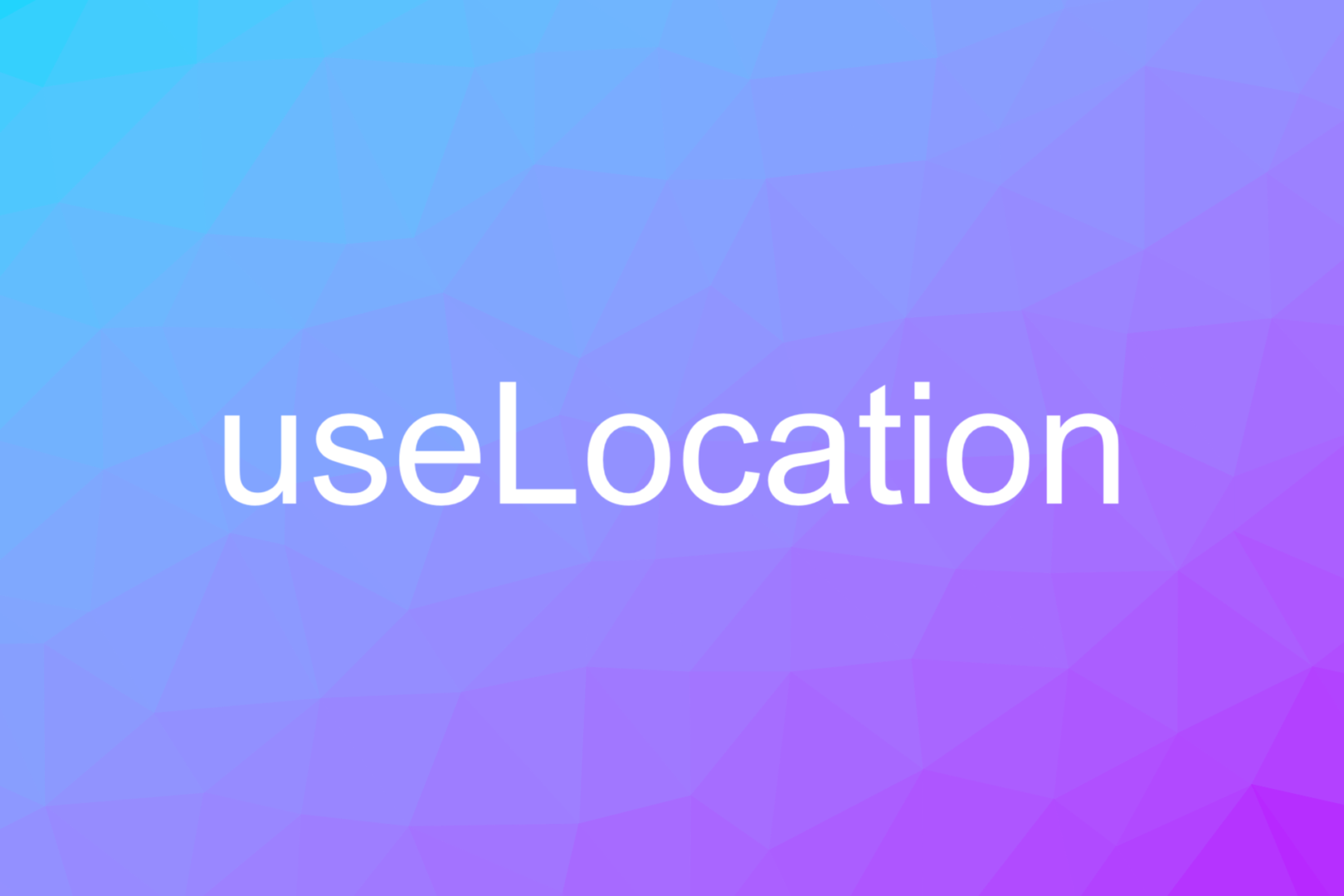
It’s 2023, and React Router is the most popular library for routing and navigation in React. At its heart, React Router is a state container for the current location or URL. It keeps track of the location and renders different routes as it changes, and it also gives you tools to update the location using links and the history API.
With the release of React Router 6, there are some useful hooks that you can use in React applications. In this article, we will take a look at the useLocation hook and walk through a few examples of using it.
Note: To use the useLocation hook, you need React 16.8+ and react-router-dom 6.x or at least 5.x (this article will show you 2 code snippets corresponding to 2 different versions of React Router).
What is the point of the useLocation hook
The useLocation hook is a function that returns the location object that contains information about the current URL. Whenever the URL changes, a new location object will be returned.
Location object properties:
- hash: the anchor part (#)
- pathname: the path name
- search: the query string part
- state
- key
Example code:
import React from 'react';
import { useLocation } from 'react-router-dom';
const Example = props => {
const location = useLocation();
console.log(location);
// ...
};
Real-world use cases
The useLocation hook is useful in many cases when you want to trigger a function based on a change of the URL. In general, it is used in conjunction with the useEffect hook provided by React.
For example, the pages of your React app are taller than the height of the screen (100 vh), and you want to scroll to the top of the page every time the user switches the route, like this:
To archive this purpose, you can do as shown below:
Using React Router 6.x
// App.js
import React, { useEffect } from "react";
import {
BrowserRouter as Router,
Route,
Routes,
Link,
useLocation,
} from "react-router-dom";
// Create a custom hook that uses both useLocation and useEffect
const useScrollToTop = () => {
const location = useLocation();
useEffect(() => {
window.scrollTo({ top: 0 });
// scroll to the top of the browser window when changing route
// the window object is a normal DOM object and is safe to use in React.
}, [location]);
};
// This is corresponding to "/" route
const Home = (props) => {
useScrollToTop();
return (
<>
<h1>Home</h1>
<hr />
<p style={{ marginTop: "150vh" }}>
<Link to="/contact">Go to contact page</Link>
</p>
</>
);
};
// This is corresponding to "/contact" route
const Contact = (props) => {
useScrollToTop();
return (
<>
<h1>Contact</h1>
<hr />
<p style={{ marginTop: "150vh" }}>
<Link to="/">Go to homepage</Link>
</p>
</>
);
};
// The root component
const App = () => {
return (
<div style={{ padding: 50 }}>
<Router>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/contact" element={<Contact />}/>
</Routes>
</Router>
</div>
);
};
export default App;
Using React Router 5.x
// App.js
import React, { useEffect } from 'react';
import {
BrowserRouter as Router,
Route,
Switch,
Link,
useLocation,
} from 'react-router-dom';
// Create a custom hook that uses both useLocation and useEffect
const useScrollToTop = () => {
const location = useLocation();
useEffect(() => {
window.scrollTo({ top: 0 });
// scroll to the top of the browser window when changing route
// the window object is a normal DOM object and is safe to use in React.
}, [location]);
};
// This is corresponding to "/" route
const Home = (props) => {
useScrollToTop();
return (
<>
<h1>Home</h1>
<hr />
<p style={{ marginTop: '150vh' }}>
<Link to="/contact">Go to contact page</Link>
</p>
</>
);
};
// This is corresponding to "/contact" route
const Contact = (props) => {
useScrollToTop();
return (
<>
<h1>Contact</h1>
<hr />
<p style={{ marginTop: '150vh' }}>
<Link to="/">Go to homepage</Link>
</p>
</>
);
};
// The root component
const App = () => {
return (
<div style={{ padding: 50 }}>
<Router>
<Switch>
<Route path="/" exact>
<Home />
</Route>
<Route path="/contact" exact>
<Contact />
</Route>
</Switch>
</Router>
</div>
);
};
export default App;
Conclusion
We’ve covered the fundamentals of the useLocation hook and gone over a complete example of using it in a React application. If you’d like to explore more new and interesting things about modern React and React Native, take a look at the following articles:
- React + TypeScript: Handling form onSubmit event
- React + TypeScript: Drag and Drop Example
- React + TypeScript: Re-render a Component on Window Resize
- React + TypeScript: onMouseOver & onMouseOut events
- React: Using inline styles with the calc() function
- React Router 6: Passing Data (States) through Links
You can also check out our React topic page and React Native topic page for additional example projects and resources.