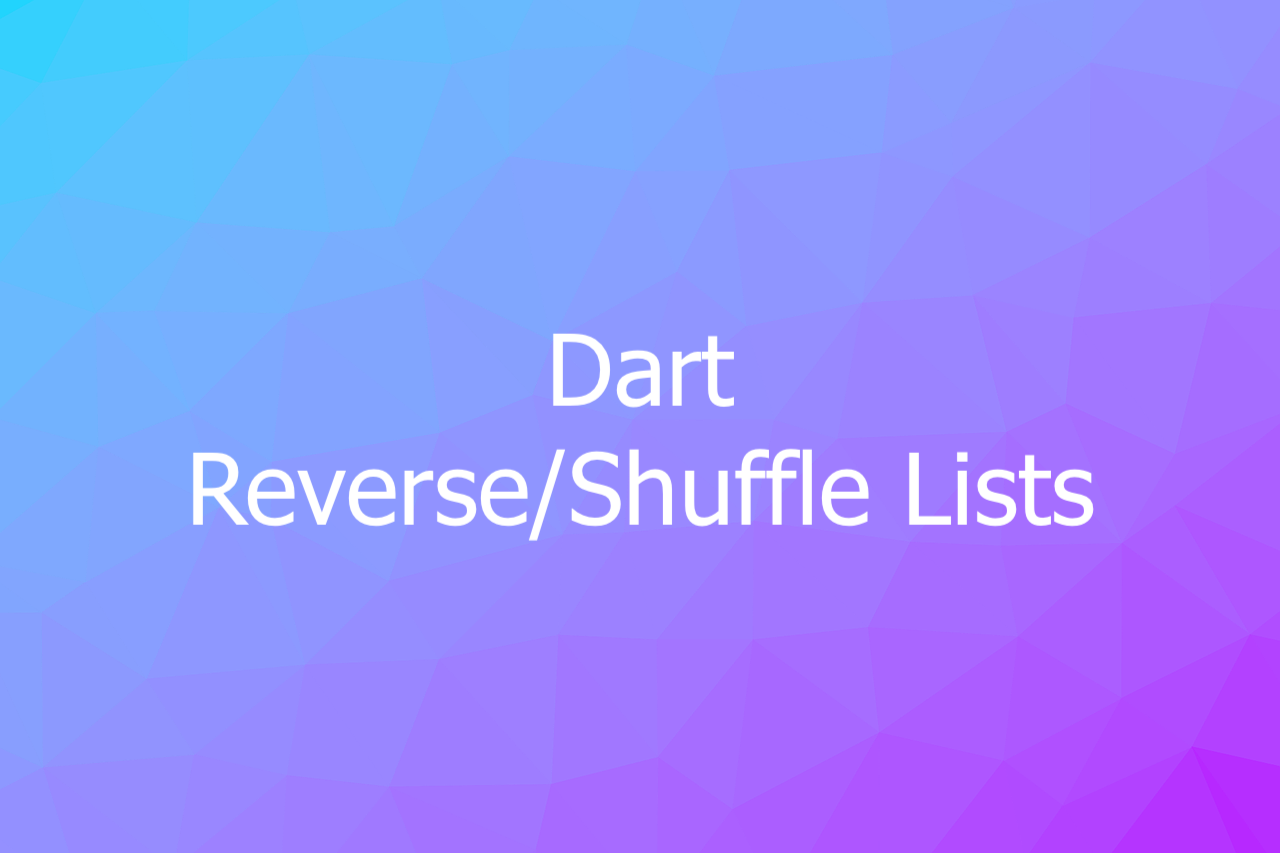
The two examples below show you how to reverse and shuffle a given list in Dart.
Reversing a Dart list
Reversing a list means changing the order of the elements in the list so that the first element becomes the last, the second element becomes the second last, and so on.
Example:
// main.dart
void main() {
final List listOne = [1, 2, 3, 4, 5, 6, 7, 8, 9];
final List listTwo = ['a', 'b', 'c', 'd'];
final List reversedListOne = listOne.reversed.toList();
print(reversedListOne);
final List reversedListTwo = listTwo.reversed.toList();
print(reversedListTwo);
}
Output:
[9, 8, 7, 6, 5, 4, 3, 2, 1]
[d, c, b, a]
Shuffling a Dart list
Shuffling a list means changing the order of the elements in the list randomly so that each possible order has an equal chance of occurring.
In Dart and Flutter, we can easily shuffle a list by using the shuffle() method. Note that this method will change the original list.
Example:
// main.dart
void main() {
final List listOne = [1, 2, 3, 4, 5, 6, 7, 8, 9];
final List listTwo = ['a', 'b', 'c', 'd'];
listOne.shuffle();
listTwo.shuffle();
print(listOne);
print(listTwo);
}
Output:
[5, 1, 9, 4, 2, 3, 7, 8, 6]
[b, c, a, d]
Due to the randomness, you are very likely to receive a different output each time you execute the code.
Further reading:
- Flutter & Dart: 3 Ways to Generate Random Strings
- Dart: Converting a List to a Map and Vice Versa
- Text Overflow in Flutter: Tutorial & Examples
- Flutter: Floating Action Button examples (basic & advanced)
- Flutter: AnimatedOpacity example
- How to Create Rounded ListTile in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.