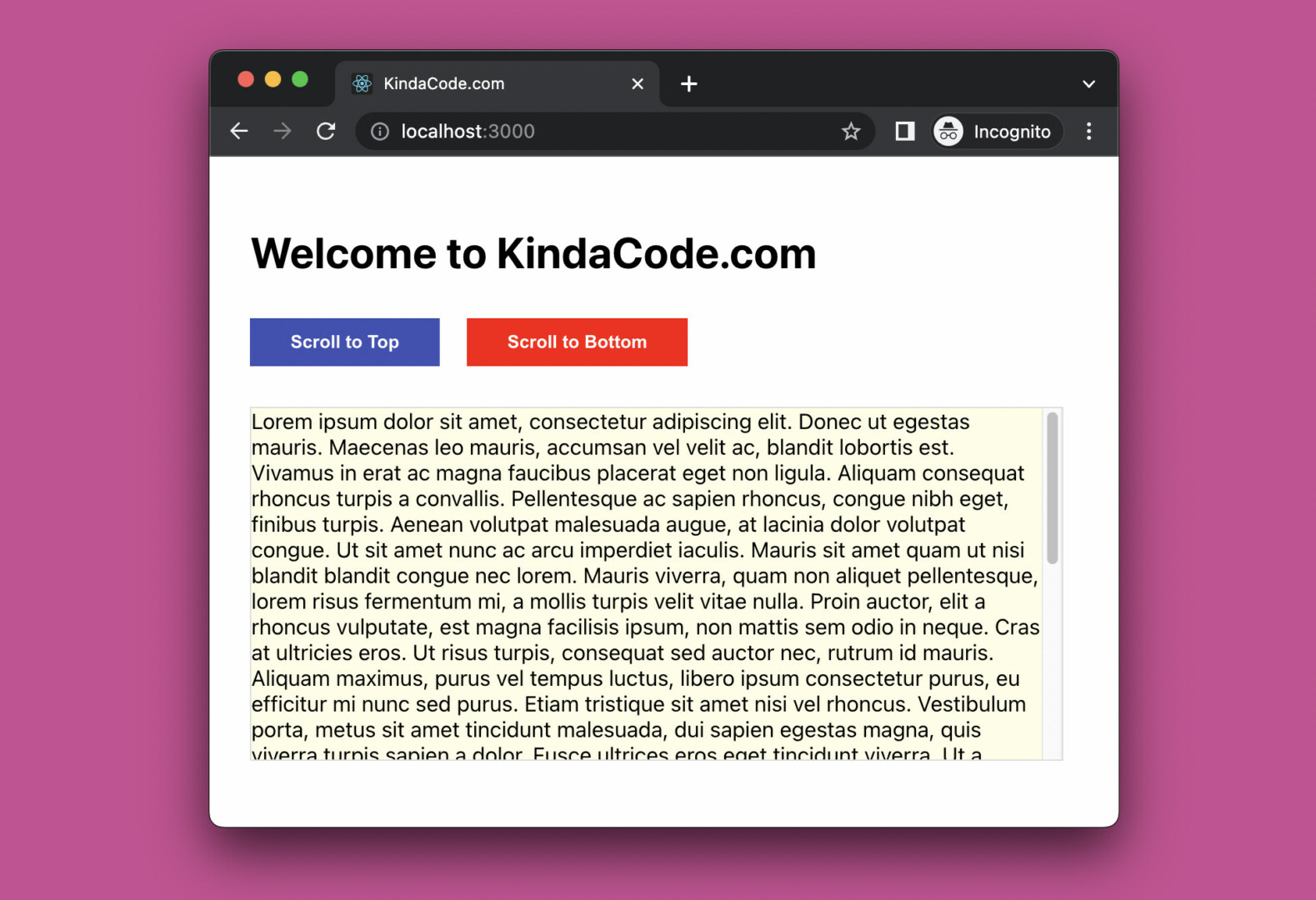
This concise and practical article shows you how to programmatically scroll to the bottom or to the top of a scrollable div element in React.
The TL;DR
Here is the process that we will go through:
1. Create a ref with the useRef() hook:
const divRef = useRef();
2. Attach the ref to the div element:
<div ref={divRef}>{/* ...*/}</div>
3. To smoothly scroll to the bottom of the div, use this:
divRef.current.scroll({
top: divRef.current.scrollHeight,
behavior: 'smooth'
});
4. To scroll to the top of the div gently, use this:
divRef.current.scroll({ top: 0, behavior: 'smooth' });
Let’s tie all this stuff into a working example.
The Full Example
App Preview
The demo we’re going to make displays a scrollable div that contains a ton of text. We also have 2 buttons. One is used to scroll the div to the bottom and the other is used to scroll the div to the top.
An animated GIF screenshot is worth more than a thousand of words:

The Code
1. Create a new React project:
npx creat-react-app kindacode-scrollable-div
From this time forward, we’ll only focus on 2 files: src/App.js and src/App.css.
2. The complete code for src/App.js (with explanations):
// KindaCode.com
// src/App.js
import { useRef } from 'react';
import './App.css';
function App() {
// Create a reference to the div with className="scrollable-div"
const divRef = useRef(null);
// This function is called when the user clicks on the "Scroll To Top" button
// it will scroll the div with className="scrollable-div" to the top
const scrollToTop = () => {
divRef.current.scroll({ top: 0, behavior: 'smooth' });
};
// This function is called when the user clicks on the "Scroll To Bottom" button
// it will scroll the div with className="scrollable-div" to the bottom
const scrollToBottom = () => {
divRef.current.scroll({ top: divRef.current.scrollHeight, behavior: 'smooth' });
};
return (
<div className='container'>
<h1>Welcome to KindaCode.com</h1>
<div className='button-group'>
<button onClick={scrollToTop} className='button'>
Scroll to Top
</button>
<button onClick={scrollToBottom} className='button'>
Scroll to Bottom
</button>
</div>
{/* This div displays a lot of dummy text and is scrollable */}
{/* We will programatically scroll it to the top/bottom */}
<div ref={divRef} className='scrollable-div'>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec ut
egestas mauris. Maecenas leo mauris, accumsan vel velit ac, blandit
lobortis est. Vivamus in erat ac magna faucibus placerat eget non
ligula. Aliquam consequat rhoncus turpis a convallis. Pellentesque ac
sapien rhoncus, congue nibh eget, finibus turpis. Aenean volutpat
malesuada augue, at lacinia dolor volutpat congue. Ut sit amet nunc ac
arcu imperdiet iaculis. Mauris sit amet quam ut nisi blandit blandit
congue nec lorem. Mauris viverra, quam non aliquet pellentesque, lorem
risus fermentum mi, a mollis turpis velit vitae nulla. Proin auctor,
elit a rhoncus vulputate, est magna facilisis ipsum, non mattis sem odio
in neque. Cras at ultricies eros. Ut risus turpis, consequat sed auctor
nec, rutrum id mauris. Aliquam maximus, purus vel tempus luctus, libero
ipsum consectetur purus, eu efficitur mi nunc sed purus. Etiam tristique
sit amet nisi vel rhoncus. Vestibulum porta, metus sit amet tincidunt
malesuada, dui sapien egestas magna, quis viverra turpis sapien a dolor.
Fusce ultrices eros eget tincidunt viverra. Ut a dapibus erat, vel
cursus odio. Phasellus erat enim, volutpat vel lacus eu, aliquam sodales
turpis. Fusce ipsum ex, vehicula tempor accumsan nec, gravida at eros.
In aliquam, metus id mollis interdum, nunc sem dignissim quam, non
iaculis tortor erat nec eros. Nunc sollicitudin ac dolor eget lobortis.
Aenean suscipit rutrum dolor in euismod. Curabitur quis dapibus lectus.
Mauris enim leo, condimentum ac elit sit amet, iaculis vulputate sem.
Integer bibendum ipsum nec sodales malesuada. Fusce eu nisi quis diam
maximus rutrum. Nulla elementum turpis lacus, ut facilisis est ornare
eget. Lorem ipsum dolor sit amet, consectetur adipiscing elit. Aliquam
dictum mauris nec tellus facilisis vehicula. Nunc mattis, lorem ac
pharetra bibendum, nisl mi gravida justo, condimentum feugiat eros
tellus vel velit. Nulla a elementum nulla. Donec tincidunt nunc nec
libero faucibus, id maximus libero porttitor. Curabitur vel libero
scelerisque, sodales elit in, fermentum odio. Phasellus rutrum eleifend
sapien, eu congue turpis blandit varius. Quisque fringilla felis
interdum magna vulputate, id tincidunt diam lacinia. Suspendisse ut
vehicula leo. Morbi ornare blandit felis vitae maximus. Aenean hendrerit
consectetur orci vitae interdum.
</div>
</div>
);
}
export default App;
3. CSS is important for this example. Remove all of the default code in your src/App.css file, then add the following into it:
/* KindaCode.com */
/* src/App.css */
.container {
padding: 30px;
}
.button-group {
margin: 30px 0;
}
.button {
margin-right: 20px;
padding: 10px 30px;
cursor: pointer;
border: none;
background: #3f51b5;
color: #fff;
font-weight: bold;
}
.button:hover {
background: red;
}
.scrollable-div {
width: 600px;
height: 260px;
overflow: auto;
background: #fffde7;
border: 1px solid #ddd;
}
Done. Run the project and contemplate your work.
Epilogue
We’ve explored the technique of scrolling to the bottom or to the top of a div element in React and traversed an end-to-end example of applying that technique in practice. Try to modify the code, change some CSS values, remove some lines of code, and see what happens next.
If you’d like to learn more new and interesting things about modern React, continue your adventure with the following articles:
- React: Update Arrays and Objects with the useState Hook
- React: How to Check Internet Connection (Online/Offline)
- React: Get the Width & Height of a dynamic Element
- React Router: How to Highlight Active Link
- React + TypeScript: Multiple Dynamic Checkboxes
- React Router: How to Create a Custom Back Button
You can also check our React category page and React Native category page for the latest tutorials and examples.