This article walks you through a list of the most popular and modern approaches to persist data locally in React. Some of them are easy to use and great for a small amount of simple data, while others are totally fit for a large amount of complex structured data.
A Brief Overview
There are many reasons why you may need to save data offline in React:
- You want to save some user authentication stuff like JSON web tokens, session IDs, etc.
- You want to persist some user personal settings like dark/light mode, font size, etc.
- You want your web app to run well even with a slow or frequent interruption internet connection.
- You’re developing a small tool like an online calculator, a length converter, or something like that with React but don’t want to build a backend. Calculation history and recent results will be saved right on the user’s browser.
And countless unforeseen situations that you may face in the path of your career.
IndexedDB: The standard client-side database
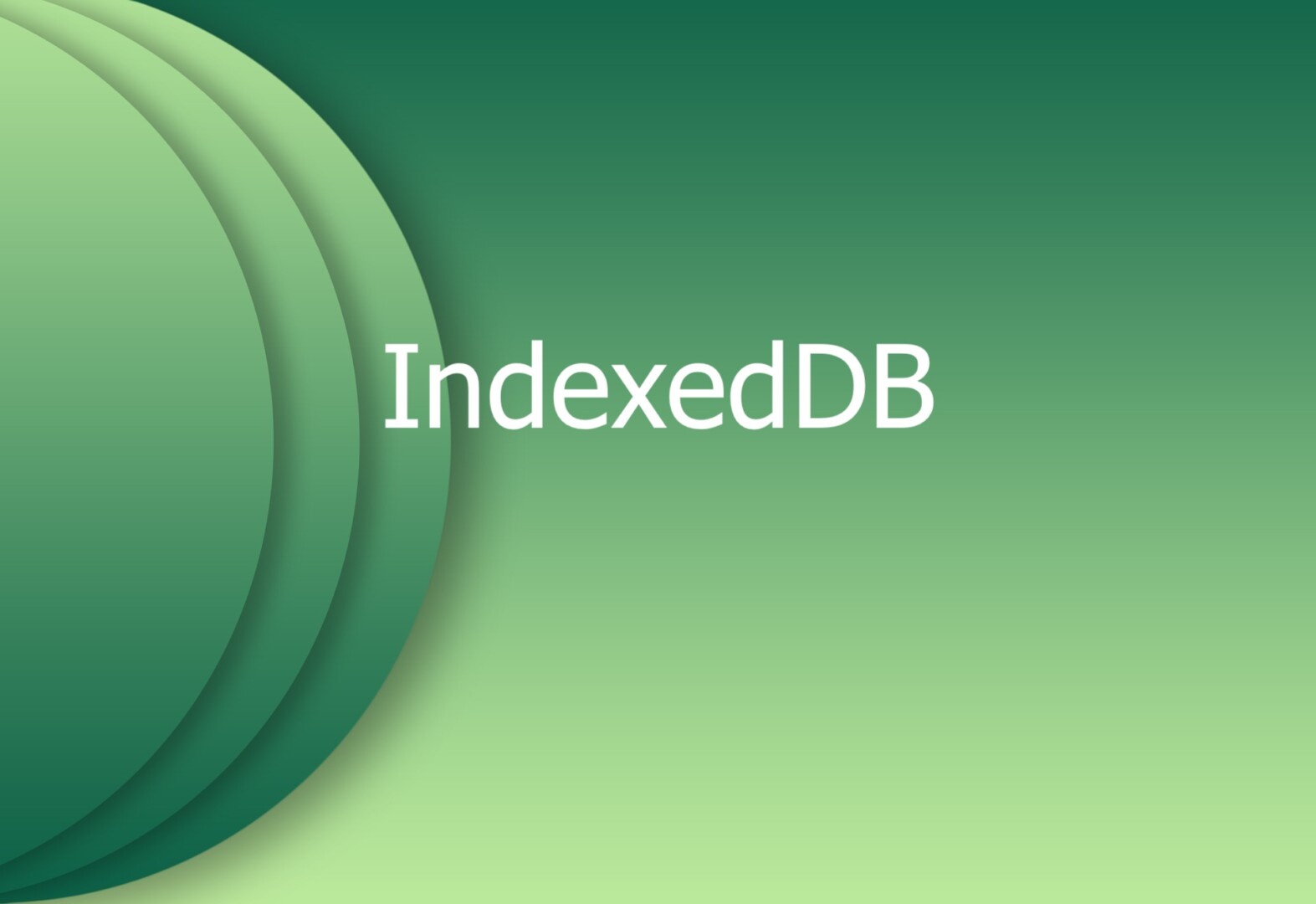
IndexedDB is a NoSQL database technology supported by every modern web browser (Google Chrome 11+, IE 10+, Firefox 4+, Safari 8+, Microsoft Edge 12+). The capacity of IndexedDB is very huge and can be used for a wide range of purposes from small to large, including caching all contents of a web app for offline usage.
In React, you can work with IndexedDB much easier by using an open-source npm package like idb (5m – 8m weekly downloads) or dexie (350k – 500k weekly downloads).
Let’s see a quick example that demonstrates how to make use of the idb package:
// import
import { openDB } from 'idb';
// working with IndexedDB asynchronously with async/await
const db = await openDB('database name');
const tx = db.transaction('keyval', 'readwrite');
const store = tx.objectStore('keyval');
const val = (await store.get('counter')) || 0;
await store.put(val + 1, 'counter');
await tx.done;
LocalStorage
Every modern browser supports web storage (even Internet Explorer 8). Web storage provides methods and protocols for the long-term storing of client-side data. The maximum size of local storage for a single web app is about 5MB (this can be changed by the user).
The upside of local storage is its simplicity. On the downside, local storage is synchronous and can only contain string (to store an object, you have to call JSON.stringify).
In React, local storage is the best suited for lightweight data, such as authentication credentials and user settings. There are several npm packages that are based on local storage:
- store2 (4m – 5.5m weekly downloads)
- localForage (2m – 3.5m weekly downloads)
- localStorage (60k – 80k weekly downloads)
For example, you can use the store2 package like so:
import store from 'store2';
store.set(key, data[, overwrite]);
// store({key: data, key2: data});
store.setAll(data[, overwrite]);
// get data
store.getAll([fillObj]);
// clear the data
store.clear();
// check if a given key exists
store.has(key);
// delete key and its data, then returns the data or alt, if none
store.remove(key[, alt]);
// concats, merges, or adds new value into existing one
store.add(key, data[, replacer]);
Cookies
Cookies were born early and have accompanied websites for decades. You can use them to store small pieces of information (the limit is usually 4kB). In the real world, it is used in most authentication-related cases (especially the session-cookie mechanism).
Cookies are synchronous and sent with every HTTP request, so you should only store a small amount of data with them. Otherwise, the size of every web request will be significantly increased.
In React, you can easily handle cookies with the help of an npm package, such as:
- js-cookie (8m+ weekly downloads)
- react-cookie (500k+ weekly downloads)
PouchDB
PouchDB is an open-source JavaScript database inspired by Apache CouchDB that is designed to run well within the browser. It can help you sync data between a local database on the user’s computer to an online database that lives on your server.
In React, you can make use of PouchDB with the help of the pouchdb package (40k+ weekly downloads):
npm i pouchdb
Sample usage:
const db = new PouchDB('dbname');
db.put({
title: 'Hello There',
description: 'How is it going?'
});
db.changes().on('change', function() {
console.log('It changes');
});
// sync data to an online server
db.replicate.to('https://your-database-address');
You can find more information on the official website.
Cache API
The Cache API can be used for storing and retrieving network requests and responses. The data can be text-based as well as file-based contents, and any kind of data can be transferred over HTTP.
You can use the cache API with the global caches property (supported on every modern browser). You can perform a simple check like so:
const isAvailable = 'caches' in self;
console.log(isAvailable);
Some common methods:
- Cache.add(request)
- Cache.put(request, response)
- Cache.delete(request, options)
You can find more great details on MDN.
Other Solutions
File System API
The File System API provides methods for reading and writing files to a sandboxed file system. However, only some browsers support it. Therefore, you shouldn’t use it.
WebSQL (depreciated)
WebSQL was created with the intention of helping developers build client-side relational databases. However, at the present time, it has been discontinued by most browsers and you should not use it. Instead, consider using IndexedDB, localStorage, or cookies.
Conclusion
We’ve discovered various kinds of implementations to persist data on the client side in React. Choose from them the ones that suit your needs and taste.
The world of React is vast and relentlessly evolving. New features and improvements are constantly being added. If you would like to explore more interesting stuff about this awesome frontend technology, take a look at the following articles:
- React: How to Detect a Click Outside/Inside a Component
- React: Check if the user device is in dark mode/light mode
- How to Use Styled JSX in React: A Deep Dive
- How to Use Tailwind CSS in React
- React Router: Redirecting with the Navigate component
- React Router: useParams & useSearchParams Hooks
You can also check our React category page and React Native category page for the latest tutorials and examples.