This article shows you 2 approaches to programmatically changing the page title in a React web app. We will go through 2 examples: the first one uses self-written code, and the second one makes use of a third-party library called React Helmet.
Using self-written code
Example Preview
In this example, our app is initially titled “Default Title”. However, the page title can change whenever the user enters (or remove) something in the text field.
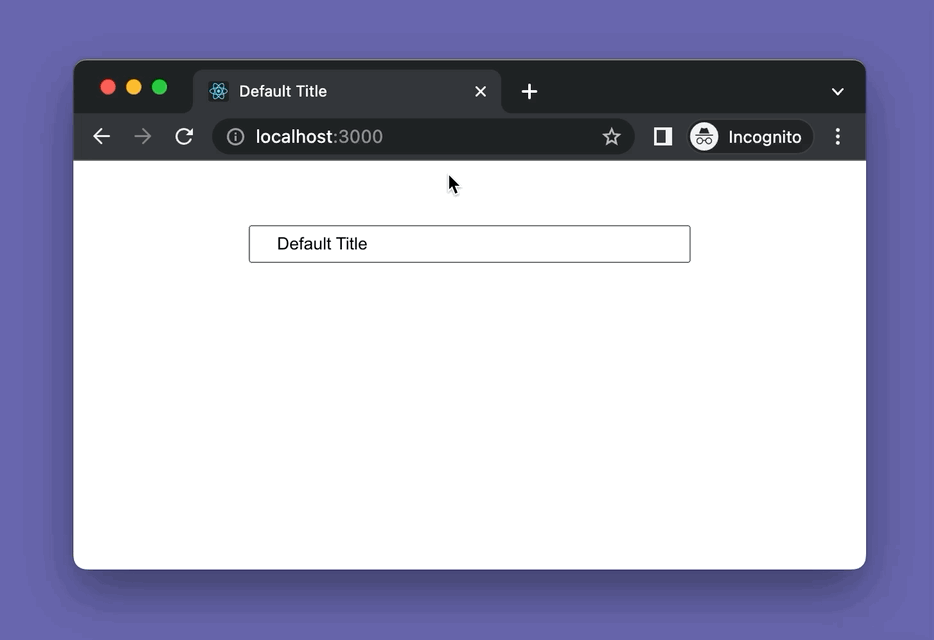
The Complete Code
// App.js
// Kindacode.com
import { useEffect, useState } from "react";
const App = () => {
const [title, setTitle] = useState("Default Title");
useEffect(() => {
// This will run when the page first loads and whenever the title changes
document.title = title;
}, [title]);
const changeTitle = (event) => {
setTitle(event.target.value);
};
return (
<div style={styles.container}>
<input
type="text"
onChange={changeTitle}
value={title}
style={styles.input}
/>
</div>
);
};
export default App;
// Just some styles
const styles = {
container: {
width: 500,
margin: "50px auto",
display: "flex",
justifyContent: "center",
},
input: {
width: 300,
padding: "5px 20px",
},
};
Using the React Helmet Async package
React Helmet Async is a popular open-source React library that manages all of your changes to the document head. You can add it to your project by running:
npm i react-helmet-async
React Helmet Async is a fork of React Helmet (which hasn’t been updated for several years).
Example Preview
In this example, we pretend to be fetching data from a server, and it takes 3 seconds to be done. When the data fetching is complete, the page title will be updated.

The Full Code
// App.js
// Kindacode.com
import { useEffect, useState } from "react";
import { Helmet, HelmetProvider } from "react-helmet-async";
const App = () => {
const [title, setTitle] = useState("");
const [content, setContent] = useState("Loading...");
useEffect(() => {
const fetchData = async () => {
// We pretend to be fetching data from a server and it takes 3 seconds
await new Promise((resolve) => setTimeout(resolve, 3000));
// When the data "fetching" process is complete, we will set the title and content
setTitle("This is a cool title");
setContent("Everything is done");
};
fetchData();
}, []);
return (
<HelmetProvider>
<Helmet>
<title>{title ? title : "No title"}</title>
</Helmet>
<div style={{ padding: 100 }}>
<h1>{content}</h1>
</div>
</HelmetProvider>
);
};
export default App;
Conclusion
In the vast majority of modern web applications (especially single-page applications), the content is loaded dynamically from the server instead of static text like in the 90s. That’s why we need to set the page titles programmatically, as in the 2 examples above.
If you would like to explore more about React and frontend development, take a look at the following articles:
- React + TypeScript: Handling onFocus and onBlur events
- React: Create an Animated Side Navigation from Scratch
- React: Copy to Clipboard When Click a Button/Link
- 5 best open-source WYSIWYG editors for React
- How to create a Filter/Search List in React
- React: How to Create a Responsive Navbar from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.
Didn’t say pros and cons of both