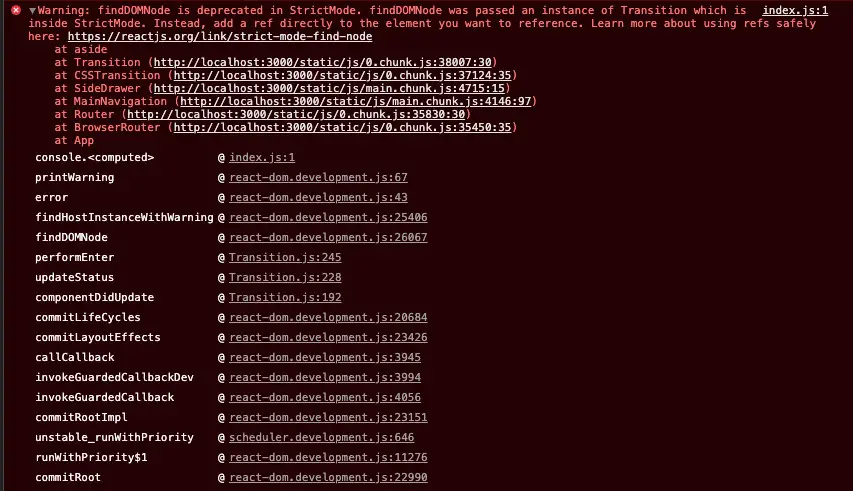
The Problem
When working with React.js, you will probably come across an annoying warning:
Warning: findDOMNode is deprecated in StrictMode. findDOMNode was passed an instance of Transition which is inside StrictMode. Instead, add a ref directly to the element you want to reference. Learn more about using refs safely here: https://reactjs.org/link/strict-mode-find-node
The Root Of The Problem
If you follow the link provided in the warning, you’ll see some helpful information from the React team:
React used to support findDOMNode to search the tree for a DOM node given a class instance. Normally you don’t need this because you can attach a ref directly to a DOM node.
But if you didn’t use findDOMNode, why does this still happen?
In many cases, the culprit that causes the annoying warning is not you or your code but some components from a third-party library used in your project. These libraries are not faulty, but simply they have not been updated to the newer version in time. Among these, there are very famous and popular libraries like React Bootstrap, React Transition Group, React Draggable, etc.

In the future, when the teams behind these libraries make changes, everything will be resolved automatically. No action is required from you at this time. However, if you find these warnings too annoying and annoying, you can resolve them using one of the following solutions listed below.
Solutions
1. Remove React.StrictMode in index.js
This method is simple and you only need to do it once in a single place. Go to your ./src/index.js file and change it from this:
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
to this:
ReactDOM.render(
<App />
document.getElementById('root')
);
Note that StrictMode is a tool for highlighting potential problems in a React application. It activates additional checks and warnings such as:
- Identifying components with unsafe lifecycles
- Warning about legacy string ref API usage
- Warning about deprecated findDOMNode usage
- Detecting unexpected side effects
- Detecting legacy context API
Strict mode checks are run in development mode only; they do not impact the production build.
2. Using Ref
This method is different for each library and will take more time and effort than the first method. However, the upside is that you can still get other warnings from the strict mode for debugging.
For example, with the React Draggable library:
const MyComponent = props => {
const nodeRef = React.useRef(null);
return (
<Draggable nodeRef={nodeRef}>
<div ref={nodeRef}>Example Target</div>
</Draggable>
);
}
Final Words
In this article, we had a look at the React findDOMNode warning and went over some approaches to get rid of it. If you’d like to learn more new and fascinating things about modern React, take a look at the following articles:
- React + TypeScript: Working with Props and Types of Props
- React + TypeScript: onMouseOver & onMouseOut events
- React useReducer hook – Tutorial and Examples
- How to Create a Scroll To Top Button in React
- React: Show Image Preview before Uploading
- React + TypeScript: Making a Custom Context Menu
- React: How to Upload Multiple Files with Axios
You can also check our React category page and React Native category page for more tutorials and examples.
good
Perfect !!
Using ref works perfectly! Thank you for the help
Thank you!!! Exactly the issue I had with my draggable
thnks so much, u r the best
You’re welcome
thanks
nice its working to remove react strict mode in index.js
Yes. It’s a trade-off.