This article will walk you through a list of the best open-source libraries for formatting and parsing date and time in React. Without any further ado, let’s discover the things that matter and are helpful.
date-fns
- GitHub stars: 34k+
- NPM weekly downloads: 18m – 22m
- License: MIT
- Written in: Javascript, TypeScript, Shell
- Links: GitHub repo | NPM page | Official website (documentation)
date-fns is a huge, comprehensive, and consistent toolset that provides more than 200 functions that can make your life much easier when dealing with date and time tasks. The package is built with pure functions and always returns a new date instance. It supports TypeScript, Flow, l18n, and many more features.

Installing:
npm i date-fns
Example:
import { format, formatDistance, formatRelative, subDays } from 'date-fns'
format(new Date(), "'Today is' eeee")
// Today is Friday
formatDistance(subDays(new Date(), 3), new Date(), { addSuffix: true })
// 3 days ago
formatRelative(subDays(new Date(), 3), new Date())
// last Tuesday at 7:26 p.m.
fecha
- GitHub stars: 2.2k+
- NPM weekly downloads: 8m – 10m
- License: MIT
- Written in: Javascript, TypeScript
- Links: GitHub repo | NPM page
fecha is a lightweight library with zero dependencies. It supports TypeScript out-of-the-box and provides a ton of intuitive functions.

Installing:
npm i fecha
Example:
import { format } from 'fecha';
type format = (date: Date, format?: string, i18n?: I18nSettings) => str;
ormat(new Date(2021, 10, 22), 'dddd MMMM Do, YYYY');
// 'Friday October 22th, 2021'
format(new Date(1988, 1, 2, 15, 23, 10, 350), 'YYYY-MM-DD hh:mm:ss.SSS A');
// '1988-01-02 03:23:10.350 PM
format(new Date(2021, 11, 10, 5, 30, 20), 'shortTime');
// '05:30'
moment
- GitHub stars: 48k+
- NPM weekly downloads: 20m – 25m
- License: MIT
- Written in: Javascript
- Links: GitHub repo | NPM page | Official website
moment.js used to be the most popular date-time library in the Javascript world. It’s now a legacy project and in maintenance mode, but still used by the vast majority of developers around the world.
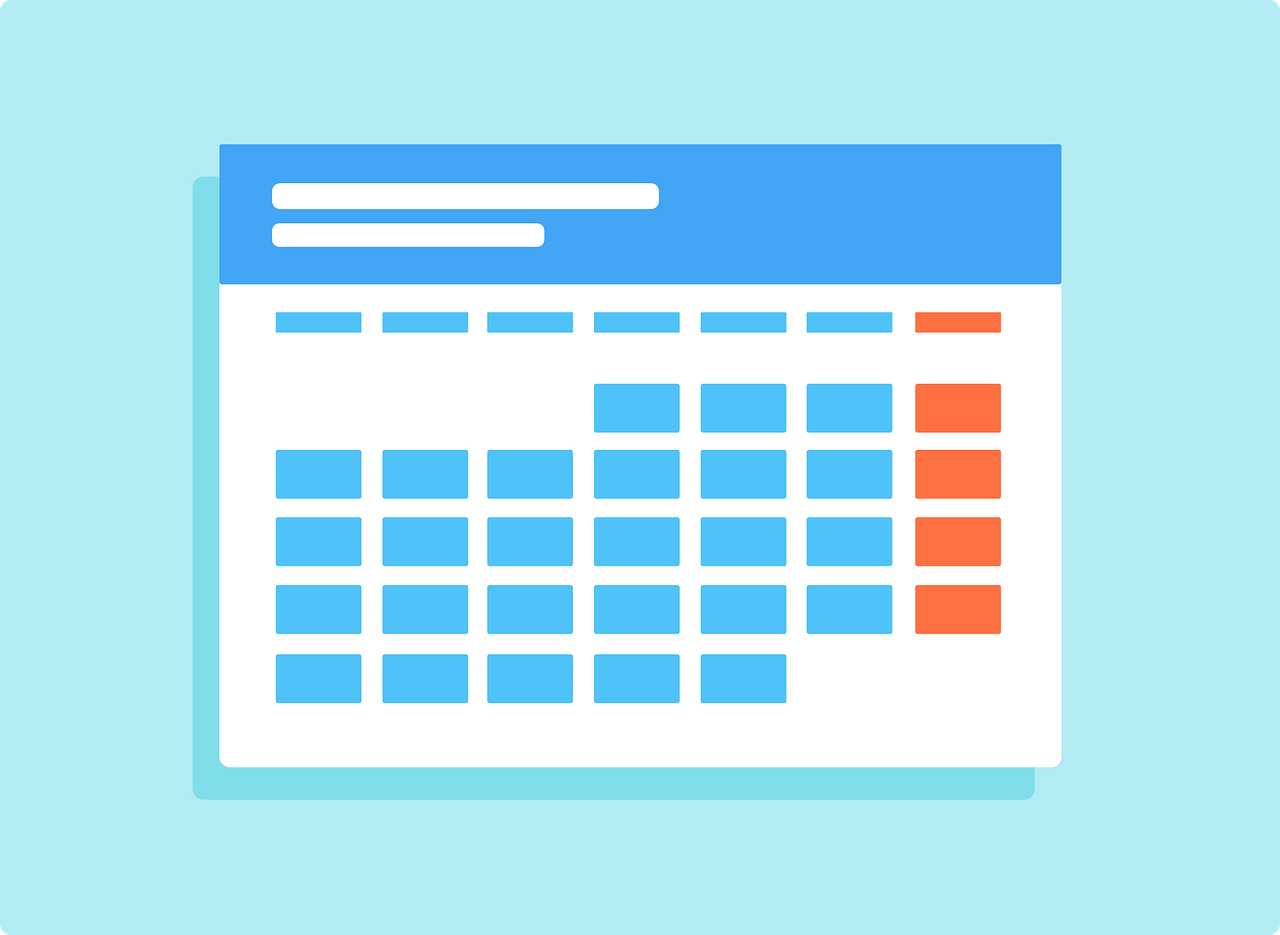
Installing:
npm i moment
Example:
import moment from 'moment';
moment().format('MMMM Do YYYY, h:mm:ss a');
// October 21st 2021, 3:11:36 pm
moment("20111031", "YYYYMMDD").fromNow();
// 10 years ago
moment().subtract(10, 'days').calendar();
// 10/11/2021
There are many large packages that were created to support moment.js or were inspired by this library:
- moment-timezone: This is an add-on for moment.js that supports the IANA Time zone.
- react-moment-prototypes: This is a React prototype validator to check if the passed prop is a moment.js construct
- react-moment: This one brings to the table a React component for moment.js, which is very handy and intuitive.
dayjs
- GitHub stars: 45.5k+
- NPM weekly downloads: 20m – 25m
- License: MIT
- Written in: Javascript
- Links: GitHub repo | NPM page | Official website
day.js is a fast-growing, minimal library for parsing, validating, manipulating, and displaying date and time with compatible API with the legacy moment.js.

Installing:
npm i dayjs
Example:
import dayjs from 'dayjs';
dayjs('2021-12-12');
dayjs().format('{YYYY} MM-DDTHH:mm:ss SSS [Z] A')
dayjs().set('month', 3).month()
luxon
- GitHub stars: 15k+
- NPM weekly downloads: 7m – 9m
- License: MIT
- Written in: Javascript
- Links: GitHub repo | NPM page | Official docs
Luxon is a modern, powerful, and friendly library for dealing with dates and times in Javascript with many features:
- Formatting and parsing datetimes, intervals, and durations
- Interval support
- Duration support
- Internationalization of strings using the Intl API
- Built-in handling of time zones

Installing:
npm i luxon
If you want to write in TypeScript, you need to add @types/luxon:
npm i @types/luxon -D
Example:
import { DateTime } from 'luxon';
DateTime.fromObject({year: 2022, month: 5, day: 15, hour: 17, minute: 36})
// 2022-05-15T17:36:00.000-05:00
DateTime.now().plus({minutes: 15, seconds: 8})
// DateTime 2022-10-21T15:55:45.660-05:00
DateTime.now().setLocale('zh').toLocaleString()
// 2022/10/21
DateTime.now().toLocaleString(DateTime.DATE_MED)
// Oct 21, 2022
date-time
- GitHub stars: 30+
- NPM weekly downloads: 700k – 1.2m
- License: MIT
- Written in: Javascript, TypeScript
- Links: GitHub repo | NPM page
date-time is a tiny library with an unpacked size is approximately 4.4 kB that can help you display dates and times in a pretty format.
Installing:
npm i date-time
Example:
import dateTime from 'date-time';
dateTime({date: new Date(1979, 2, 4, 10)});
// 1979-02-04 10:00:00
dateTime({showTimeZone: true});
// 2021-10-22 10:07:05 UTC-5
dateTime({local: false, showTimeZone: true});
// 2021-10-22 10:07:05 UTC-5
Conclusion
We’ve gone through a list that covered the best and most-loved open-source libraries that can help you a lot when working with date and time in React. Which one will you choose for your next (or current) project? If you’d like to explore more new and interesting things about modern React, take a look at the following articles:
- 2 Ways to Use Custom Port in React (create-react-app)
- 5 Best Open-Source HTTP Request Libraries for React
- React + TypeScript: Re-render a Component on Window Resize
- React + TypeScript: Handling onScroll event
- React + TypeScript: Multiple Dynamic Checkboxes
- How to implement tables in React Native
You can also check our React category page and React Native category page for the latest tutorials and examples.