
In Flutter, the CupertinoTimerPicker
widget is used to display an iOS-style countdown timer picker. It shows a countdown duration with hour, minute, and second spinners. The duration is bound between 0 and 23 hours 59 minutes 59 seconds.
In this code-centric article, we’ll examine a complete example that demonstrates how to implement CupertinoTimerPicker
by using the showCupertinoModalPopup()
function, and then later learn the fundamentals of the widget.
The Example
App Preview
The small app we’re going to make has a Text
widget and a Cupertino button in the center of the screen. When this button gets pressed, a bottom modal will show up. This modal contains a CupertinoTimerPicker
, a Cancel button, and a Done button. If the Cancel button is hit, no duration is selected and the modal will be closed. If the Done button is hit, the selected duration will be saved, and the modal will go away afterward.
Here’s how it works:
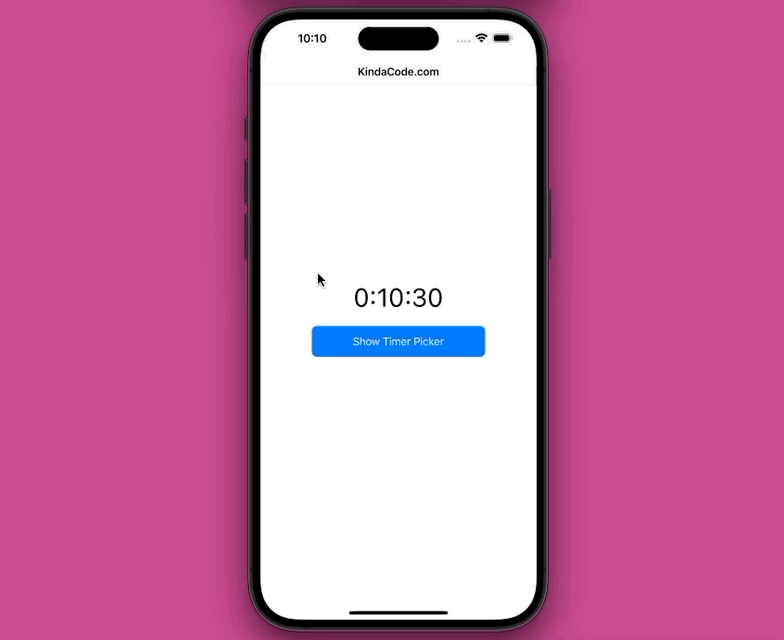
The Code
Here’s the full source code in main.dart
with explanations:
import 'package:flutter/cupertino.dart';
void main() => runApp(const TimerPickerApp());
class TimerPickerApp extends StatelessWidget {
const TimerPickerApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const CupertinoApp(
debugShowCheckedModeBanner: false,
theme: CupertinoThemeData(brightness: Brightness.light),
title: "KindaCode.com",
home: KindaCodeDemo(),
);
}
}
class KindaCodeDemo extends StatefulWidget {
const KindaCodeDemo({Key? key}) : super(key: key);
@override
State<KindaCodeDemo> createState() => _KindaCodeDemoState();
}
class _KindaCodeDemoState extends State<KindaCodeDemo> {
// The duration the user has selected.
// In the beginning, it is set to 10 minutes and 30 seconds.
Duration duration = const Duration(minutes: 10, seconds: 30);
// This function is triggered when the "show timer picker" button is pressed.
void _showDialog() {
showCupertinoModalPopup(
context: context,
builder: (BuildContext context) => Container(
height: 300,
padding: const EdgeInsets.only(top: 6.0),
margin: EdgeInsets.only(
bottom: MediaQuery.of(context).viewInsets.bottom,
),
color: CupertinoColors.systemBackground.resolveFrom(context),
child: SafeArea(
top: false,
child: Column(
children: [
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
// Close the modal
CupertinoButton(
child: const Text('Cancel'),
onPressed: () => Navigator.pop(context),
),
// Save the duration and close the modal
CupertinoButton(
child: const Text('Done'),
onPressed: () {
Navigator.pop(context);
setState(() {});
},
),
],
),
// The timer picker widget
Expanded(
child: CupertinoTimerPicker(
mode: CupertinoTimerPickerMode.hms,
initialTimerDuration: duration,
secondInterval: 5,
// This is called when the user changes the timer's
// duration.
onTimerDurationChanged: (Duration newDuration) {
duration = newDuration;
},
),
),
],
),
),
),
);
}
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('KindaCode.com'),
),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
duration.toString().split('.').first,
style: const TextStyle(fontSize: 40),
),
const SizedBox(height: 20),
CupertinoButton.filled(
onPressed: _showDialog,
child: const Text('Show Timer Picker'),
),
],
),
),
);
}
}
API
Below’s the constructor of the CupertinoTimerPicker
widget:
CupertinoTimerPicker({
Key? key,
CupertinoTimerPickerMode mode = CupertinoTimerPickerMode.hms,
Duration initialTimerDuration = Duration.zero,
int minuteInterval = 1,
int secondInterval = 1,
AlignmentGeometry alignment = Alignment.center,
Color? backgroundColor,
double itemExtent = _kItemExtent,
required ValueChanged<Duration> onTimerDurationChanged
})
There are so many parameters. Let me explain them one by one:
key
: An optional key for the widget.mode
: An optional parameter that specifies the mode of the timer picker. It can be one of the following values:CupertinoTimerPickerMode.hms
(default),CupertinoTimerPickerMode.hm
,CupertinoTimerPickerMode.ms
, orCupertinoTimerPickerMode.s
.initialTimerDuration
: An optional parameter that specifies the initial duration of the timer. It defaults to zero.minuteInterval
: An optional parameter that specifies the granularity of the minute spinner. It must be a positive integer that divides 60 evenly. It defaults to 1.secondInterval
: An optional parameter that specifies the granularity of the second spinner. It must be a positive integer that divides 60 evenly. It defaults to 1.alignment
: An optional parameter that specifies how the timer picker should be positioned within its available space. It defaults to the center.backgroundColor
: An optional parameter that specifies the background color of the timer picker. It defaults to white.onTimerDurationChanged
: A required callback function that is called when the user changes the timer’s duration. It takes a Duration parameter as the new duration.
Final Words
CupertinoTimerPicker
is a useful and easy-to-use widget that allows users to select a countdown duration in an iOS-style interface. It has several modes and options to customize its appearance and functionality. It can be used for various purposes, such as setting timers, alarms, reminders, etc.
If you’d like to explore more built-in iOS-style widgets in Flutter, take a look at the following articles:
- Working with Cupertino Date Picker in Flutter
- Flutter Cupertino Button – Tutorial and Examples
- Flutter CupertinoSegmentedControl Example
- Flutter CupertinoSliverNavigationBar: Tutorial & Example
- Flutter CupertinoSlider Example
- Working with Cupertino Bottom Tab Bar in Flutter
- Flutter Cupertino ActionSheet: Tutorial & Example
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.