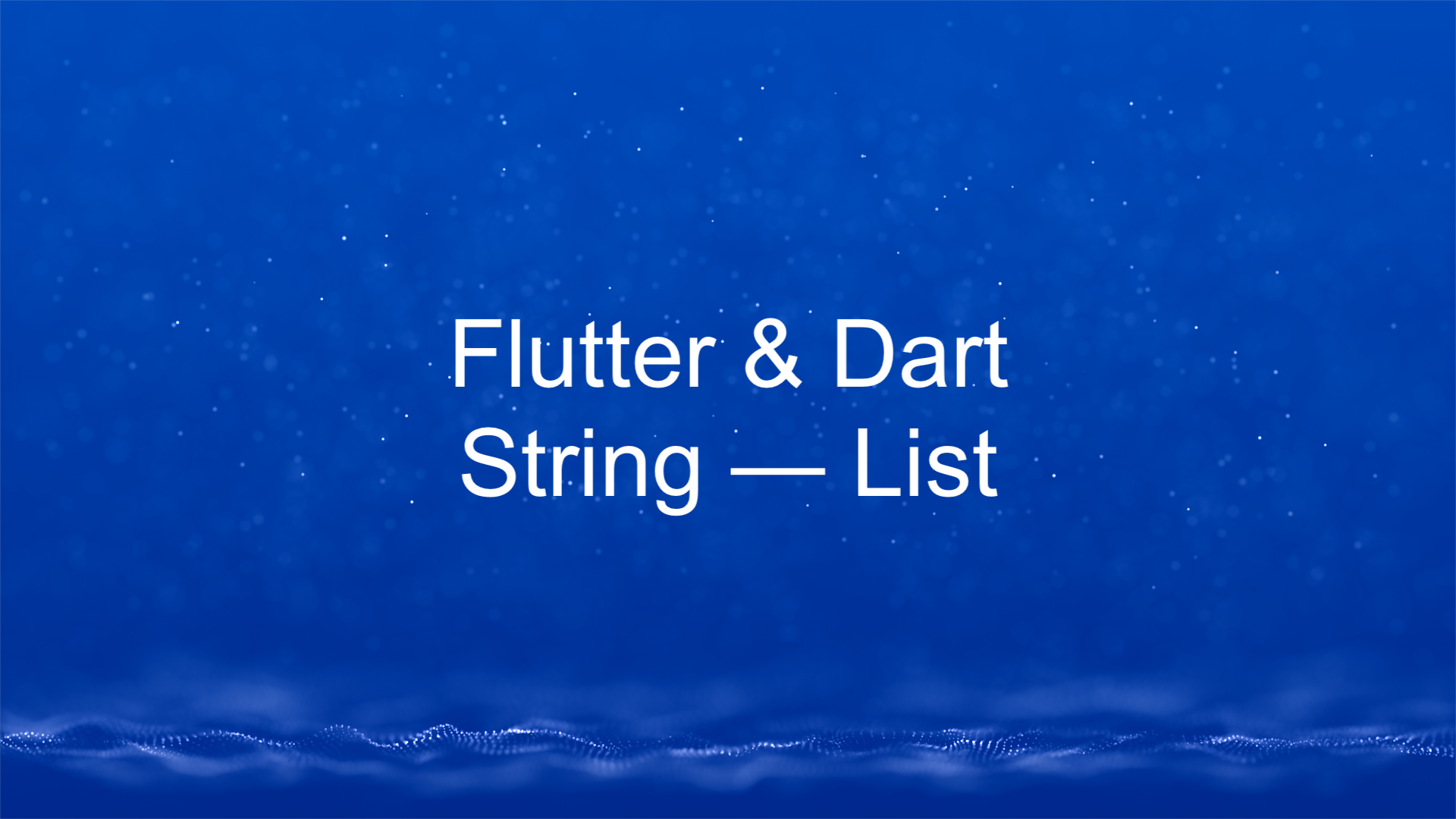
In Flutter and Dart, you can turn a given string into a list (with string elements) by using the split() method.
Example:
// main.dart
void main() {
const String s =
"blue red orange amber green yellow purple pink brown indigo";
final List<String> colors = s.split(' ');
print(colors);
}
Output:
[blue, red, orange, amber, green, yellow, purple, pink, brown, indigo]
In the opposite direction, to convert a list of strings to a string, you can use the join() method.
Example:
// main.dart
void main() {
final List<String> list = [
'dog',
'cat',
'dragon',
'pig',
'monkey',
'cricket'
];
final String result = list.join(', ');
print(result);
}
Output:
dog, cat, dragon, pig, monkey, cricket
Further reading:
- Dart: Extract a substring from a given string (advanced)
- Dart regular expressions to check people’s names
- Dart: Get Strings from ASCII Codes and Vice Versa
- Flutter & Dart: Regular Expression Examples
- Sorting Lists in Dart and Flutter (5 Examples)
- Flutter form validation example
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.