In order to make a horizontal GridView in Flutter, just set its scrollDirection property to Axis.horizontal, like this:
GridView(
scrollDirection: Axis.horizontal,
gridDelegate: SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: 200,
childAspectRatio: 3 / 2,
crossAxisSpacing: 20,
mainAxisSpacing: 20
),
children: [
// Some widgets
],
),
Example 1
Demo:
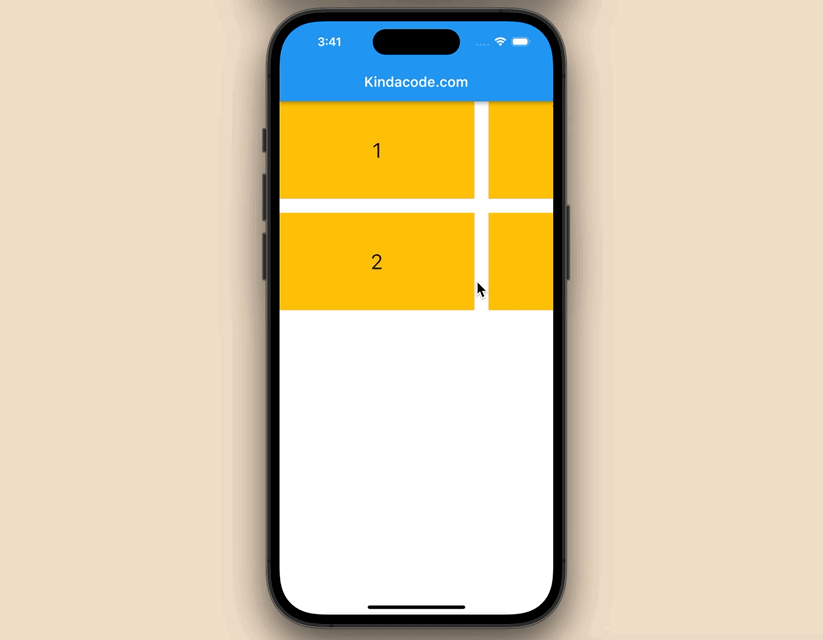
The code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: SafeArea(
child: SizedBox(
width: double.infinity,
height: 300,
child: GridView(
scrollDirection: Axis.horizontal,
gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: 250,
childAspectRatio: 1 / 2,
crossAxisSpacing: 20,
mainAxisSpacing: 20),
children: [
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'1',
style: TextStyle(fontSize: 30),
),
),
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'2',
style: TextStyle(fontSize: 30),
),
),
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'3',
style: TextStyle(fontSize: 30),
),
),
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'4',
style: TextStyle(fontSize: 30),
),
),
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'5',
style: TextStyle(fontSize: 30),
),
),
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'6',
style: TextStyle(fontSize: 30),
),
),
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'7',
style: TextStyle(fontSize: 30),
),
),
Container(
color: Colors.amber,
alignment: Alignment.center,
child: const Text(
'8',
style: TextStyle(fontSize: 30),
),
),
],
),
),
),
);
}
}
Example 2: Using GridView.builder
Screen record:

The code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
HomePage({Key? key}) : super(key: key);
// Generating dummy data for testing purpose
final List dummyData = List.generate(10000, (index) => '$index');
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: SafeArea(
child: SizedBox(
width: double.infinity,
height: 300,
child: GridView.builder(
scrollDirection: Axis.horizontal,
itemCount: dummyData.length,
gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: 250,
childAspectRatio: 3 / 2,
crossAxisSpacing: 20,
mainAxisSpacing: 20),
itemBuilder: (context, index) {
return GridTile(
child: Container(
color: Colors.blue[200],
alignment: Alignment.center,
child: Text(dummyData[index])));
},
),
),
),
);
}
}
Final Words
You’ve walked through a few examples of implementing horizontal grid views. If you’d like to gain more knowledge about the grid stuff and explore more new and interesting stuff about Flutter, take a look at the following articles:
- Creating Masonry Layout in Flutter with Staggered Grid View
- Flutter: GridPaper example
- Flutter: GridTile examples
- Flutter: Safety nesting ListView, GridView inside a Column
- Flutter SliverList – Tutorial and Example
- Best Libraries for Making HTTP Requests in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.
Example 1 getting me the error when i use it insdie customScrollView
package:flutter/src/widgets/overscroll_indicator.dart’: Failed assertion: line 720 pos 14: ‘notification.metrics.axis == widget.axis’: is not true.
how to solve it
You should not put it inside a custom scroll view