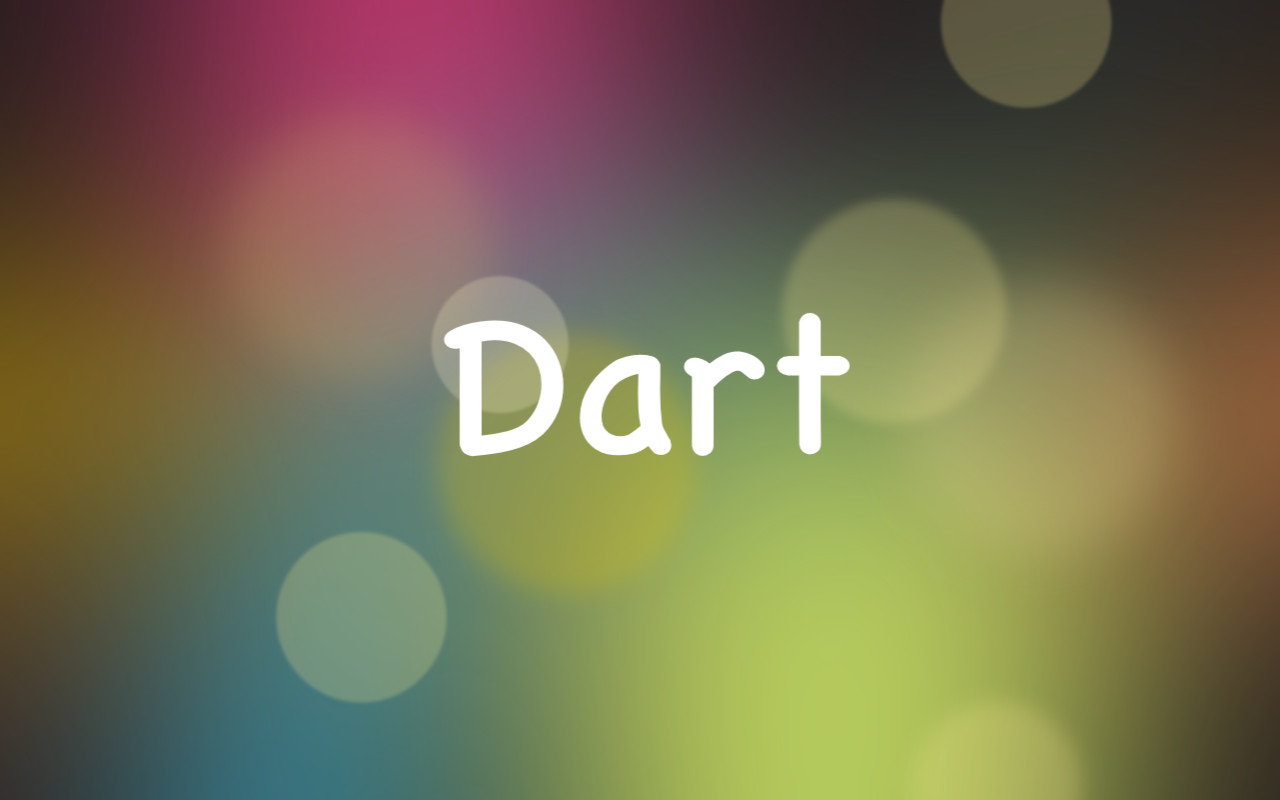
This concise and straightforward article shows you two different ways to create multi-line strings in Dart (and Flutter as well).
Table of Contents
Using Triple Quotes
Both triple-single-quote (”’) and triple-double-quote (“””) work fine.
Example:
import 'package:flutter/material.dart';
void main() {
String s1 = '''
This is a
multi-line
string
''';
debugPrint(s1);
String s2 = """
Lorem ipsum dolor sit amet,
consectetur 'adipiscing elit'.
Vivamus id sapien nec felis pulvinar feugiat.
""";
debugPrint(s2);
}
Output:
This is a
multi-line
string
Lorem ipsum dolor sit amet,
consectetur 'adipiscing elit'.
Vivamus id sapien nec felis pulvinar feugiat.
Note that anything between the starting and ending quotes becomes part of the string so this example has a leading blank. The strings will also contain both blanks and newlines.
Using Adjacent String Literals
This approach doesn’t add any extra blanks or newlines. If you want some line breaks, you need to add “\n”.
Example:
import 'package:flutter/material.dart';
void main() {
String s1 = 'He thrusts his fists'
'against the posts'
'and still insists'
'he sees the ghosts.';
debugPrint(s1);
debugPrint('-------'); // Just a horizontal line
String s2 = 'He thrusts his fists\n'
'against the posts\n'
'and still insists\n'
'he sees the ghosts.';
debugPrint(s2);
}
Output:
He thrusts his fistsagainst the postsand still insistshe sees the ghosts.
-------
He thrusts his fists
against the posts
and still insists
he sees the ghosts.
Conclusion
You’ve learned how to make multi-line strings in Dart through some examples. Continue exploring more interesting stuff about Dart, an awesome programming language for app development by taking a look at the following articles:
- Sorting Lists in Dart and Flutter (5 Examples)
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- Flutter & Dart: Get File Name and Extension from Path/URL
- Understanding Typedefs (Type Aliases) in Dart and Flutter
- Flutter & Dart: Regular Expression Examples
- Flutter & Dart: Get a list of dates between 2 given dates
You can also take a tour around our Flutter topic page or Dart topic page for the latest tutorials and examples.