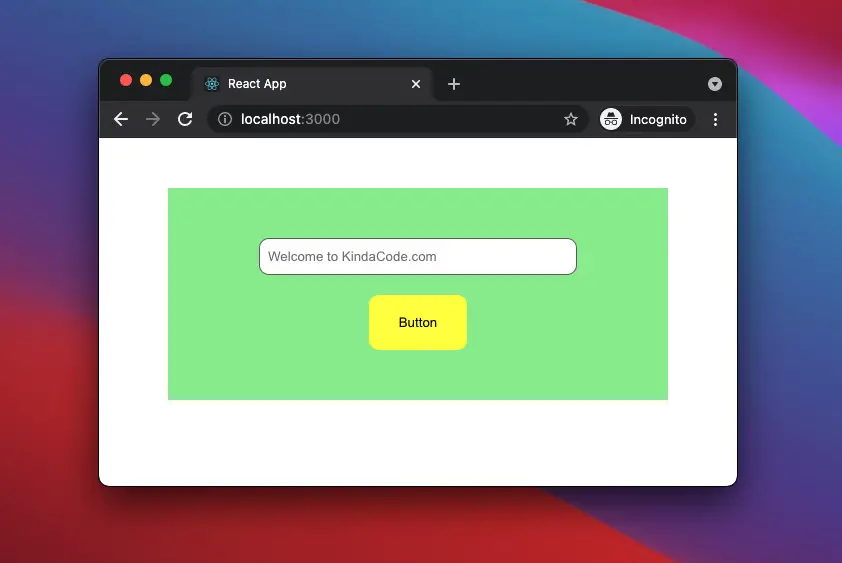
This article is about the onMouseOver and onMouseOut events in a React project written in TypeScript. We’ll go through the fundamentals of these events then examine a complete example of using them in practice.
Table of Contents
Overview
The onMouseOver event in React occurs when the mouse pointer is moved onto an element (it can be a div, a button, an input, a textarea, etc). The event handler function will be fired and we can execute our logic there.
The onMouseOut event in React occurs when the mouse pointer is moved out of an element. This event and the onMouseOver event are a close pair and often used together.
The event parameter of the handler function has the type as shown below:
React.MouseEvent<HTML[element-name]Element>
// Some common use cases
React.MouseEvent<HTMLDivElement>
event: React.MouseEvent<HTMLInputElement>
event: React.MouseEvent<HTMLHeadingElement>
For more clarity, see the example below.
Example
Preview
The small demo project we are going to build handles the onMouseOver and onMouseOut events on 3 common elements: div, input, and button:
- When the mouse pointer moves over the box, the background color will change from green to light blue.
- When the mouse pointer moves over the text input, the background color also changes
- When the mouse pointer moves over the button, the background color and the border style change.
Elements will return to their original state as the mouse pointer exits them.
The Code
1. Create a new React project by performing the following command:
npx create-react-app kindacode_example --template typescript
2. The full source code in src/App.tsx with explanations:
// App.tsx
// Kindacode.com
import React from "react";
const App = () => {
// This function will be triggered when the mouse pointer is over the box
const boxMouseOverHandler = (event: React.MouseEvent<HTMLDivElement>) => {
const box: HTMLDivElement = event.currentTarget;
box.style.backgroundColor = "lightblue";
};
// This function will be triggered when the mouse pointer is moving out the box
const boxMouseOutHandler = (event: React.MouseEvent<HTMLDivElement>) => {
const box: HTMLDivElement = event.currentTarget;
box.style.backgroundColor = "lightgreen";
};
// This function will be triggered when the mouse pointer is over the input
const inputMouseOverHandler = (event: React.MouseEvent<HTMLInputElement>) => {
const input: HTMLInputElement = event.currentTarget;
input.style.backgroundColor = "lime";
};
// This function will be triggered when the mouse pointer is moving out of the input
const inputMouseOutHandler = (event: React.MouseEvent<HTMLInputElement>) => {
const input: HTMLInputElement = event.currentTarget;
input.style.backgroundColor = "white";
};
// This function will be triggered when the mouse pointer is over the button
const buttonMouseOverHandler = (
event: React.MouseEvent<HTMLButtonElement>
) => {
const btn: HTMLButtonElement = event.currentTarget;
btn.style.border = "3px solid red";
btn.style.backgroundColor = "orange";
};
// This function will be triggered when the mouse pointer is moving out of the button
const buttonMouseOutHandler = (
event: React.MouseEvent<HTMLButtonElement>
) => {
const btn: HTMLButtonElement = event.currentTarget;
btn.style.border = "none";
btn.style.backgroundColor = "yellow";
};
return (
<div
style={styles.box}
onMouseOver={boxMouseOverHandler}
onMouseOut={boxMouseOutHandler}
>
<input
onMouseOver={inputMouseOverHandler}
onMouseOut={inputMouseOutHandler}
style={styles.input}
placeholder="Welcome to KindaCode.com"
/>
<button
onMouseOver={buttonMouseOverHandler}
onMouseOut={buttonMouseOutHandler}
style={styles.button}
>
Button
</button>
</div>
);
};
// Styling
const styles: { [key: string]: React.CSSProperties } = {
box: {
margin: "50px auto",
width: 400,
padding: 50,
backgroundColor: "lightgreen",
display: "flex",
flexDirection: "column",
justifyContent: "center",
alignItems: "center",
},
input: {
width: 300,
padding: "10px 8px",
border: "1px solid #666",
borderRadius: 10,
},
button: {
marginTop: 20,
padding: "20px 30px",
border: "none",
backgroundColor: "yellow",
cursor: "pointer",
borderRadius: 10,
},
};
export default App;
Conclusion
You’ve learned how to use the onMouseOver and onMouseOut events in a TypeScript React project. This knowledge might be very useful when you want to improve your user experience or add some fancy effects to your app. If you would like to explore more new and interesting stuff in modern React and frontend development, take a look at the following articles:
- React + TypeScript: Image onLoad & onError Events
- React + TypeScript: Handling onClick event
- React + TypeScript: Create an Autosize Textarea from scratch
- 5 best open-source WYSIWYG editors for React
- React + TypeScript: Multiple Dynamic Checkboxes
- React + TypeScript: Multiple Select example
You can also check our React category page and React Native category page for the latest tutorials and examples.