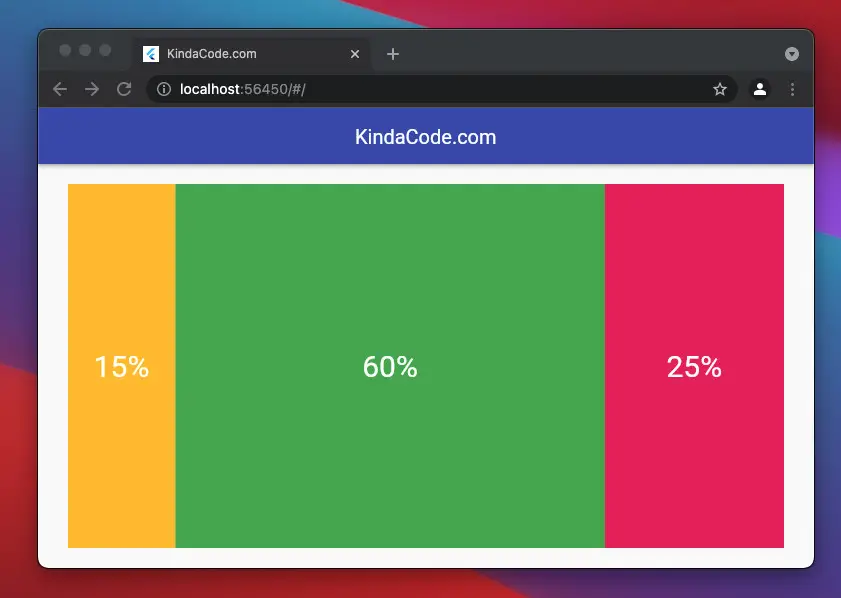
This article walks you through a couple of examples of implementing layouts with multiple columns whose widths aren’t fixed but in percentages.
Example 1: Using Flexible or Expanded widget
This example creates a layout with 3 columns whose widths are 15%, 60%, and 25%, respectively.
Preview (on Chrome):
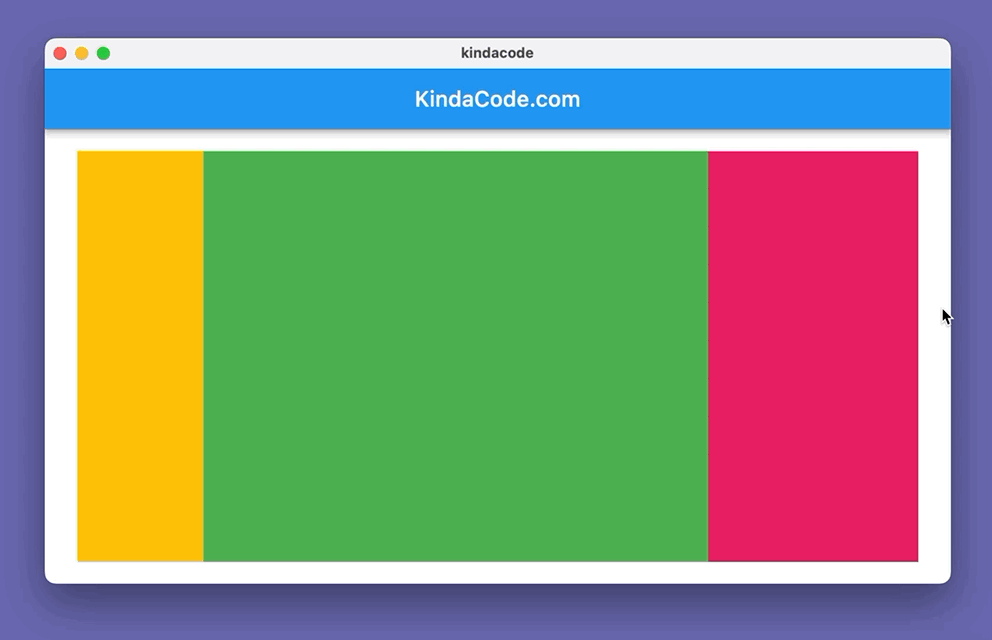
The code:
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Padding(
padding: const EdgeInsets.symmetric(vertical: 20, horizontal: 30),
child: Row(
mainAxisSize: MainAxisSize.max,
children: [
Flexible(
flex: 15, // 15%
child: Container(
color: Colors.amber,
alignment: Alignment.center,
child: Column(),
)),
Flexible(
flex: 60, // 60%
child: Container(
color: Colors.green,
alignment: Alignment.center,
child: Column(),
)),
Flexible(
flex: 25, // 25%
child: Container(
color: Colors.pink,
alignment: Alignment.center,
child: Column(),
))
],
),
),
);
You can produce the same result by replacing the Flexible widget with the Expanded widget.
Example 2: Using MediaQuery
This example creates a layout that consists of 3 columns with widths are 30%, 50%, and 20%, respectively.
Screenshot:
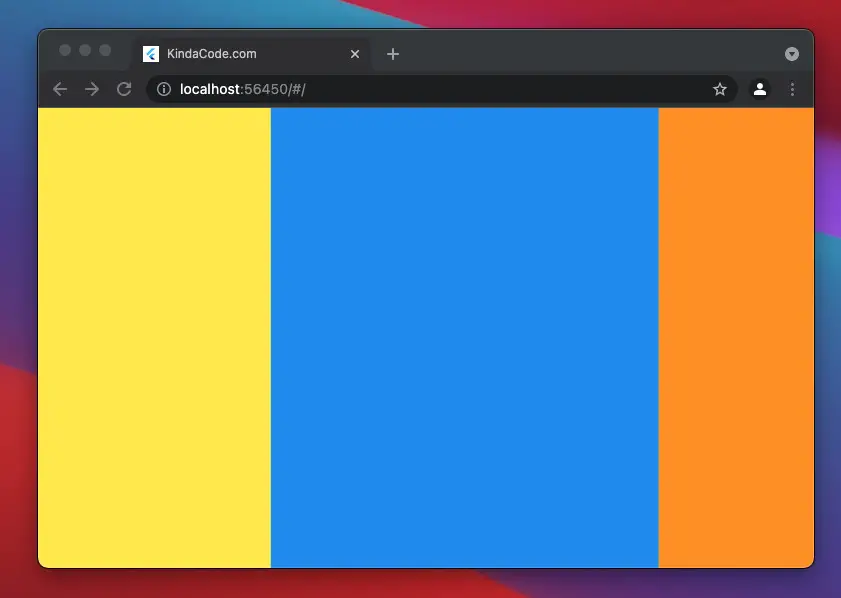
The code:
Scaffold(
body: Row(
mainAxisSize: MainAxisSize.max,
children: [
Container(
width: MediaQuery.of(context).size.width * 0.3,
color: Colors.yellow,
alignment: Alignment.center,
child: Column(),
),
Container(
width: MediaQuery.of(context).size.width * 0.5,
color: Colors.blue,
alignment: Alignment.center,
child: Column(),
),
Container(
width: MediaQuery.of(context).size.width * 0.2,
color: Colors.orange,
alignment: Alignment.center,
child: Column(),
)
],
),
);
One More Solution
Flutter is awesome and we often have more than one solution to archive the same thing. Besides using Flexible/Expanded and MediaQuery, you can build a layout with percentage width columns by using the LayoutBuilder widget. You can see the details and practical examples of LayoutBuilder in this article.
Wrapping Up
You’ve learned a few techniques to implement Columns with percentage widths. If you’d like to explore more interesting and useful things in Flutter, take a look at the following articles:
- Flutter & Hive Database: CRUD Example
- Flutter & SQLite: CRUD Example
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Flutter: ListTile examples
- Flutter SliverList – Tutorial and Example
- Flutter: Making a Dropdown Multiselect with Checkboxes
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.