In simple words, SafeArea is a widget that automatically adds padding needed to prevent its descendants from being covered by notches, the system status bar, rounded corners… or other similar creative physical features on the device screen.
Constructor:
SafeArea({
Key? key,
bool left = true,
bool top = true,
bool right = true,
bool bottom = true,
EdgeInsets minimum = EdgeInsets.zero,
bool maintainBottomViewPadding = false,
required Widget child
})
Where:
- left/ top / right / bottom: Whether to avoid system intrusions on the left / top / right / bottom side of the screen
- minimum: The minimum padding to apply
- maintainBottomViewPadding: Determines whether the SafeArea should maintain the bottom MediaQueryData.viewPadding instead of the bottom MediaQueryData.padding
- child: The child widget of SafeArea
Example
Without SafeArea
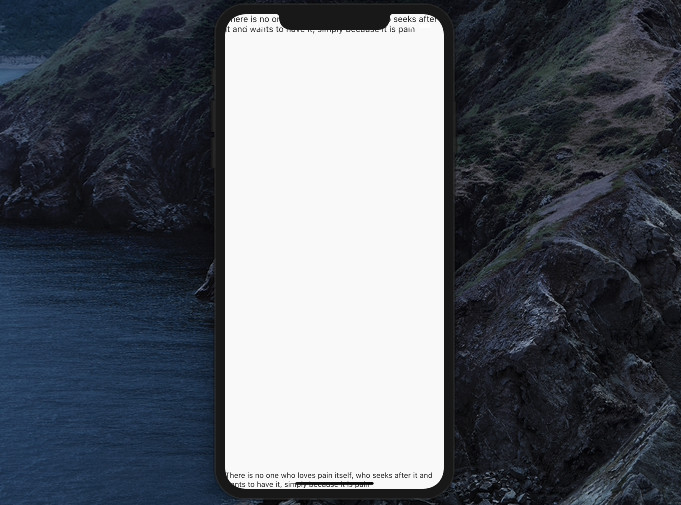
With SafeArea

The complete source code that produces the sample app that shown in the second screenshot:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: const [
Text(
'There is no one who loves pain itself, who seeks after it and wants to have it, simply because it is pain',
style: TextStyle(fontSize: 16),
),
Text(
'There is no one who loves pain itself, who seeks after it and wants to have it, simply because it is pain')
],
),
),
);
}
}
Further reading:
- Flutter: Create a Password Strength Checker from Scratch
- Flutter: How to Draw a Heart with CustomPaint
- Implementing Tooltips in Flutter
- Adding a Border to Text in Flutter
- Flutter: How to Colorize Debug Console Logs
- Flutter: How to Make Spinning Animation without Plugins
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.