
This article shows you how to determine the width and height of a non-fixed-size component in React. We’ll use new features of React, including hooks and functional components. You won’t see old-fashioned stuff like class-based components or things related to them.
Overview
The size of an element may depend on many factors, such as:
- Window resizing
- The element contains dynamic content and changeable children that are loaded from a remote server or modified by the user
- Animation effects
To calculate the width and height of an element, we can use the useRef hook:
const myRef = useRef();
// Width
const width = myRef.current.clientWidth;
// Height
const height = myRef.current.clientWidth;
For more clarity, please see the complete example below.
The Example
The app we are going to build contains a button, a list of items, and some text that represents the width and height of the list.
- When the user presses the button, a new item will be added to the list and the height of the list will increase as well.
- When the window gets resized, the width of the list changes.
The three hooks we are going to use are useRef, useState, and useEffect.
Preview
A demo is worth more than a thousand words:
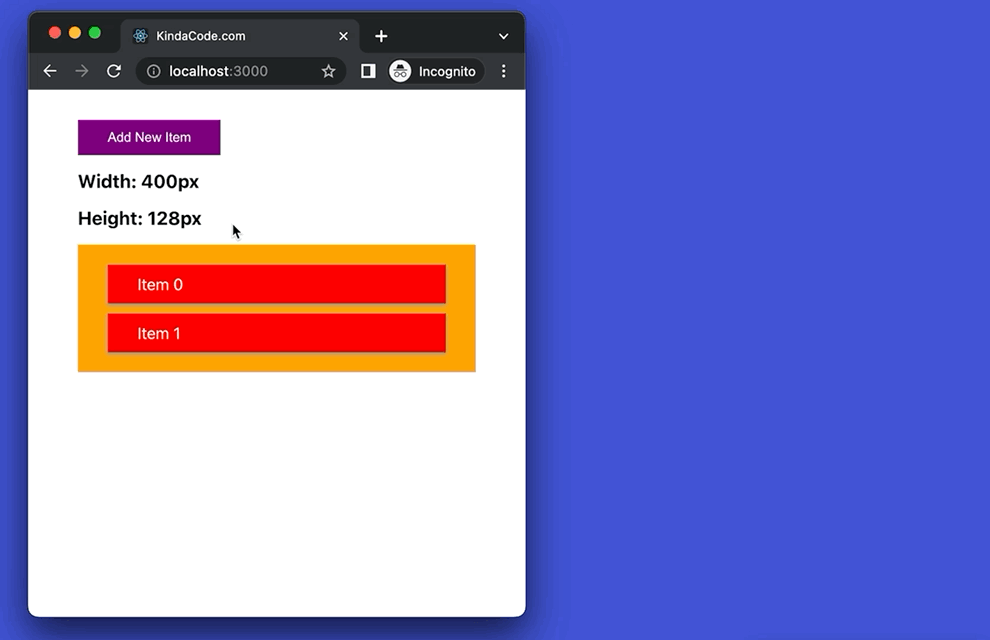
The Code
1. Create a new React project:
npx create-react-app kindacode_react
2. Full source code in src/App.js with explanations:
// App.js
// Kindacode.com
import { useState, useEffect, useRef } from "react";
import "./App.css";
const App = () => {
// This ref is connected to the list
const listRef = useRef();
// The size of the list
// It will be updated later
const [width, setWidth] = useState();
const [height, setHeight] = useState();
// The data of the list at the beginning
const [listItems, setListItems] = useState([
{
id: 0,
title: "Item 0",
},
{
id: 1,
title: "Item 1",
},
]);
// This function is trggered when the "Add new item" button gets clicked
const addItem = () => {
const items = [...listItems];
const newItem = {
id: items.length + 1,
title: `Item ${items.length + 1}`,
};
items.push(newItem);
setListItems(items);
};
// This function calculates width and height of the list
const getListSize = () => {
const newWidth = listRef.current.clientWidth;
setWidth(newWidth);
const newHeight = listRef.current.clientHeight;
setHeight(newHeight);
};
// Get 'width' and 'height' after the initial render and every time the list changes
useEffect(() => {
getListSize();
}, [listItems]);
// Update 'width' and 'height' when the window resizes
useEffect(() => {
window.addEventListener("resize", getListSize);
}, []);
return (
<div className="container">
<button className="button" onClick={addItem}>
Add New Item
</button>
{width && <h3>Width: {width}px</h3>}
{height && <h3>Height: {height}px</h3>}
<ul className="list" ref={listRef}>
{listItems.map((item) => (
<li className="item" key={item.id}>
{item.title}
</li>
))}
</ul>
</div>
);
};
export default App;
3. src/App.css:
.container {
width: 80vw;
margin: 30px auto;
}
.list {
list-style: none;
background: orange;
padding: 10px 30px;
}
.item {
background: red;
color: white;;
box-shadow: 0 2px 4px #999;
padding: 10px 30px;
margin: 10px 0;
}
.button {
border: none;
outline: none;
background: purple;
color: white;
padding: 10px 30px;
}
.button:hover {
background: orange;
}
h3 {
margin: 15px 0;
}
4. Run your project and check the result at http://localhost:3000:
npm start
See also: Get the Position (X & Y) of an Element in React
Conclusion
You’ve learned how to get the size of a component that contains dynamic content and children. If you’d like to explore more new and awesome things in modern React, take a look at the following articles:
- React + TypeScript: Working with Radio Button Groups
- React + TypeScript: Create an Autosize Textarea from scratch
- React: Using inline styles with the calc() function
- React: Create an Animated Side Navigation from Scratch
- 5 best open-source WYSIWYG editors for React
- Most popular React Component UI Libraries
You can also check our React category page and React Native category page for the latest tutorials and examples.
Yeah, Simply Very Nice. It was very helpful to me.
Simply salved me, thanks a lot!
Happy coding
Thanks! Very helpful for what I was doing.
Happy coding
Thank you very much.
Happy coding