This article is about hiding and showing the status bar in Flutter applications.
Overview
A status bar presents some information such as time, wifi, battery life, etc. Here is the status bar on iOS (the left one) and Android (the right one) that are shown by default:

To hide them, the first thing you need to do is import the services library:
import 'package:flutter/services.dart';
Then we need to call the SystemChrome.setEnabledSystemUIMode method (this is the replacement for the SystemChrome.setEnabledSystemUIOverlays method which is now depreciated). Add these lines to the main() function before runApp():
void main() {
// Add these 2 lines
WidgetsFlutterBinding.ensureInitialized();
SystemChrome.setEnabledSystemUIOverlays([
SystemUiOverlay.bottom, //This line is used for showing the bottom bar
]);
// Then call runApp() as normal
runApp(MyApp());
}
The code above will remove the status bar from all screens. If you only want to remove them from a specific screen, place the settings code inside the initState() method instead:
@override
void initState() {
super.initState();
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [
SystemUiOverlay.bottom,
]);
}
If you want to show the status bar again, just place the SystemUiOverlay.top option inside the square brackets or remove the SystemChrome.setEnabledSystemUIMode function.
A Complete Example
Preview
Here are the screens when the status bar are gone away:
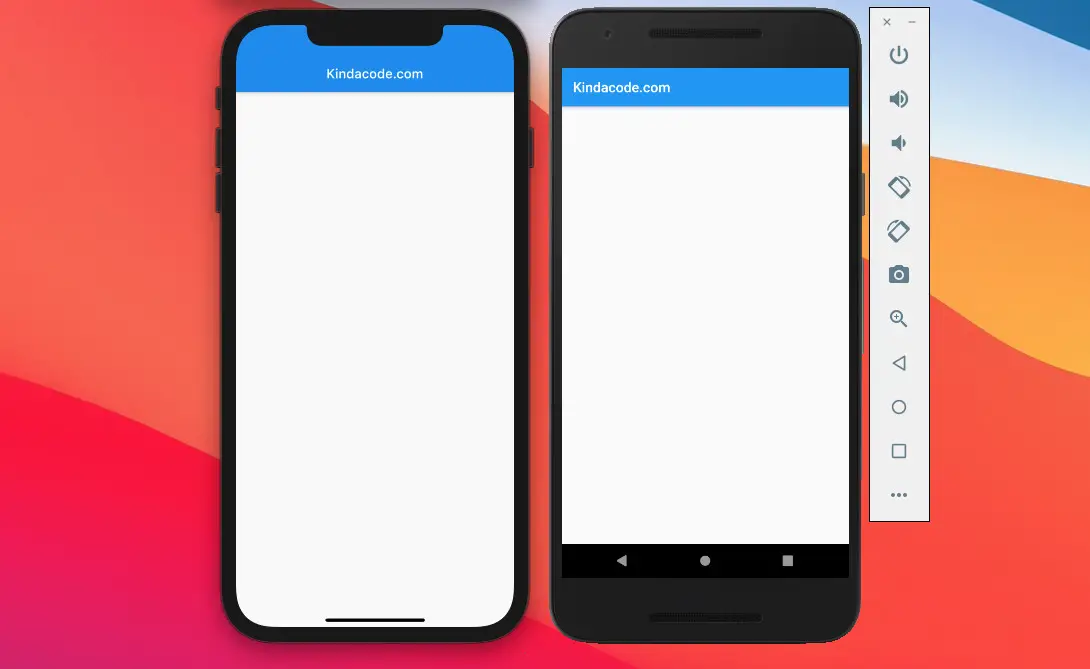
The Code
Choose one of the 2 approaches below to suit your needs.
Modifying the main() function
This will hide the status bar across the app:
// KindaCode.com
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
// Add these 2 lines
WidgetsFlutterBinding.ensureInitialized();
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [
SystemUiOverlay.bottom, //This line is used for showing the bottom bar
]);
// Then call runApp() as normal
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Kindacode.com',
),
),
body: Container(),
);
}
}
Using initState()
This will hide the status bar on the HomePage screen only:
// KindaCode.com
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
void initState() {
super.initState();
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [
SystemUiOverlay.bottom,
]);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Kindacode.com',
),
),
body: Container(),
);
}
}
Wrapping Up
You have learned how to disable the status bar for Android and iOS by calling the SystemChrome.setEnabledSystemUIMode method from the services library. If you would like to learn more about Flutter, see the following articles:
- Flutter: How to Read and Write Text Files
- Flutter SliverList – Tutorial and Example
- How to disable Landscape mode in Flutter
- Flutter: Load and display content from CSV files
- Flutter: GridPaper example
- Creating Masonry Layout in Flutter with Staggered Grid View
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.