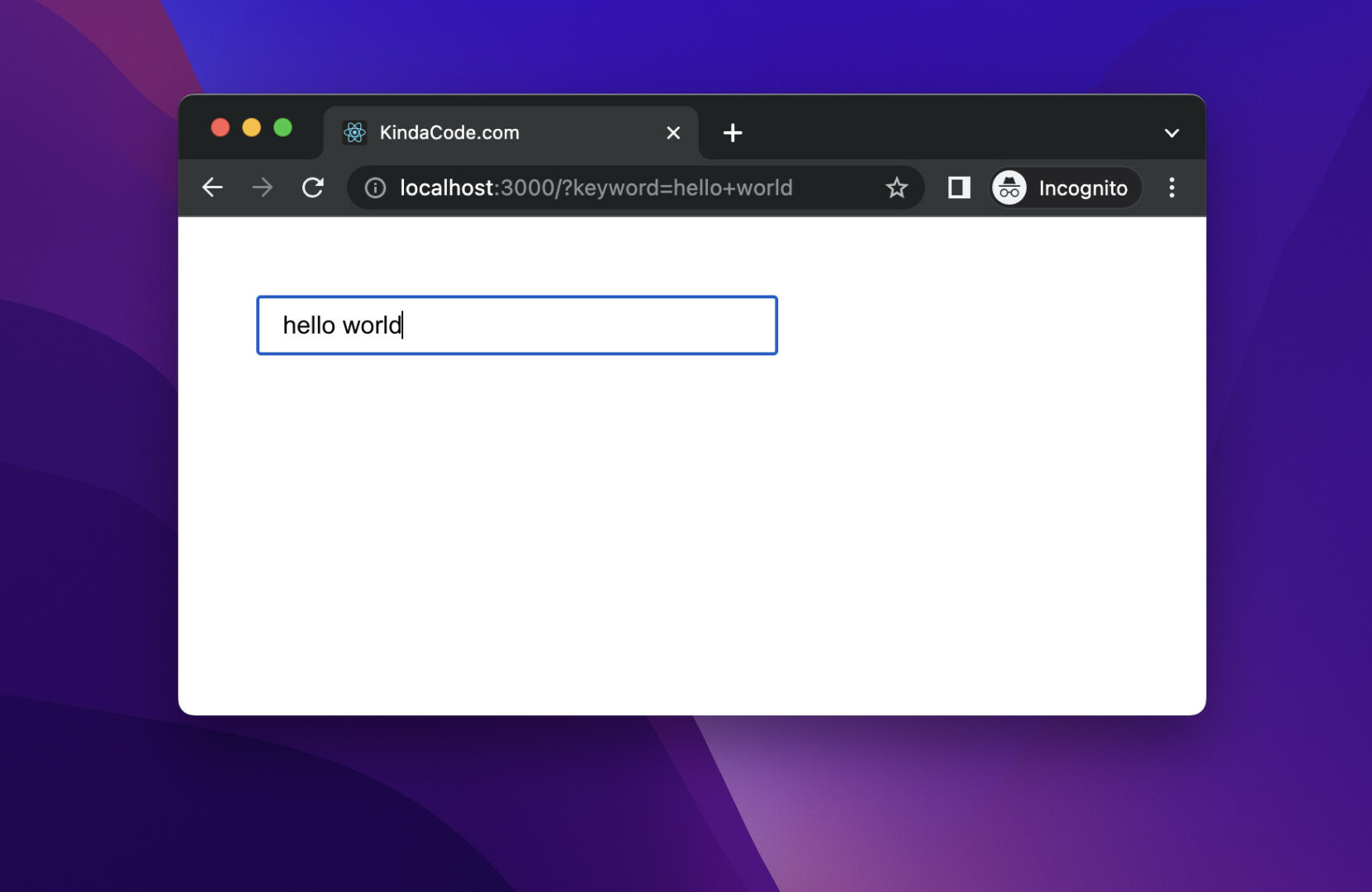
This article is about the useParams and useSearchParams hooks that come with React Router. We’ll cover the fundamentals of these hooks and then walk through a complete example of using them in practice.
Note: We’ll use the latest version of React Router (6.x)
Table of Contents
useParams hook
The useParams hook is used to read the dynamic params from the current URL that were matched by <Route path>. For example, if a route looks like this:
/some-path/:name/:id
Then the useParams hook will return an object with key/value pairs looking like so:
{
name: <some value>,
id: <some value>
}
Example
Preview:
The tiny app we’re going to build consists of two pages: Home and Post. The path of the Post page looks like this:
/post/:id/:slug
The user can navigate to the Post page by using one of the links on the Home page. Here’s how it works:
The full code:
import {
Routes,
Route,
BrowserRouter,
Link,
useParams,
} from 'react-router-dom'
function App() {
return (
<BrowserRouter>
<Routes>
<Route path='/' element={<Home />} />
<Route path='/post/:id/:slug' element={<Post />} />
</Routes>
</BrowserRouter>
);
}
// Home page
// Route: /
const Home = () => {
return <div style={{ padding: 30 }}>
<p><Link to={`/post/123/hello-world`}>/post/123/hello-world</Link></p>
<p><Link to={`/post/456/goodbye-moon`}>/post/345/goodbye-moon</Link></p>
</div>
}
// Post page
// Route: /post/:id/:slug
const Post = () => {
const params = useParams();
return <div style={{ padding: 30 }}>
<h1>ID: {params.id}</h1>
<h2>Slug: {params.slug}</h2>
</div>
}
export default App;
useSearchParams hook
The useSearchParams hook is used to Read and Modify the query string in the URL for the current location. Similar to the useState hook of React, the useSearchParams hook of React Router returns an array with two elements: the first is the current location’s search params, and the latter is a function that can be used to update them:
import { useSearchParams } from 'react-router-dom';
const App = () => {
const [searchParams, setSearchParams] = useSearchParams();
return /* ... */
}
To see how it works in action, move on to the following example.
Example
Preview
In this small example, when the text in the search field changes, the search portion of the URL changes too (the part after the question mark ?):
The complete code:
import {
Routes,
Route,
BrowserRouter,
useSearchParams
} from 'react-router-dom'
function App() {
return (
<BrowserRouter>
<Routes>
<Route path='/' element={<Search />} />
</Routes>
</BrowserRouter>
);
}
// Search page
const Search = () => {
const [searchParams, setSearchParams] = useSearchParams();
// This function will be called whenever the text input changes
const searchHandler = (event) => {
let search;
if (event.target.value) {
search = {
keyword: event.target.value
}
} else {
search = undefined;
}
setSearchParams(search, { replace: true });
}
return <div style={{ padding: 50 }}>
<input
value={searchParams.keyword}
onChange={searchHandler}
placeholder='Search...'
style={{ width: '300px', padding: '8px 15px', fontSize: '16px' }}
/>
</div>
}
export default App;
Conclusion
We’ve learned almost everything about the useParams and useSearchParams hooks. From this point, you can make use of them to solve your problems creatively and flexibly. If you’d like to explore more new and interesting stuff about modern React, take a look at the following articles:
- Working with the useId() hook in React
- React Router: How to Highlight Active Link
- React Router: How to Highlight Active Link
- React Router: 3 Ways to Disable/Inactivate a Link
- React: Show Image Preview before Uploading
- Best open-source Admin Dashboard libraries for React
You can also check our React category page and React Native category page for the latest tutorials and examples.