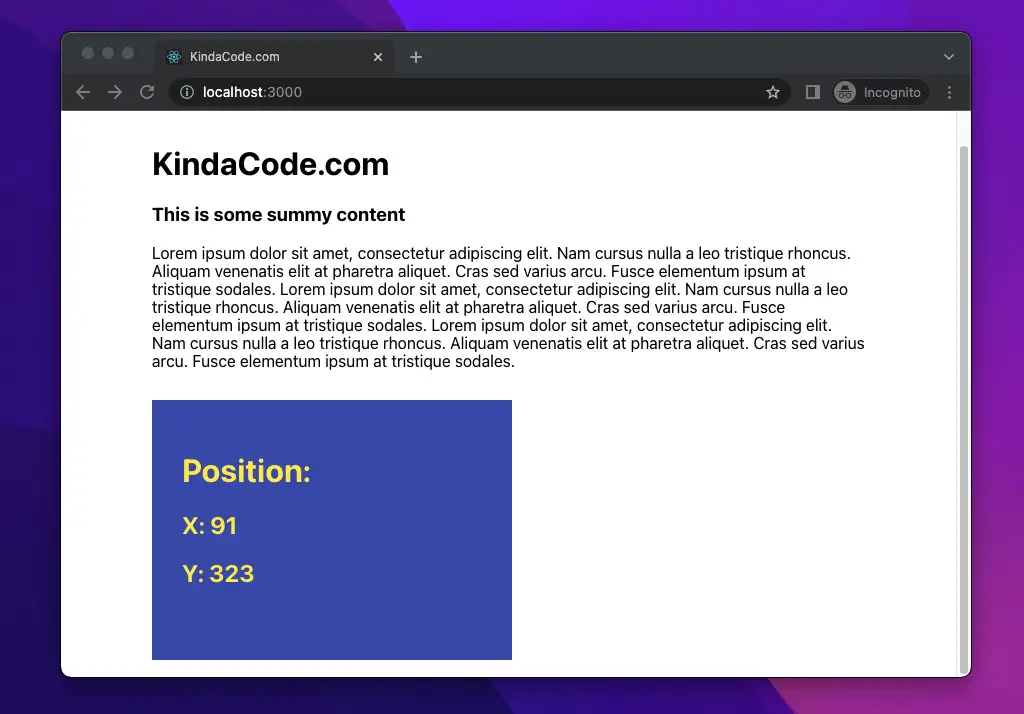
This article shows you how to get the position of an element in React regardless of whether the element is fixed or repositionable. We’ll explore the technique to get the job done and then walk through a complete example of applying that in practice. The code will be written in Javascript and TypeScript for your reference according to your preferences. We’ll use modern features of React like hooks and functional components. Old-fashioned stuff like class-based components or things related to them won’t appear.
Overview
The X (or offsetLeft position) and Y (or the offsetTop position) are both in pixels. In simple words, they are the distance from the left and top edges of an element to the left and top edges of the viewport, respectively:
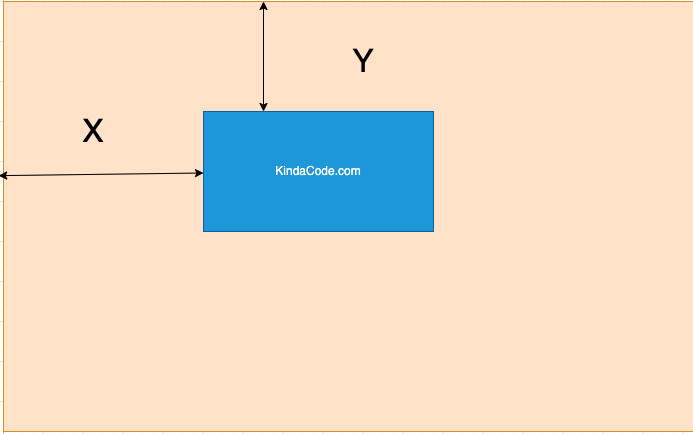
The position of an element can be consistent or changeable, such as:
- Your app contains dynamic content, and they change over time. This content will take up space and push your element down or in another direction.
- Your app is responsive, and when the user resizes the window, the layout changes as well.
In React, we can take advantage of the useRef hook to get the coordinate of an arbitrary element like so:
const myRef = useRef();
// X
const x = myRef.current.offsetLeft;
// Y
const y = myRef.current.offsetTop;
It’s important to tie the ref to the element you want to get the position:
<div ref={myRef}>
{/* Other things here */}
</div>
Please see the complete example below for more clarity if you get confused.
The Example
App Preview
The small app we will build displays some text (you can replace them with dynamic content fetched from the API or generated by the user) and a blue box. Our job is to calculate the box’s position and display that information on the screen. Whenever the browser window is resized, X and Y of the blue box will be updated immediately.
A demo is worth more than a thousand of words:
Javascript Code
If you only love to work with TypeScript, you can skip this section and move to the next one.
1. Create a new React project:
npx create-react-app react_kindacode
2. Here’s the full source code in src/App.tsx:
// App.js
import { useRef, useState, useEffect } from "react";
import "./App.css";
function App() {
// THis ref will be connected to the orange box
const boxRef = useRef();
// X
const [x, setX] = useState();
// Y
const [y, setY] = useState();
// This function calculate X and Y
const getPosition = () => {
const x = boxRef.current.offsetLeft;
setX(x);
const y = boxRef.current.offsetTop;
setY(y);
};
// Get the position of the red box in the beginning
useEffect(() => {
getPosition();
}, []);
// Re-calculate X and Y of the red box when the window is resized by the user
useEffect(() => {
window.addEventListener("resize", getPosition);
}, []);
return (
<div className="wrapper">
<div className="content">
<h1>KindaCode.com</h1>
<h3>This is some summy content</h3>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam cursus
nulla a leo tristique rhoncus. Aliquam venenatis elit at pharetra
aliquet. Cras sed varius arcu. Fusce elementum ipsum at tristique
sodales. Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam
cursus nulla a leo tristique rhoncus. Aliquam venenatis elit at
pharetra aliquet. Cras sed varius arcu. Fusce elementum ipsum at
tristique sodales. Lorem ipsum dolor sit amet, consectetur adipiscing
elit. Nam cursus nulla a leo tristique rhoncus. Aliquam venenatis elit
at pharetra aliquet. Cras sed varius arcu. Fusce elementum ipsum at
tristique sodales.
</p>
</div>
<div className="box" ref={boxRef}>
<h1>Position: </h1>
<h2>X: {x ?? "No result"}</h2>
<h2>Y: {y ?? "No result"}</h2>
</div>
</div>
);
}
export default App;
3. And here is the code for src/App.css:
.wrapper {
padding-left: 10vw;
padding-right: 10vw;
padding-top: 8vh;
padding-bottom: 3vh;
}
/* Style the box */
.box {
margin-top: 30px;
width: 300px;
height: 200px;
padding: 30px;
background: #3f51b5;
color: #ffeb3b;
}
4. Get the project up and running:
npm start
TypeScript Code
1. Create a new React project:
npx create-react-app react_kindacode --template typescript
2. Here’s the full source code in src/App.tsx:
// App.tsx
import { useRef, useState, useEffect } from "react";
import "./App.css";
function App() {
// THis ref will be connected to the orange box
const boxRef = useRef<HTMLDivElement>(null);
// X
const [x, setX] = useState<number | undefined>();
// Y
const [y, setY] = useState<number | undefined>();
// This function calculate X and Y
const getPosition = () => {
const x = boxRef.current?.offsetLeft;
setX(x);
const y = boxRef.current?.offsetTop;
setY(y);
};
// Get the position of the red box in the beginning
useEffect(() => {
getPosition();
}, []);
// Re-calculate X and Y of the red box when the window gets resized by the user
useEffect(() => {
window.addEventListener("resize", getPosition);
}, []);
return (
<div className="wrapper">
<div className="content">
<h1>KindaCode.com</h1>
<h3>This is some summy content</h3>
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam cursus
nulla a leo tristique rhoncus. Aliquam venenatis elit at pharetra
aliquet. Cras sed varius arcu. Fusce elementum ipsum at tristique
sodales. Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nam
cursus nulla a leo tristique rhoncus. Aliquam venenatis elit at
pharetra aliquet. Cras sed varius arcu. Fusce elementum ipsum at
tristique sodales. Lorem ipsum dolor sit amet, consectetur adipiscing
elit. Nam cursus nulla a leo tristique rhoncus. Aliquam venenatis elit
at pharetra aliquet. Cras sed varius arcu. Fusce elementum ipsum at
tristique sodales.
</p>
</div>
<div className="box" ref={boxRef}>
<h1>Position: </h1>
<h2>X: {x ?? "No result"}</h2>
<h2>Y: {y ?? "No result"}</h2>
</div>
</div>
);
}
export default App;
3. The code for src/App.css is the same as the CSS code in the Javascript version of this example.
Conclusion
We’ve examined an end-to-end example that demonstrates how to calculate the position of an element. React is awesome, and there are many things to learn about it. Keep moving forward and sharpen your skills by taking a look at the following articles:
- React + TypeScript: Making a Reading Progress Indicator
- React: Show Image Preview before Uploading
- React Router: How to Highlight Active Link
- React + TypeScript: Handling onScroll event
- React + TypeScript: Multiple Dynamic Checkboxes
- React: Using inline styles with the calc() function
- React: Create an Animated Side Navigation from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.
It’s calculating x and y accurately. I’m getting the wrong values. Could you please help me out with this? Following is my code
I see nothing
You want me to share the code?