn’t work with VS Code).
You can find more information about the ANSI escape code in this article on Wikipedia.
Example
The code:
// main.dart
import 'dart:developer' as developer;
// Blue text
void logInfo(String msg) {
developer.log('\x1B[34m$msg\x1B[0m');
}
// Green text
void logSuccess(String msg) {
developer.log('\x1B[32m$msg\x1B[0m');
}
// Yellow text
void logWarning(String msg) {
developer.log('\x1B[33m$msg\x1B[0m');
}
// Red text
void logError(String msg) {
developer.log('\x1B[31m$msg\x1B[0m');
}
void main() {
logInfo('Hello buddy');
logSuccess('Welcome to KindaCode.com');
logWarning('I am a bad man. Be careful');
logError('Ops. We ran into some trouble');
}
Start debugging by hitting the debug button on the top right corner of your VS Code window:
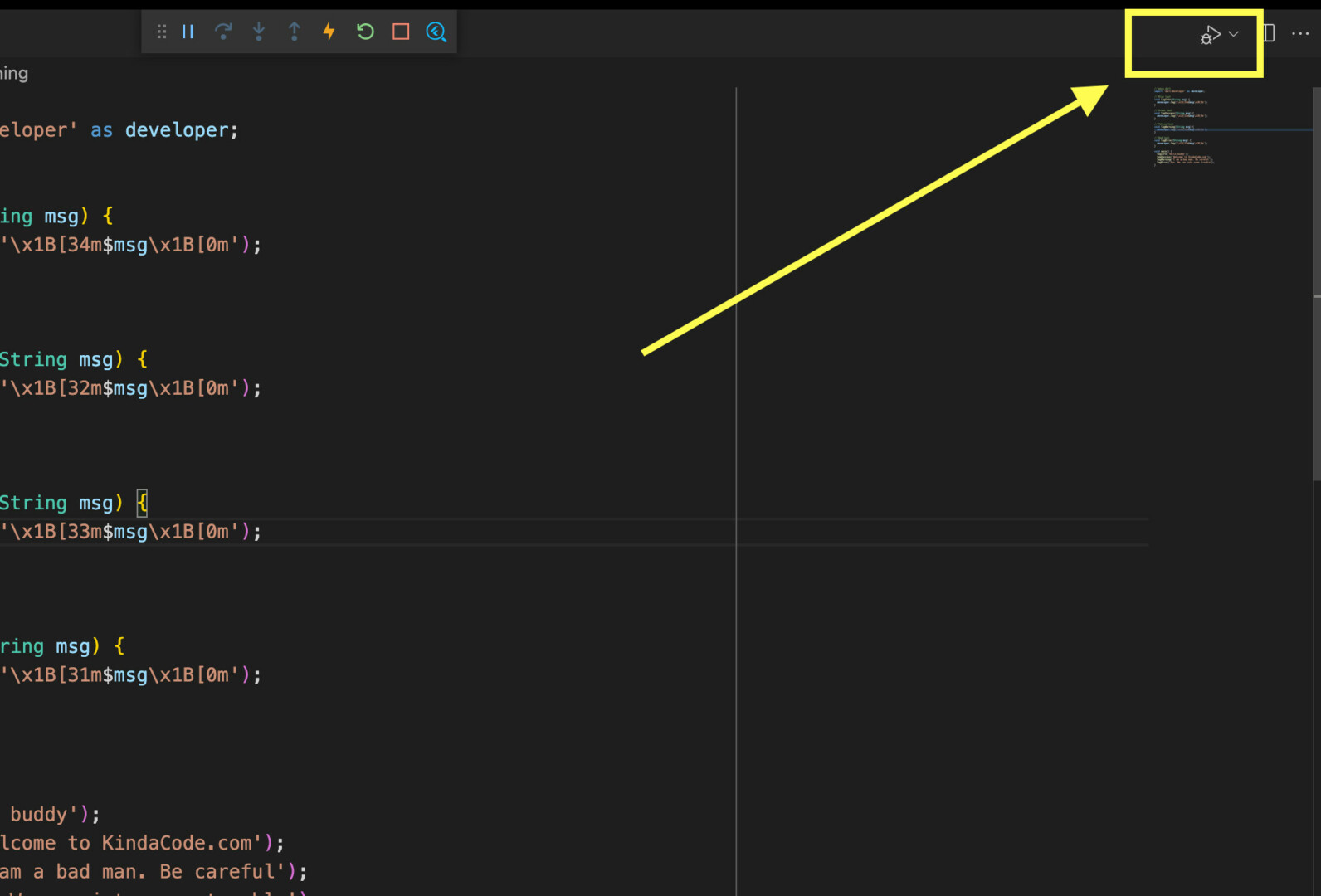
The result in the DEBUG CONSOLE tab (not the TERMINAL tab):

Conclusion
You’ve learned how to colorize the text output in the Debug Console. If you’d like to explore more new and interesting stuff in Flutter, take a look at the following articles:
- Flutter: 2 Ways to Compare 2 Deep Nested Maps
- Flutter: Caching Network Images for Big Performance gains
- Flutter: Add a Search Field to an App Bar (2 Approaches)
- Flutter & SQLite: CRUD Example
- Flutter StreamBuilder examples (null safety)
- Flutter AnimatedList – Tutorial and Examples
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.
Thanks a lot 🥰🎉