WYSIWYG (What You See Is What You Get or Rich Text) editors are commonly used on many websites and web apps related to newspapers, blogs, e-commerce, forums, and online communities to help users publish and edit articles, product information, directions manual, etc. with functions such as alignment, change font size and text color, insert images, and so on.
This article walks you through a list of the best and most popular open-source WYSIWYG editors for React. Their order is based on the number of stars they receive on Github and the number of downloads per week on npmjs.com.
Draft.js
Summary:
- GitHub stars: 22.5k+
- Weekly NPM downloads: 900k -1.1m
- Links: GitHub repo | npm page | live demo | official website
- License: MIT
- Written in: Javascript, CSS
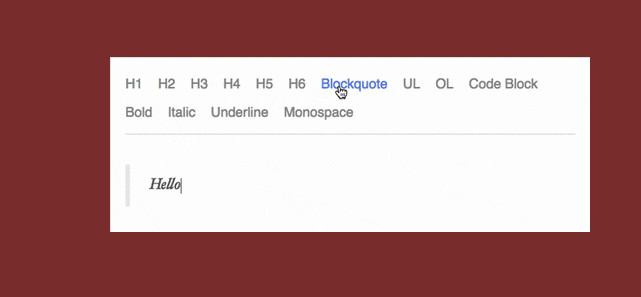
Draft.js is a highly extensible and customizable JavaScript rich-text editor framework built for React and fits seamlessly into React applications, abstracting away the details of rendering, selection, and input behavior with a familiar declarative API.
Sample use:
import React from 'react';
import ReactDOM from 'react-dom';
import {Editor, EditorState} from 'draft-js';
const MyEditor = props => {
const [editorState, setEditorState] = React.useState(
EditorState.createEmpty()
);
const editor = React.useRef(null);
function focusEditor() {
editor.current.focus();
}
React.useEffect(() => {
focusEditor()
}, []);
return (
<div onClick={focusEditor}>
<Editor
ref={editor}
editorState={editorState}
onChange={editorState => setEditorState(editorState)}
/>
</div>
);
}
React Quill
Summary:
- GitHub stars: 6.5k+
- Weekly NPM downloads: 500k – 650k
- Links: GitHub repo | npm page | live demo
- License: MIT
- Written in: Javascript, TypeScript
React Quill is a rich text editor that is fully compatible with React hooks and TypeScript. It provides 3 built-in themes:
- Snow: A Quill’s standard appearance,
- Bubble: Similar to the inline editor on Medium
- Core theme: Contains only the bare essentials to allow modules like toolbars or tooltips to work.

Sample code:
import React, { useState } from "react";
import ReactQuill from 'react-quill';
import 'react-quill/dist/quill.snow.css';
const MyEditor = () => {
const [value, setValue] = useState('');
return (
<ReactQuill theme="snow" value={value} onChange={setValue}/>
);
}
Slate React
Summary:
- GitHub stars: 28.28+
- Weekly NPM downloads: 700k – 900k
- Links: GitHub repo | npm page | live demo | official website
- License: MIT
- Written in: Javascript, TypeScript, CSS
Slate lets you build rich, intuitive editors like those in Medium, Dropbox Paper, or Google Docs without your codebase getting mired in complexity. t can do this because all of its logic is implemented with a series of plugins, so you aren’t ever constrained by what is or isn’t in “core”.

React Draft Wysiwyg
Summary:
- GitHub stars: 6.3k+
- Weekly NPM downloads: 250k -350k
- Links: GitHub repo | npm page | live demo
- License: MIT
- Written in: Javascript, CSS

This is a lightweight rich text editor built on top of ReactJS and DraftJS. It provides lots of features:
- Configurable toolbar with the option to add/remove controls.
- Option to change the order of the controls in the toolbar.
- Support for block types: Paragraph, H1 – H6, Blockquote, Code.
- Support for coloring text or background.
- Support for adding/uploading images.
- Option of undo and redo.
And many more…
Official TinyMCE React component
Summary:
- GitHub stars: 910+
- Weekly NPM downloads: 300k – 400k
- Links: GitHub repo | npm page | live demo | official website
- License: Apache License, Version 2.0
- Written in: Almost TypeScript
TinyMCE is an advanced open-source core-rich text editor that can be easily integrated into your React projects.
It is easy to configure the UI to match the layout design of your site, product, or application. Due to its flexibility, you can configure the editor with as much or as little functionality as you like, depending on your requirements.
With a ton of powerful plugins available, extending and adding additional functionality to TinyMCE is as simple as including a single line of code.
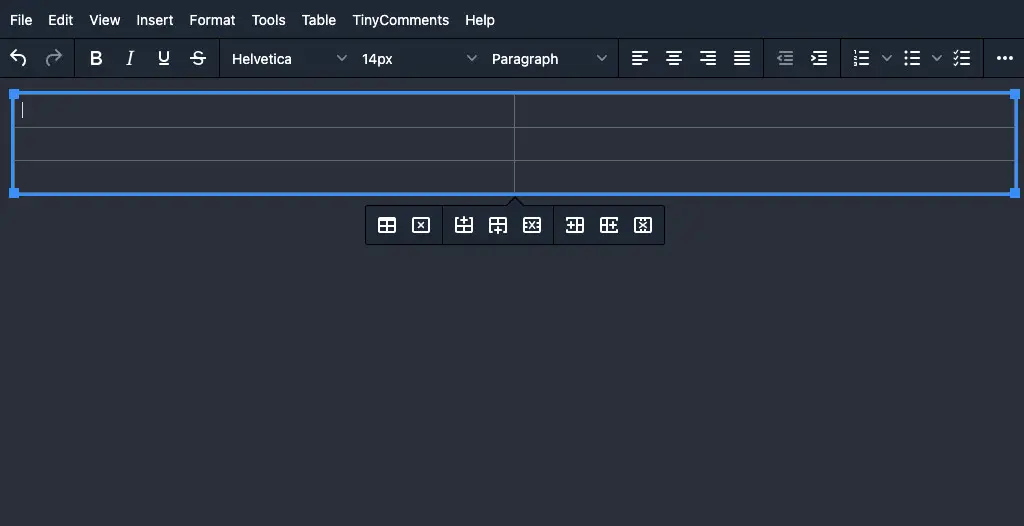
Sample use:
import React, { useRef } from 'react';
import { Editor } from '@tinymce/tinymce-react';
const App = () => {
const editorRef = useRef(null);
const log = () => {
if (editorRef.current) {
console.log(editorRef.current.getContent());
}
};
return (
<>
<Editor
onInit={(evt, editor) => editorRef.current = editor}
initialValue="<p>This is the initial content of the editor.</p>"
init={{
height: 500,
menubar: false,
plugins: [
'advlist autolink lists link image charmap print preview anchor',
'searchreplace visualblocks code fullscreen',
'insertdatetime media table paste code help wordcount'
],
toolbar: 'undo redo | formatselect | ' +
'bold italic backcolor | alignleft aligncenter ' +
'alignright alignjustify | bullist numlist outdent indent | ' +
'removeformat | help',
content_style: 'body { font-family:Helvetica,Arial,sans-serif; font-size:14px }'
}}
/>
<button onClick={log}>Log editor content</button>
</>
);
}
export default App;
Conclusion
We’ve gone over a collection of great, free, and open-source rich text editors that you can use in your React applications. Which one will you choose? Please let us know in the comment section.
If you’d like to explore more new and exciting things in React and front-end development, take a look at the following articles:
- React + TypeScript: Handling form onSubmit event
- How to fetch data from APIs with Axios and Hooks in React
- Most popular React Component UI Libraries
- Best open-source React form validation libraries
- React useReducer hook – Tutorial and Examples
- React + TypeScript: Multiple Dynamic Checkboxes
- React + TypeScript: Working with Props and Types of Props
You can also check our React topic page and React Native topic page for the latest tutorials and examples.
Thank you for sharing your expertise through this post. It has been incredibly beneficial and has expanded my knowledge on the subject. Your efforts in creating such a helpful resource are commendable!
i hope you feel good, stranger.
console.log(‘test’)
asdada
DraftJS is under maintenance mode and will be archived in Dec 2022. Facebook is replacing it with “Lexical”: https://github.com/facebook/lexical
ding a single line of code.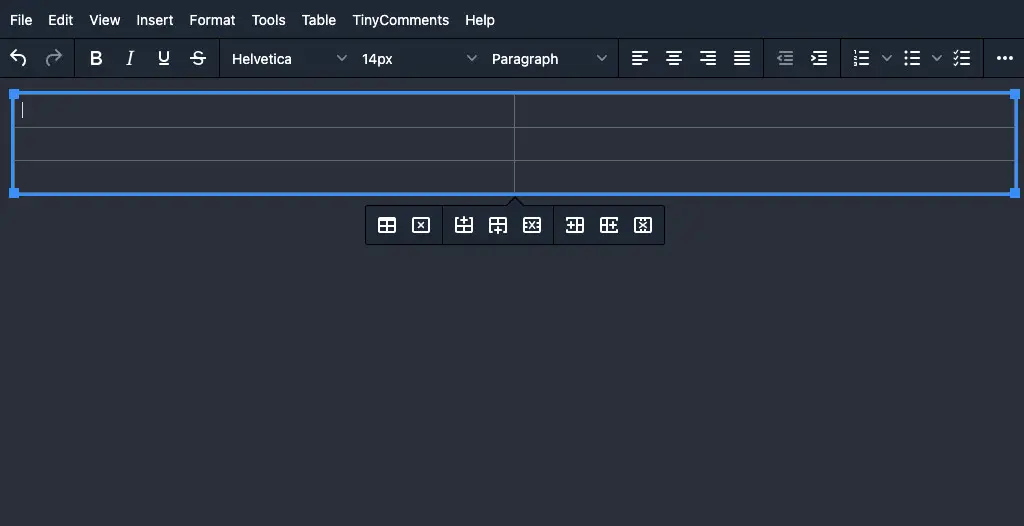
Sample use:
sadsad
My recommended ReactJS editor is Codelobster – http://www.codelobster.com/reactjs.html
This article is not about code editors but wysiwg editors to embed in react apps
Right. Obviously, most React developers use VS Code today.
Ty Editor.js
https://editorjs.io