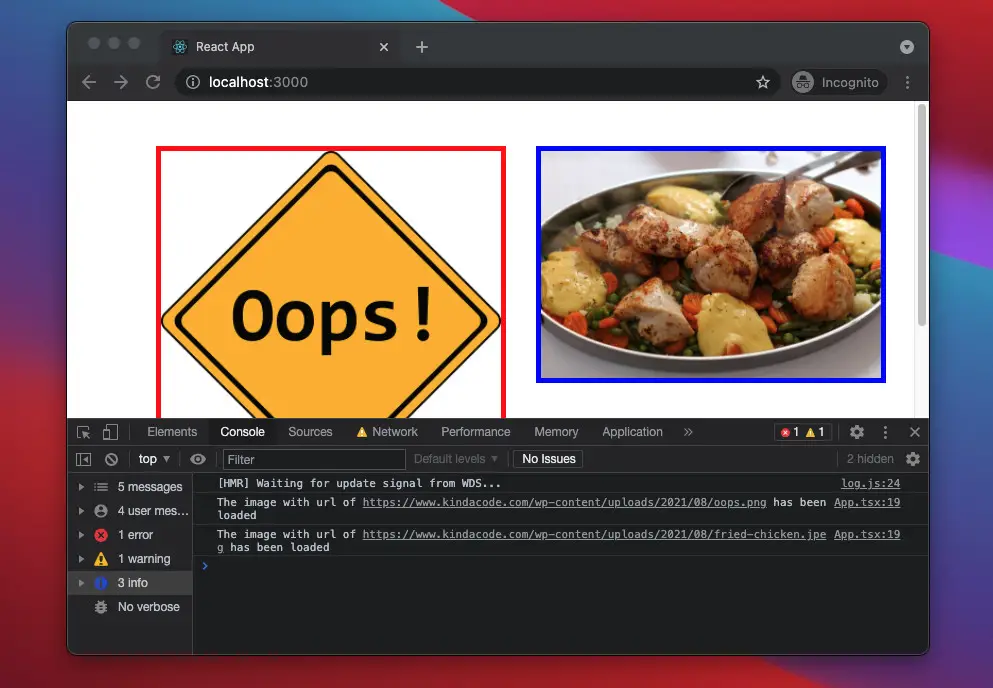
The end-to-end example below shows you how to handle image events in a React app that uses TypeScript. There are 2 events that we are going to work with:
- onLoad: This event occurs when an image has been loaded.
- onError: This event is triggered if an error occurs while loading an image.
Table of Contents
Example
App Preview
The app we are going to build will display 2 images. The first image is faulty but the second one is not. With the error image, a replacement image takes its place (thanks to the onError event). Also, the URLs of the images will be printed out in the console when they have finished loading (thanks to the onLoad event).
Below is how our app works. If your internet speed is fast, go to Chrome DevTools > Network > Fast 3G (or slow 3G) to see the loading process more clearly.
The Code
1. Create a new project by running:
npx create-react-app --template typescript
2. Remove the boilerplate in src/App.tsx and add the following:
// App.tsx
// Kindacode.com
import React from "react";
import "./App.css";
const IMAGE_1 = "https://test.kindacode.com/this-image-does-not-exist.jpeg";
const IMAGE_2 =
"https://www.kindacode.com/wp-content/uploads/2021/08/fried-chicken.jpeg";
// This image will be used as the fallback for the error images
const FALLBACK_IMAGE =
"https://www.kindacode.com/wp-content/uploads/2021/08/oops.png";
const App = () => {
// This function is triggered when an image has been loaded
const imageOnLoadHandler = (
event: React.SyntheticEvent<HTMLImageElement, Event>
) => {
console.log(
`The image with url of ${event.currentTarget.src} has been loaded`
);
if (event.currentTarget.className !== "error") {
event.currentTarget.className = "success";
}
};
// This function is triggered if an error occurs while loading an image
const imageOnErrorHandler = (
event: React.SyntheticEvent<HTMLImageElement, Event>
) => {
event.currentTarget.src = FALLBACK_IMAGE;
event.currentTarget.className = "error";
};
return (
<div className="container">
<img
src={IMAGE_1}
onLoad={imageOnLoadHandler}
onError={imageOnErrorHandler}
alt="www.kindacode.com"
/>
<img
src={IMAGE_2}
onLoad={imageOnLoadHandler}
onError={imageOnErrorHandler}
alt="www.kindacode.com"
/>
</div>
);
};
export default App;
3. Replace the unwanted CSS code in src/App.css with the following:
/*
App.css
Kindacode.com
*/
.container {
box-sizing: border-box;
margin: 30px auto;
width: 700px;
display: flex;
align-items: start;
}
img {
max-width: 340px;
margin: 15px;
}
/* Non-error image */
.success {
border: 5px solid blue;
}
/* Error image */
.error {
border: 5px solid red;
}
Conclusion
We’ve gone through a complete example of handling image onLoad and onError events in React and TypeScript. If your project is large and has a lot of images, then it’s hard to guarantee that all of them will be loaded correctly. Therefore, understanding the onError event is important if you want to improve your user experience.
If you’d like to explore more about modern React and TypeScript, take a look at the following articles:
- React + TypeScript: Handling onClick event
- React + TypeScript: Handling Keyboard Events
- React + TypeScript: Multiple Dynamic Checkboxes
- React + TypeScript: Password Strength Checker example
- React + TypeScript: Using Inline Styles Correctly
- React + TypeScript: Create an Autosize Textarea from scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.