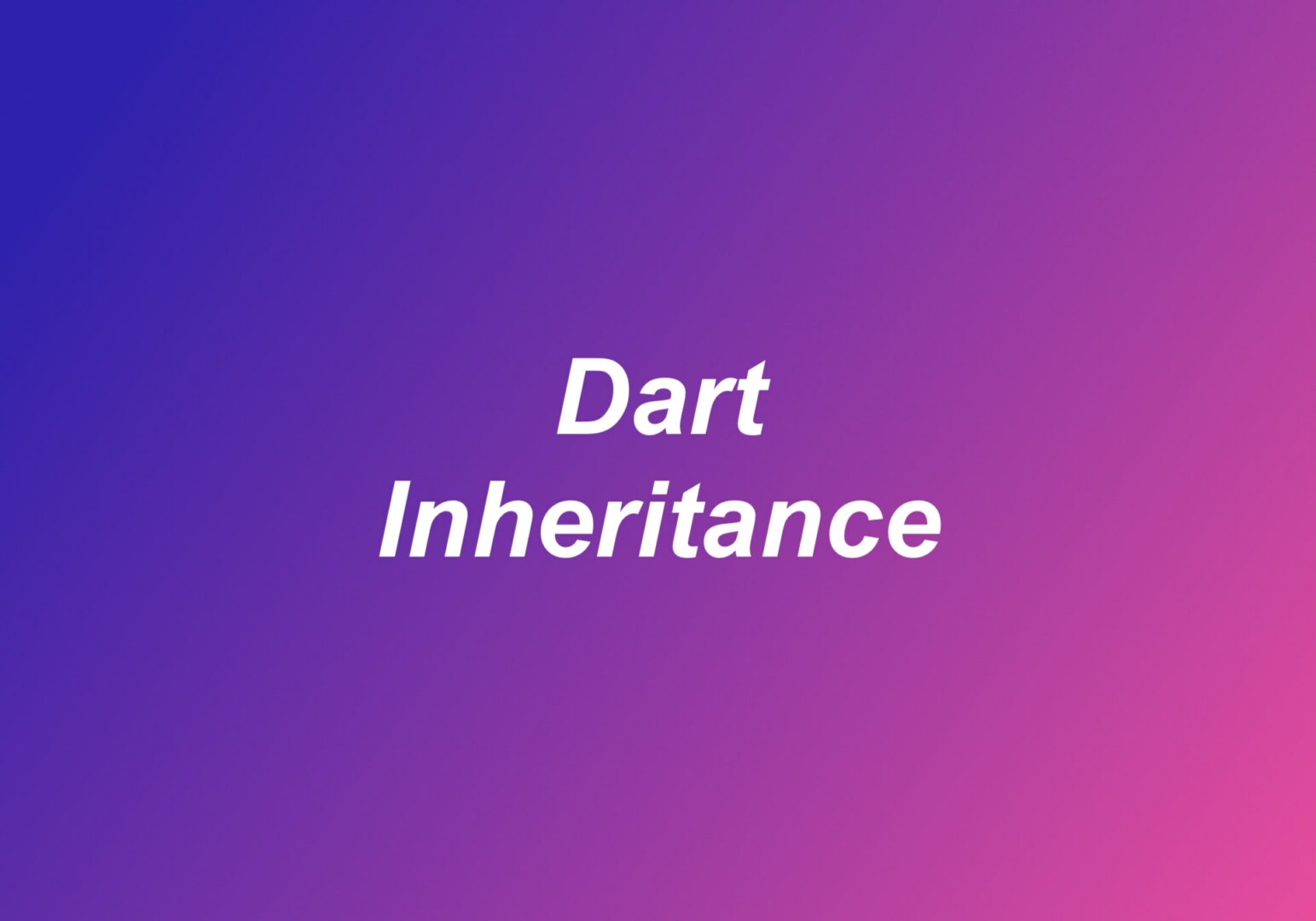
In Dart, you can use the extends keyword with any class when you want to extend the superclass’s functionality. A class can only extend one class since Dart does not support multiple inheritances.
Below is a quick example of implementing inheritance in Dart. Imagine we are building an employee management project for a company. The most general class will be Employee and other classes will inherit it:
import 'package:flutter/foundation.dart';
// Define the Employee class
class Employee {
final String first;
final String last;
Employee(this.first, this.last);
@override
String toString() {
return '$first $last';
}
}
// Define the subclass which extends the Employee class
class Developer extends Employee {
final String _role;
Developer(this._role, String first, String last) : super(first, last);
@override
String toString() {
return '${super.toString()}: $_role';
}
}
void main() {
final dev = Developer('Flutter Developer', 'John', 'Doe');
debugPrint(dev.toString());
}
The preceding code will produce the following output:
John Doe: Flutter Developer
Further reading:
- Using Static Methods in Dart and Flutter
- Prevent VS Code from Auto Formatting Flutter/Dart Code
- Dart: How to Update a Map
- Dart: How to remove specific Entries from a Map
- Sorting Lists in Dart and Flutter (5 Examples)
- 2 Ways to Create Multi-Line Strings in Dart
- Flutter: Full-Screen Semi-Transparent Modal Dialog
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.