
This practical article shows you how to create a word counter in React (in addition, we’re going to add a character counter, since it doesn’t take much effort).
A Quick Note
There is a common pitfall that some developers fall into when counting words in a given string. If you do as the code snippet below, it might produce inaccurate results in many cases:
const text = /* ... */;
// create array of words
const words = text.split(' ');
const wordCount = words.length;
// text = 'abc ' -> wordCount = 2
// text = '' -> wordCount = 1
Below is a proper approach to determining the number of words in a given string:
const text = /* ... */;
// create array of words
const words = text.split(' ');
let wordCount = 0;
words.forEach((word) => {
if (word.trim() !== '') {
wordCount++;
}
});
Counting characters in a given string is simple:
const text = /* ... */
const charCount = text.length;
Full Example
App Preview
The small demo we’re going to make has a textarea. When you type some text into this textarea, the number of words and the number of characters (including whitespace) will be displayed instantly:
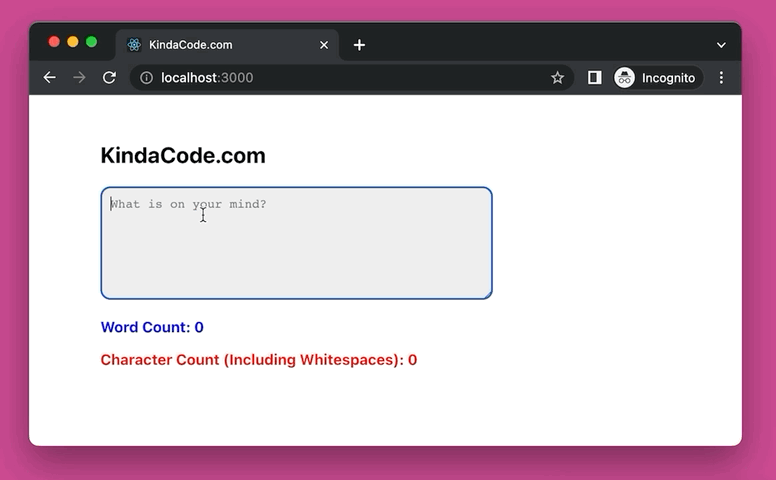
The Code
1. To make sure we have the same starting point, let’s initialize a brand new React project:
npx create-react-app kindacode-example
2. The complete code in App.js (with explanations):
// KindaCode.com
// src/App.js
import { useState, useEffect } from 'react';
import './App.css';
function App() {
// the text entered by the user
const [text, setText] = useState('');
// word count
const [wordCount, setWordCount] = useState(0);
// character count
const [charCount, setCharCount] = useState(0);
const changeHandler = (event) => {
setText(event.target.value);
};
useEffect(() => {
// array of words
const words = text.split(' ');
// update word count
let wordCount = 0;
words.forEach((word) => {
if (word.trim() !== '') {
wordCount++;
}
});
setWordCount(wordCount);
// update char count (including whitespaces)
setCharCount(text.length);
}, [text]);
return (
<div className='container'>
<h2>KindaCode.com</h2>
<div>
<textarea
value={text}
onChange={changeHandler}
placeholder='What is on your mind?'
></textarea>
<div>
<p className='word-count'>Word Count: {wordCount}</p>
<p className='char-count'>
Character Count (Including Whitespaces): {charCount}
</p>
</div>
</div>
</div>
);
}
export default App;
3. And here’s all the CSS code in App.css:
.container {
width: 80%;
margin: 50px auto;
}
textarea {
width: 400px;
height: 100px;
background: #eee;
padding: 10px;
border-radius: 10px;
}
.word-count {
color: blue;
font-weight: bold;
}
.char-count {
color: red;
font-weight: bold;
}
4. Run the project in the development mode:
npm start
And go to http://localhost:3000 to check your work.
Conclusion
We’ve implemented a word counter and a character counter with the useState and useEffect hooks. I hope you like the example. Try to modify the code, change some things, and see what happens.
The world of front-end development evolves so fast. Continue exploring more new and interesting stuff by taking a look at the following articles:
- React: Check if user device is in dark mode/light mode
- React + MUI: Create Dark/Light Theme Toggle
- React + TypeScript: Making a Custom Context Menu
- React: How to Check Internet Connection (Online/Offline)
- React + TypeScript: Handling Keyboard Events
- How to Implement a Picker in React Native
You can also check our React category page and React Native category page for the latest tutorials and examples.
thanks, it was very useful for me