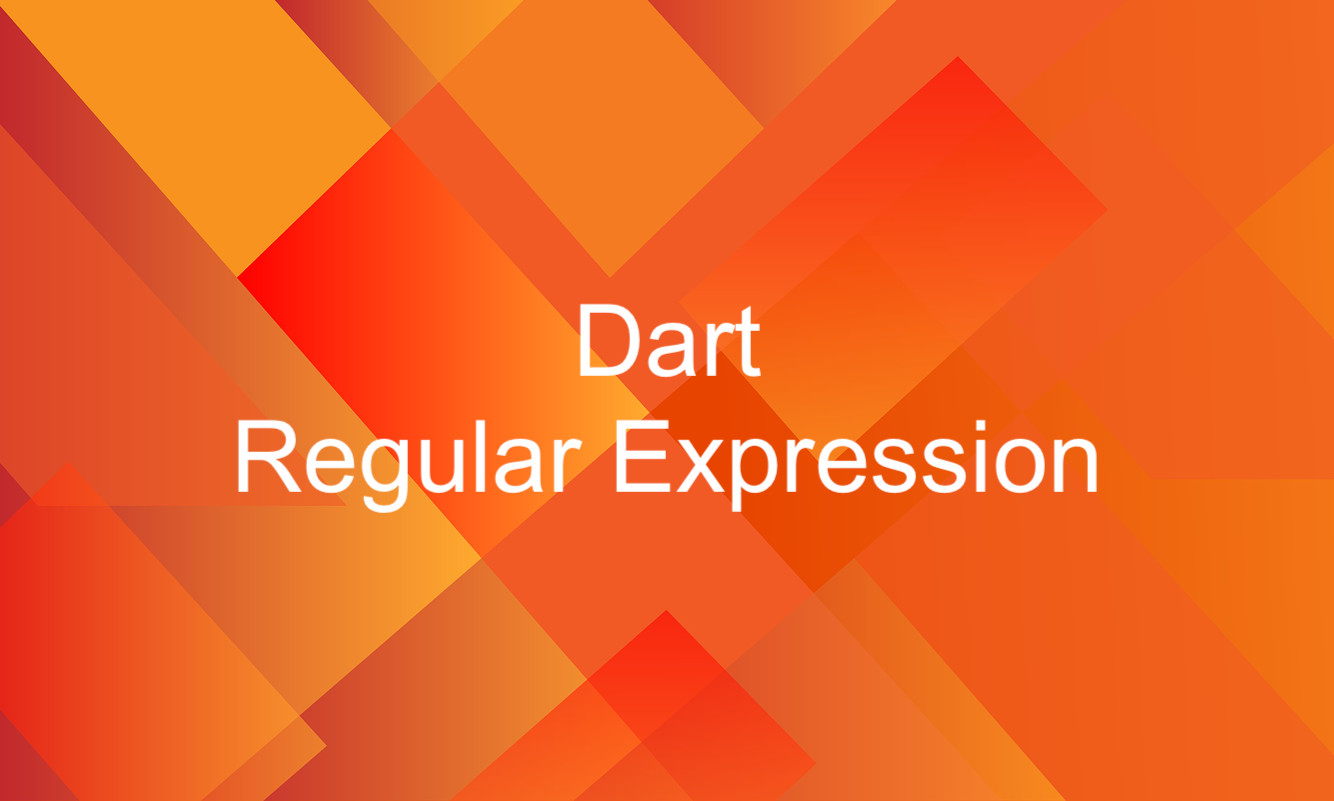
The name of a person (in common languages) usually contains the letters A through Z (lowercase or uppercase), spaces, periods (in abbreviations), and may contain dashes.
Examples of human names:
Donand J. Trump
Geogre W. Bush
Walter White
Brady Hartsfield
Ken Pat O'Biden
A-Crazy-Name Saitama
Vegeta
This is a simple regex pattern to check whether a person’s name is valid in Dart (and Flutter as well):
r"^\s*([A-Za-z]{1,}([\.,] |[-']| ))+[A-Za-z]+\.?\s*$"
Test it:
// kindacode.com
// main.dart
void main() {
// Regular expression to validate a name
RegExp rex = RegExp(r"^\s*([A-Za-z]{1,}([\.,] |[-']| ))+[A-Za-z]+\.?\s*$");
// Expected ouput: True
if (rex.hasMatch("Donand J. Trump")) {
print('True');
} else {
print("False");
}
// Expected ouput: True
if (rex.hasMatch("Brady Hartsfield")) {
print("True");
} else {
print("False");
}
// Expected ouput: True
if (rex.hasMatch("Ken Pat O'Biden")) {
print("True");
} else {
print("False");
}
// Expected ouput: True
if (rex.hasMatch("A-Crazy-Name Saitama")) {
print('True');
} else {
print("False");
}
// Expected ouput: False
if (rex.hasMatch("A wrong name ____ @! ?")) {
print('True');
} else {
print("False");
}
}
Output:
True
True
True
True
False
It should be noted that the above method is mainly for names of people in English-speaking countries.
If you use a language such as Chinese, Korean, Japanese, or another language that is too different from English, the above method may give less accurate (or totally incorrect) results.
That’s it. Futher reading:
- Flutter & Dart: Regular Expression Examples
- Dart regex to validate US/CA phone numbers
- How to create a Filter/Search ListView in Flutter
- How to Create a Stopwatch in Flutter
- Flutter Autocomplete example
- 2 Ways to Add Multiple Floating Buttons in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.