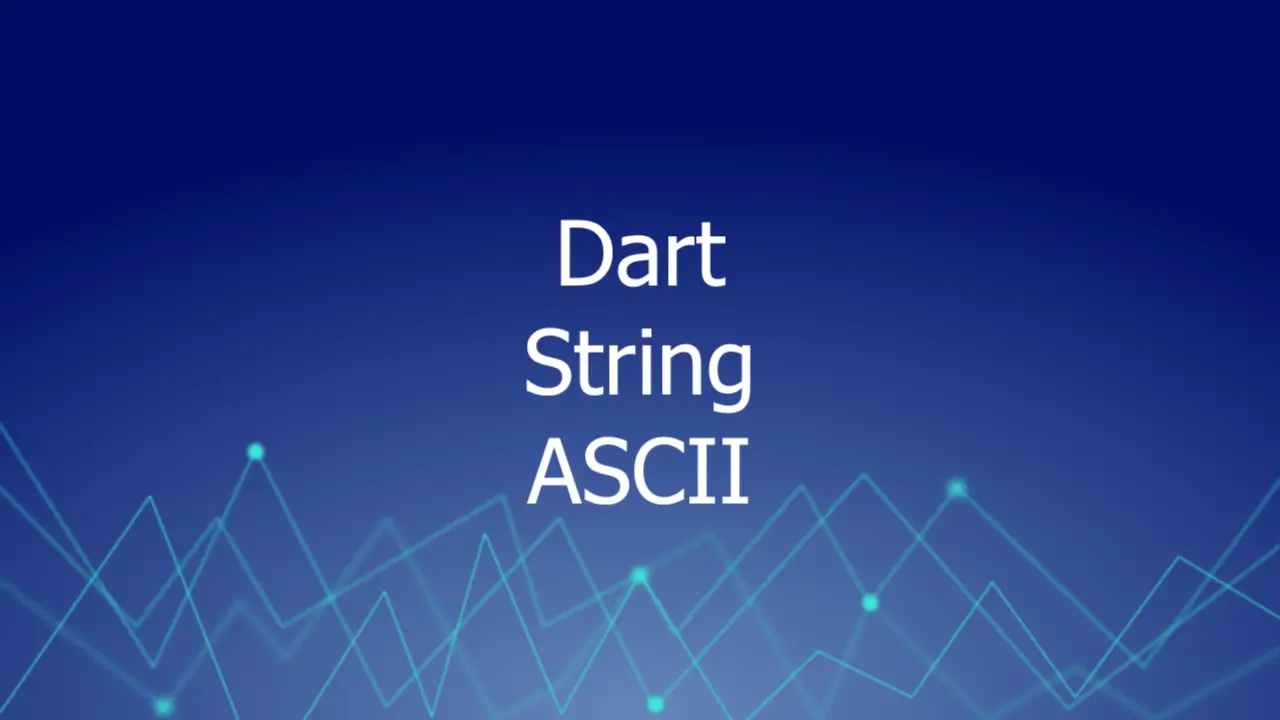
ASCII stands for American Standard Code for Information Interchange, is a character encoding standard that uses a 7-bit binary code to represent 128 possible characters, including letters, digits, punctuation marks, and control codes. For example, the ASCII code for the letter “A” is 65, and the ASCII code for the number 0 is 48.
In this article, you will learn how to create a string from a series of ASCII codes and how to get ASCII codes from a given string in Dart (and Flutter as well).
Note: In ASCII encoding, uppercase letters and lowercase letters have different numeric codes. For example, the ASCII code for the uppercase letter “A” is 65, while the ASCII code for the lowercase letter “a” is 97.
Creating a string from ASCII codes
To get a string from an ASCII code, you can use the String.fromCharCode() method. The example below demonstrates how to use a loop to create a string from a series of ASCII codes:
void main() {
List<int> asciiCodes = [
75,
105,
110,
100,
97,
67,
111,
100,
101,
46,
99,
111,
109
];
String message = '';
for (int code in asciiCodes) {
message += String.fromCharCode(code);
}
print(message);
}
Output:
KindaCode.com
Getting ASCII codes from a string
To get the ASCII code for a character in a string, you can use the codeUnitAt property. In the following example, we will use a loop to get the ASCII code for each character in a given string and store all the codes in a list:
void main() {
String message = "Welcome to KindaCode.com";
List<int> asciiCodes = [];
for (int i = 0; i < message.length; i++) {
asciiCodes.add(message.codeUnitAt(i));
}
print(asciiCodes);
}
Output:
[87, 101, 108, 99, 111, 109, 101, 32, 116, 111, 32, 75, 105, 110, 100, 97, 67, 111, 100, 101, 46, 99, 111, 109]
Real-World Use Cases
Learning to construct strings from ASCII codes (and vice versa) won’t waste your time. It will be useful in various situations.
When working with text data, you may encounter situations where you need to convert between ASCII codes and strings. For example, when reading data from a file that contains ASCII codes instead of text, you would need to convert the codes to their corresponding characters to make sense of the data.
When sending or receiving data over a network, you may need to convert text data to a format that can be transmitted over the network. ASCII codes can be used to represent text data in a compact, binary format that can be easily transmitted.
In some encryption and decryption algorithms, ASCII codes are used to represent text data. In these situations, you would need to convert between ASCII codes and strings to encrypt or decrypt the data.
Final Words
This article might seem boring, but I think it will be helpful in some way. If you find something unclear or incorrect, please let me know by leaving a comment.
To be able to build modern and well-functioning applications, we need to constantly learn and update new technologies. Continue exploring more new and interesting stuff about Flutter and Dart by taking a look at the following articles:
- Dart: How to Remove or Replace Substrings in a String
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- 2 Ways to Create Typewriter Effects in Flutter
- How to Programmatically Take Screenshots in Flutter
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
- Hero Widget in Flutter: Tutorial & Example
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.