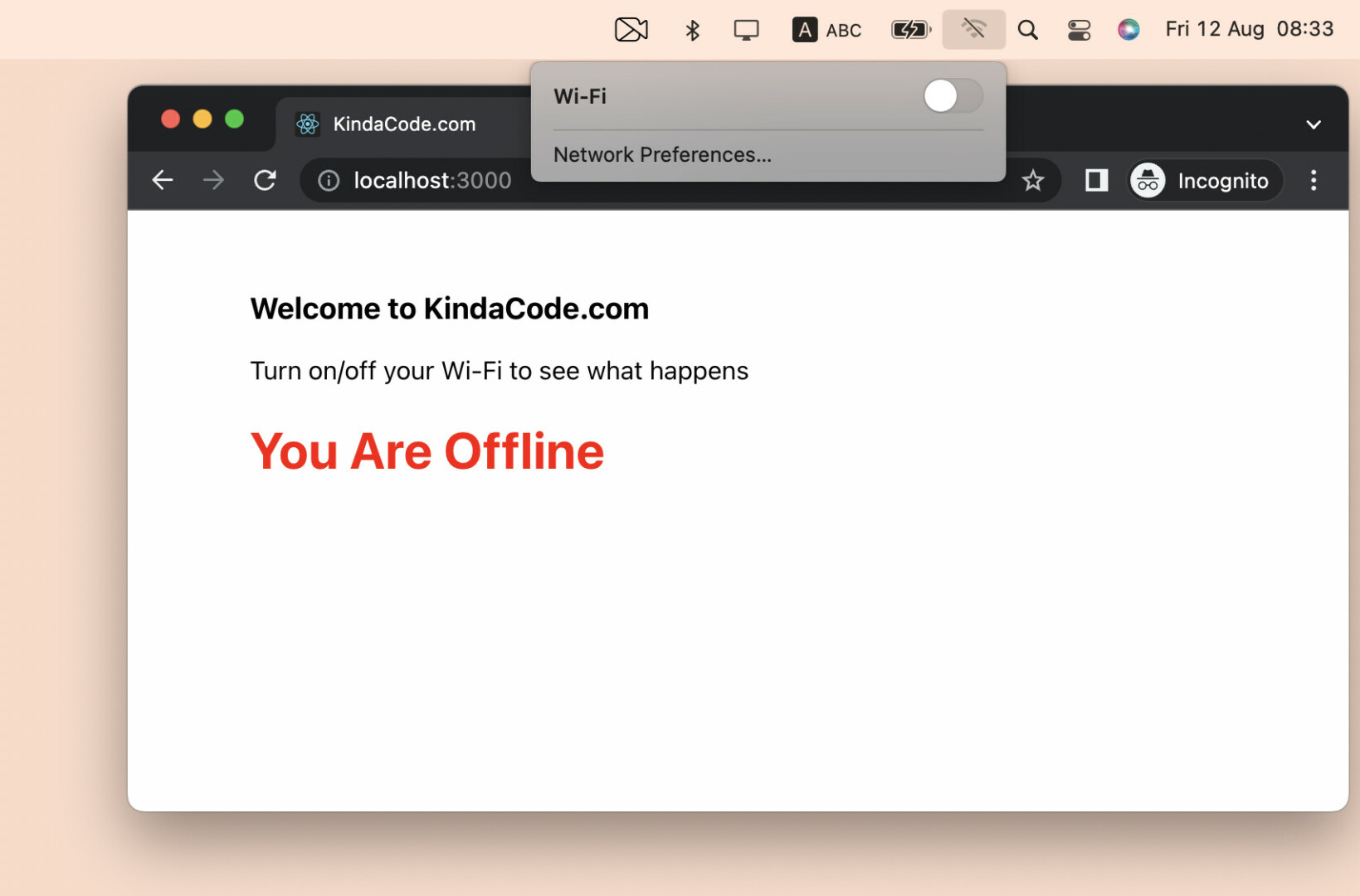
This succinct, practical article shows you how to check the internet connection in React by using hooks. No third-party libraries are required.
Table of Contents
What Is The Point?
In Javascript, there is an object called navigator that can tell us about the network status. It can be accessed with window.navigator, or just navigator (you don’t need to import anything). The navigator.onLine property will be true if there is an internet connection. Otherwise, it is false. There is a strange and interesting thing here is that onLine has a capital L, not all lowercase letters:
navigator.onLine // true or false
In React, we can rerender the user interface every time the network status changes by using the useState and useEffect hooks, like so:
// Online state
const [isOnline, setIsOnline] = useState(navigator.onLine);
useEffect(() => {
// Update network status
const handleStatusChange = () => {
setIsOnline(navigator.onLine);
};
// Listen to the online status
window.addEventListener('online', handleStatusChange);
// Listen to the offline status
window.addEventListener('offline', handleStatusChange);
// Specify how to clean up after this effect for performance improvment
return () => {
window.removeEventListener('online', handleStatusChange);
window.removeEventListener('offline', handleStatusChange);
};
}, [isOnline]);
For more clarity, see the concrete, working example below.
Full Example
App Overview
The GIF below clearly depicts what we’re going to make. When the Wi-Fi is On, it will display the blue text You Are Online. When the Wi-Fi if Off, it shows the red text You Are Offline.
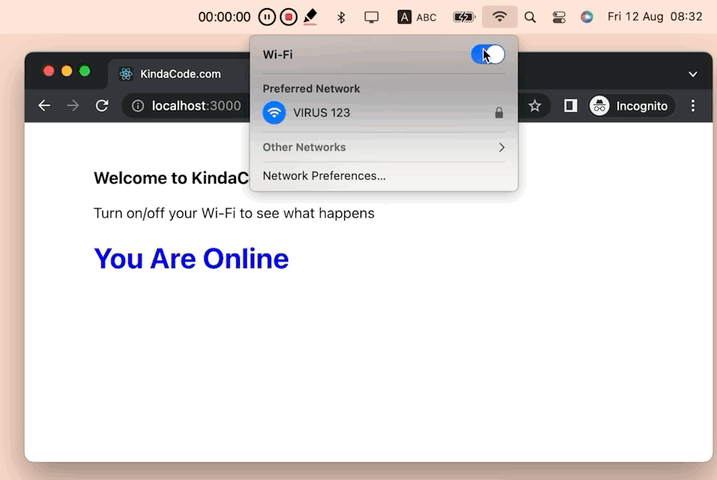
The Code
1. The complete JS code (with explanations):
// KindaCode.com
// src/App.js
import { useState, useEffect } from 'react';
import './App.css';
function App() {
// Online state
const [isOnline, setIsOnline] = useState(navigator.onLine);
useEffect(() => {
// Update network status
const handleStatusChange = () => {
setIsOnline(navigator.onLine);
};
// Listen to the online status
window.addEventListener('online', handleStatusChange);
// Listen to the offline status
window.addEventListener('offline', handleStatusChange);
// Specify how to clean up after this effect for performance improvment
return () => {
window.removeEventListener('online', handleStatusChange);
window.removeEventListener('offline', handleStatusChange);
};
}, [isOnline]);
return (
<div className='container'>
<h3>Welcome to KindaCode.com</h3>
<p>Turn on/off your Wi-Fi to see what happens</p>
{isOnline ? (
<h1 className='online'>You Are Online</h1>
) : (
<h1 className='offline'>You Are Offline</h1>
)}
</div>
);
}
export default App;
2. And here is the CSS code:
/* KindaCode.com */
/* src/App.cs */
.container {
width: 80%;
margin: 50px auto;
}
.online {
color: blue;
}
.offline {
color: red;
}
Conclusion
Congratulation! You made it, a simple and intuitive React app that can detect internet status changes. You can implement this approach in many situations, such as a chat app or a gamelike app.
React is awesome, and mastering it requires relentless learning. Continue entrenching your skills and gaining more experience by taking a look at the following articles:
- React: How to Detect Caps Lock is On
- React: How to Create an Image Carousel from Scratch
- React: How to Create a Responsive Navbar from Scratch
- React + TypeScript: Making a Reading Progress Indicator
- React: Get the Position (X & Y) of an Element
- How to set a gradient background in React Native
You can also check our React category page and React Native category page for the latest tutorials and examples.