There might be times when you need to render HTML content in a single-page app made with React. For instance, you have a blog or a news website that uses React for the front end and use a headless CMS for the back end. In this case, one of the most important tasks is to fetch data from the backend via REST API or GraphQL and then display it to your users.
This article walks you through 2 examples that use different techniques to render raw HTML in React.
Using dangerouslySetInnerHTML
In vanilla javascript, you have the innerHTML property to set or return the HTML content (inner HTML) of an element. In React, you can use dangerouslySetInnerHTML to let React know that the HTML inside of that component is not something it cares about.
Example
The code:
// App.js
// Kindacode.com
const rawHTML = `
<div>
<p>The <strong>rat</strong> hates the <strong>cat</strong></p>
<p><i>This is something special</i></p>
<div>
<img src="https://www.kindacode.com/wp-content/uploads/2021/06/cute-dog.jpeg"/>
</div>
<h1>H1</h1>
<h2>H2</h2>
<h3>H3</h3>
<h4>Just Another Heading</h4>
</div>
`;
const App = () => {
return (
<div style={container}>
<div dangerouslySetInnerHTML={{ __html: rawHTML }}></div>
</div>
);
};
export default App;
// Styling
const container = {
width: 500,
margin: '50px auto'
}
Output:
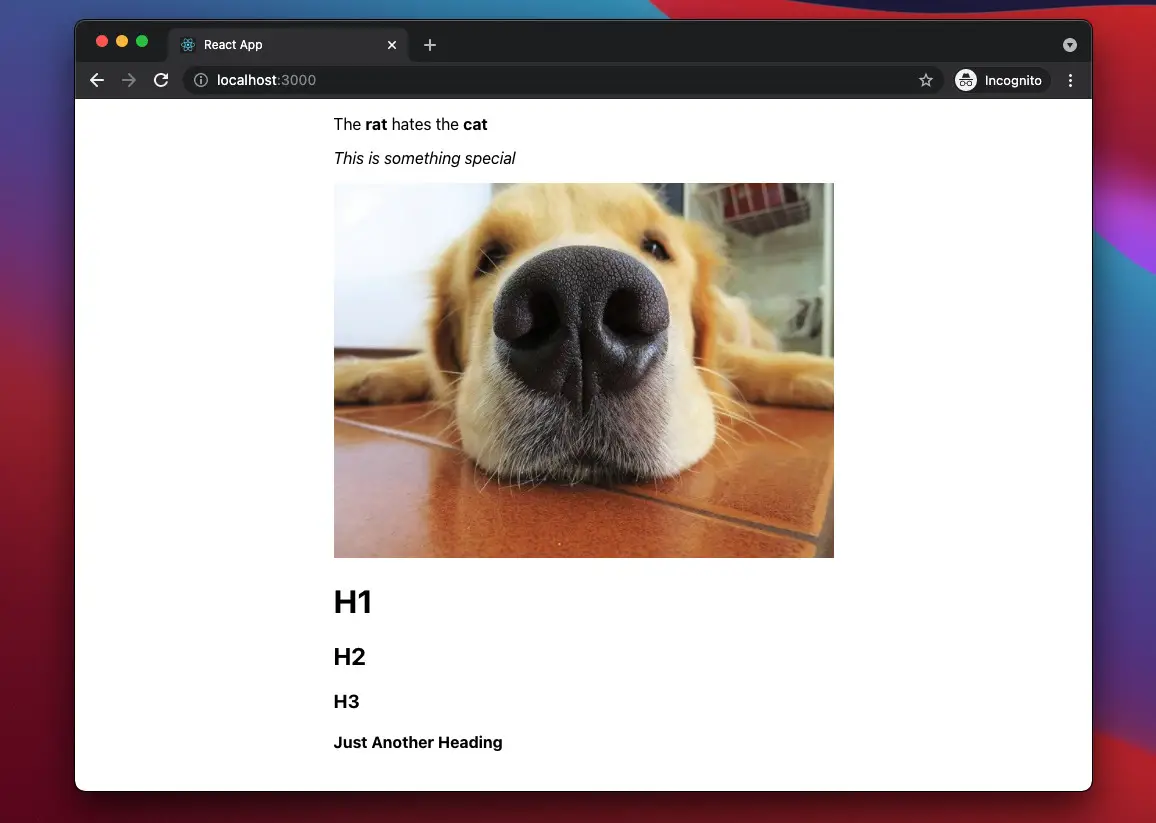
You can find more information about dangerouslySetInnerHTML in the React official docs.
Using a third-party library
If you have a strong reason not to use dangerouslySetInnerHTML, you can use a third-party library that doesn’t use dangerouslySetInnerHTML internally. There are several npm packages that meet this requirement, such as html-to-react or react-render-html.
The example below uses html-to-react. You can install it by running:
npm install html-to-react --save
Example
The code:
// App.js
// Kindacode.com
import { Parser } from 'html-to-react'
const rawHTML = `
<div>
<h1>The Second Example</h1>
<p>The <strong>rat</strong> hates the <strong>cat</strong></p>
<p><i>This is something special</i></p>
<hr/>
<div>
<img src="https://www.kindacode.com/wp-content/uploads/2021/06/pi-2.jpeg" width="500"/>
</div>
<hr/>
<h4>Just Another Heading</h4>
</div>
`;
const App = () => {
return (
<div style={container}>
{Parser().parse(rawHTML)}
</div>
);
};
export default App;
// Styling
const container = {
width: 500,
margin: 'auto'
};
Output:

Conclusion
You’ve learned a couple of different approaches to rendering raw HTML in JSX and React. If you’d like to explore more new and interesting things about modern frontend development, take a look at the following articles:
- React + TypeScript: Handling onFocus and onBlur events
- React & TypeScript: Using useRef hook example
- Most popular React Component UI Libraries
- How to fetch data from APIs with Axios and Hooks in React
- React useReducer hook – Tutorial and Examples
- React: Create an Animated Side Navigation from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.