When using inline styles in a React project that is written in TypeScript, you may encounter some annoying problems.
For example, the code below:
// App.tsx
// Kindacode.com
import React from "react";
const App: React.FunctionComponent = () => {
return <div style={styles.container}></div>;
};
const styles = {
container: {
fontWeight: "bold",
},
};
export default App;
will result in an error like this:
/Users/goodman/Desktop/Test/react_ts_kindacode/src/App.tsx
TypeScript error in /Users/goodman/Desktop/Test/react_ts_kindacode/src/App.tsx(5,15):
Type '{ fontWeight: string; }' is not assignable to type 'Properties<string | number, string & {}>'.
Types of property 'fontWeight' are incompatible.
Type 'string' is not assignable to type 'FontWeight | undefined'. TS2322
3 | import React from "react";
4 | const App: React.FunctionComponent = () => {
> 5 | return <div style={styles.container}></div>;
| ^
6 | };
7 |
8 | const styles = {
Similar errors can also occur with some common properties like flexDirection, position, etc.
Solutions
We have more than one solution to get rid of the above issue.
1. Casting styles as const
// App.tsx
import React from "react";
const App: React.FunctionComponent = () => {
return (
<div style={styles.container}>
<h3>Kindacode.com</h3>
</div>
);
};
const styles = {
container: {
width: 300,
height: 300,
margin: '50px auto',
backgroundColor: "orange",
display: "flex",
flexDirection: "column",
justifyContent: 'center',
alignItems: 'center',
fontWeight: "bold",
},
} as const;
export default App;
Screenshot:
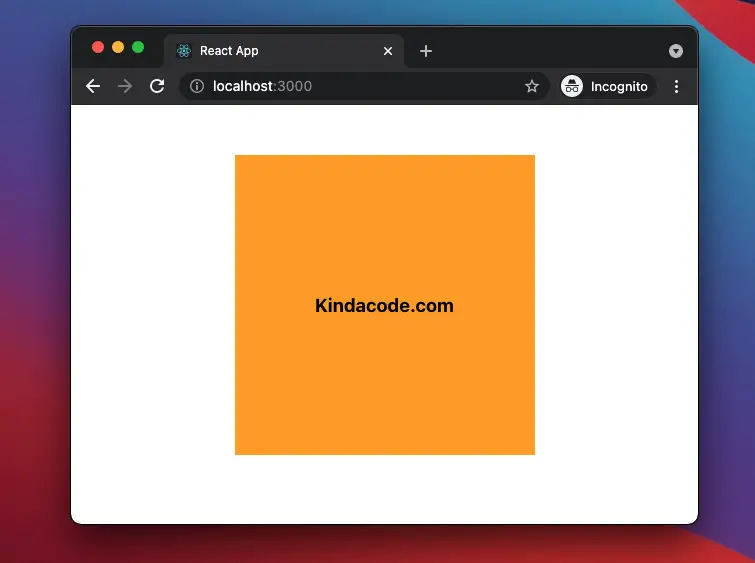
2. Using { [key: string]: React.CSSProperties }
You can modify the code of the first solution as this:
const styles: { [key: string]: React.CSSProperties } = {
container: {
width: 300,
height: 300,
margin: "50px auto",
backgroundColor: "orange",
display: "flex",
flexDirection: "column",
justifyContent: "center",
alignItems: "center",
fontWeight: "bold",
},
};
3. Casting each child of styles as React.CSSProperties
You can change the code in the first solution to this:
const styles = {
container: {
width: 300,
height: 300,
margin: "50px auto",
backgroundColor: "orange",
display: "flex",
flexDirection: "column",
justifyContent: "center",
alignItems: "center",
fontWeight: "bold",
} as React.CSSProperties,
};
Conclusion
We’ve gone through a few approaches to make TypeScript happy and stop warning when using inline styles. If you would like to explore more about modern React and TypeScript, take a look at the following:
- React + TypeScript: Create an Autosize Textarea from scratch
- React + TypeScript: Handling Select onChange Event
- React + TypeScript: Handling onFocus and onBlur events
- React & TypeScript: Using useRef hook example
- React + TypeScript: Drag and Drop Example
- React + TypeScript: Using setTimeout() with Hooks
- React + TypeScript: setInterval() example (with hooks)
You can also check our React category page and React Native category page for the latest tutorials and examples.