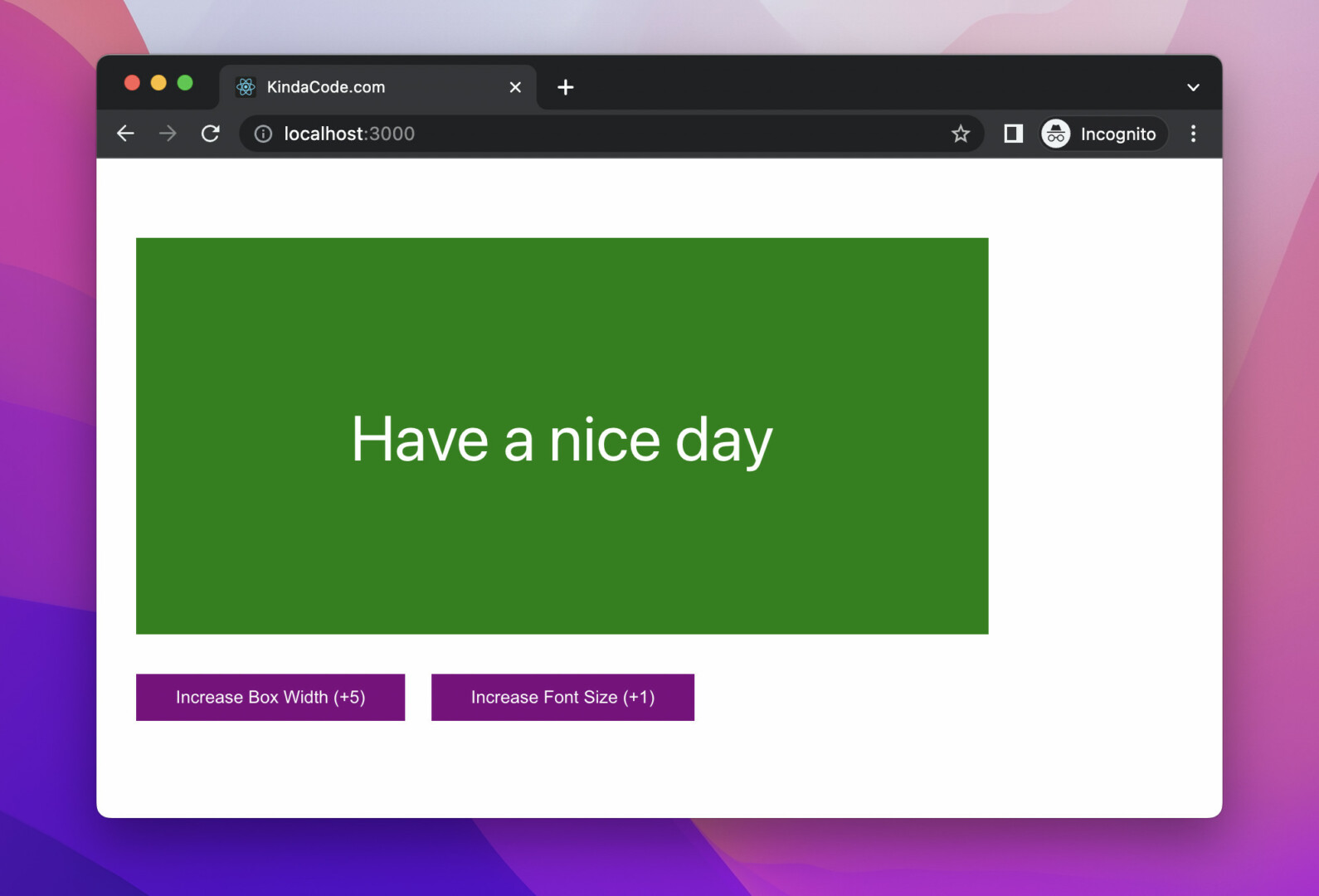
This comprehensive article shows you how to use Styled JSX in a React application created by create-react-app.
Overview
Before using styled JSX, you need to install the styled-jsx package:
npm i styled-jsx
No extra configuration is required for the vast majority of use cases.
Styled JSX Scope
With styled JSX, you can use standard CSS syntax in your Javascript (or TypeScript) files. Just put your CSS code inside a pair of back-ticks (“) and the <style> or <style jsx> tag as follows:
function App() {
return (
<div className="wrapper">
<h1>Hello</h1>
<style>
{`
.wrapper {
background: #3f51b5;
padding: 60px 30px;
}
h1 {
color: #ff9800;
}
`}
</style>
</div>
);
}
Styled JSX, by default, is scoped to a single component only (local styles) by applying additional unique class names to styled elements. That’s why the styles in the preceding code snippet DO NOT affect elements with the wrapper class name or <h1> tags in other components. Therefore, you won’t have to worry about using duplicate class names.
Dynamic Styles
You can also add Javascript variables to styled JSX to create dynamic styles, like so:
function App() {
let width = '400px';
let height = '200px';
let isLoggedIn = false;
return (
<div className="main">
<style>{`
.main {
width: ${width};
height: ${height};
background: ${isLoggedIn ? 'pink' : 'orange'};
}
`}
</style>
</div>
);
}
A Small Issue
When using the <style jsx> tag, you might see the following warning in the console:
react-dom.development.js:86 Warning: Received `true` for a non-boolean attribute `jsx`.
If you want to write it to the DOM, pass a string instead: jsx="true" or jsx={value.toString()}.
at style
at div
at App
To solve this, just use <style> or assign jsx to an empty string:
<style>{``}</style>
<style jsx=''>{``}</style>
A Complete Example
App Preview
The small app we’re going to build has a green box with white text inside it and two buttons. You can use these buttons to increase the width of the box and the font size of the text.
Here’s how it works:
The Code
1. Initialize a brand new React project:
npx create-react-app kindacode-example
The name is totally up to you.
2. Install styled-jsx:
npm i styled-jsx
3. Replace all of the default code in src/App.js with the following:
// KindaCode.com
// src/App.js
import { useState } from 'react';
function App() {
// Box width
const [boxWidth, setBoxWidth] = useState(200);
// Font size
const [fontSize, setFontSize] = useState(14);
return (
<div className="wrapper">
<div className='box'>
<p className='text'>Have a nice day</p>
</div>
{/* This button is use to increase the box width */}
<button
className='btn'
onClick={() => {
setBoxWidth(boxWidth + 5);
}}>
Increase Box Width (+5)
</button>
{/* This button is used increase text font size */}
<button className='btn'
onClick={() => setFontSize(fontSize + 1)}>
Increase Font Size (+1)
</button>
<style>
{`
.wrapper {
padding: 60px 30px;
}
.box {
width: ${boxWidth}px;
height: 300px;
display: flex;
justify-content: center;
align-items: center;
background: green;
}
.text {
font-size: ${fontSize}px;
color: white;
}
.btn {
margin: 30px 20px 30px 0px;
padding: 10px 30px;
cursor: pointer;
border: none;
background: purple;
color: white;
}
.btn:hover {
background: red;
}
`}
</style>
</div>
);
}
export default App;
4. Run the project:
npm start
And go the http://localhost:3000 to examine the result.
Conclusion
You’ve learned how to use styled JSX with create-react-app. From this time forward, you have one more option to choose from for building user interfaces. If you’d like to explore more new and interesting stuff about modern React development, take a look at the following articles:
- React Router: Redirecting with the Navigate component
- React: 2 Ways to Open an External Link in New Tab
- How to Use Tailwind CSS in React
- Working with the useId() hook in React
- React: Get the Position (X & Y) of an Element
- React + TypeScript: Making a Reading Progress Indicator
You can also check our React category page and React Native category page for the latest tutorials and examples.