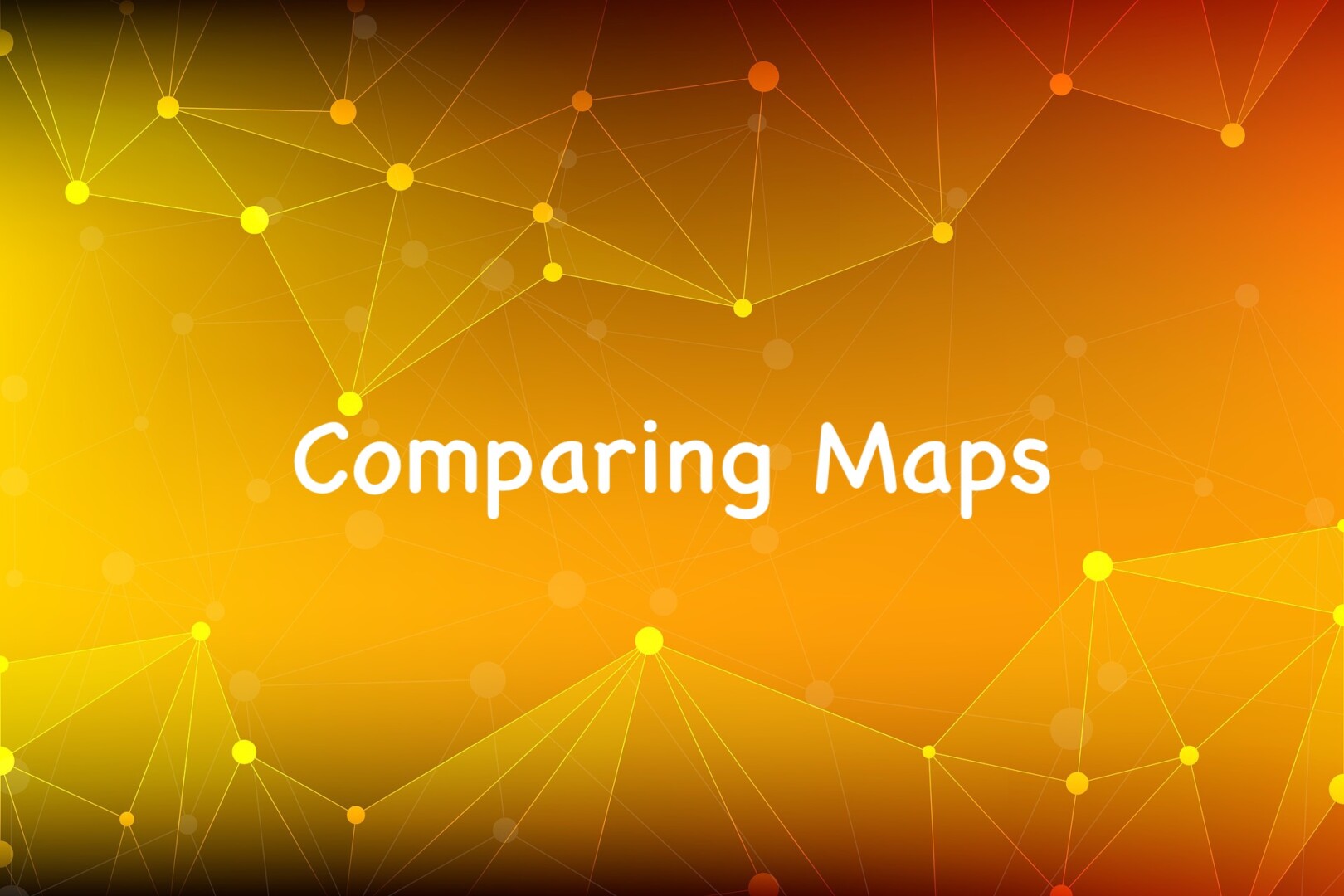
In most programming languages, 2 maps are considered equal if they have the same length, and contain the same keys associated with the same values. This article shows you 2 different ways to correctly compare 2 deep nested maps in Flutter. Without any further ado, let’s examine the code.
Using equals() method (DeepCollectionEquality class)
The equals method of the DeepCollectionEquality class can help us compare 2 nested maps with a few lines of code. To use it, you need to import the collection library:
import 'package:collection/collection.dart';
Example
Let’s say we have 3 maps: item1, item2, and item3. Our job is to compare the first item with the second and the third one.
// kindacode.com example
import 'package:collection/collection.dart';
// Create dummy maps for testing
// map 1
Map<String, dynamic> item1 = {
'name': 'T-shirt',
'color': 'blue',
'price': 10.99,
'details': {
'department': 'men',
'manufactuer': 'KindaCode.com',
'style': 'classic',
'size': ['S', 'M', 'L', 'XL', '2XL', '3XL'],
'material': 'cotton',
'ship': ['Standart', 'FedEx Air']
}
};
// map 2
Map<String, dynamic> item2 = {
'name': 'T-shirt',
'color': 'blue',
'price': 10.99,
'details': {
'department': 'men',
'manufactuer': 'KindaCode.com',
'style': 'classic',
'size': ['S', 'M', 'L', 'XL', '2XL', '3XL'],
'material': 'cotton',
'ship': ['Standart', 'FedEx Air']
}
};
// map 3
Map<String, dynamic> item3 = {
'name': 'T-shirt',
'color': 'blue',
'price': 10.99,
'details': {
'department': 'mens',
'manufactuer': 'KindaCode.com',
'style': 'classic',
'size': ['S', 'M', 'L', 'XL'],
'material': 'cotton',
'ship': ['Standart', 'FedEx Air']
}
};
// compare the maps
void main() {
// Comparing item #1 vs item #2
if (const DeepCollectionEquality().equals(item1, item2)) {
print('Item #1 and item #2 are equal');
} else {
print('Item #1 and item #2 are not equal');
}
// Comparing item #1 vs item #3
if (const DeepCollectionEquality().equals(item1, item3)) {
print('Item #1 and item #3 are equal');
} else {
print('Item #1 and item #3 are not equal');
}
}
Output:
Item #1 and item #2 are equal
Item #1 and item #3 are not equal
If you look closer, item 3 doesn’t go with 2XL size. That’s why it is different from the others.
Converting Maps to JSON
The strategy here is to convert each map to a JSON string and then compare the returned strings.
Example
We’ll use the same maps as the first example.
// kindacode.com example
import 'dart:convert';
Map<String, dynamic> item1 = {
'name': 'T-shirt',
'color': 'blue',
'price': 10.99,
'details': {
'department': 'men',
'manufactuer': 'KindaCode.com',
'style': 'classic',
'size': ['S', 'M', 'L', 'XL', '2XL', '3XL'],
'material': 'cotton',
'ship': ['Standart', 'FedEx Air']
}
};
Map<String, dynamic> item2 = {
'name': 'T-shirt',
'color': 'blue',
'price': 10.99,
'details': {
'department': 'men',
'manufactuer': 'KindaCode.com',
'style': 'classic',
'size': ['S', 'M', 'L', 'XL', '2XL', '3XL'],
'material': 'cotton',
'ship': ['Standart', 'FedEx Air']
}
};
Map<String, dynamic> item3 = {
'name': 'T-shirt',
'color': 'blue',
'price': 10.99,
'details': {
'department': 'mens',
'manufactuer': 'KindaCode.com',
'style': 'classic',
'size': ['S', 'M', 'L', 'XL'],
'material': 'cotton',
'ship': ['Standart', 'FedEx Air']
}
};
void main() {
// Converting maps to strings
String s1 = json.encode(item1);
String s2 = json.encode(item2);
String s3 = json.encode(item3);
// Comparing item #1 vs item #2
if (s1 == s2) {
print('Item #1 and item #2 are equal');
} else {
print('Item #1 and item #2 are not equal');
}
// Comparing item #1 vs item #3
if (s1 == s3) {
print('Item #1 and item #3 are equal');
} else {
print('Item #1 and item #3 are not equal');
}
}
Output:
Item #1 and item #2 are equal
Item #1 and item #3 are not equal
What’s Next?
We’ve gone through a few techniques to deep compare two different maps in Flutter. These things may be very useful for you in certain situations in the future when you work with massive data apps.
Flutter is awesome and there are so many great features to explore. Keep yourself moving and continue learning by taking a look at the following articles:
- Dart: Calculate the Sum of all Values in a Map
- Dart: Sorting Entries of a Map by Its Values
- Flutter & SQLite: CRUD Example
- Flutter: ValueListenableBuilder Example
- Flutter StreamBuilder examples (null safety)
- 4 Ways to Store Data Offline in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.