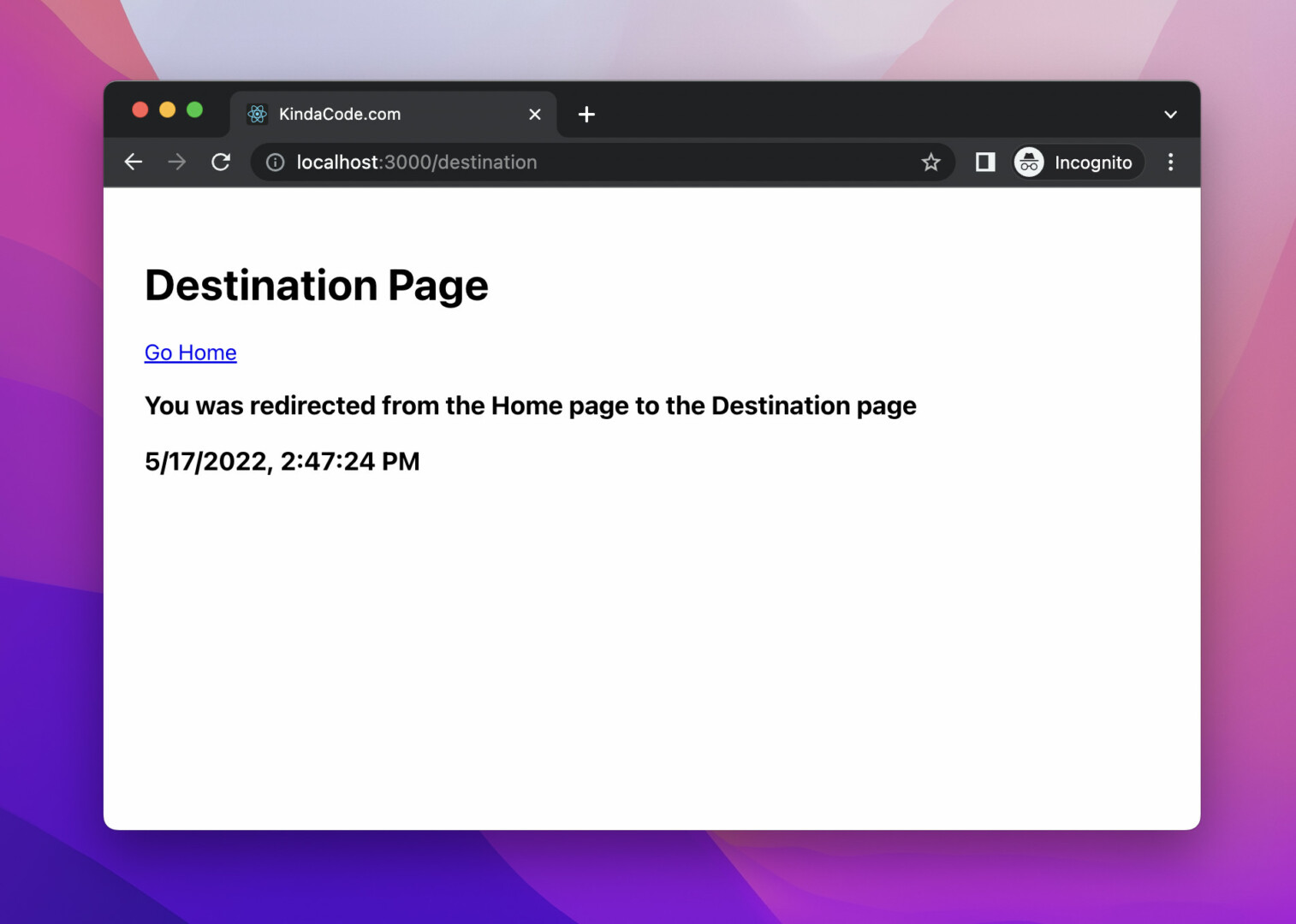
In this article, we’ll explore the fundaments of the <Navigate> component in React Router and then walk through a complete example of using it in practice. We’ll use the latest version of React Router (6.x) and modern features of React including hooks and functional components. Old-fashioned stuff like class-based components won’t appear.
Overview
The <Navigate> component is the replacement for the <Redirect> component which was removed in React Router 6. It’s a wrapper around the useNavigate hook and can change the current location when it’s rendered.
The table below lists the props of <Navigate>.
Prop | Required/Optional | Type | Description |
---|---|---|---|
to | required | To | the destination route |
replace | optional | boolean | if true, replaces the current entry on the stack instead of pushing a new one |
state | optional | any | passes data (state) to the destination |
Note: The type definition of To is string | Partial<Path>
To retrieve the passed data via the <Navigate> component, we can use the useLocation hook like so:
// KindaCode.com
import { useLocation } from 'react-router-dom';
/*...*/
const location = useLocation();
const data = location.state;
console.log(data);
For more clarity, see the example below.
Complete Example
App Preview
The small React app we’re going to build consists of two routes:
- /: HomePage
- /destination: DestinationPage
The user can be redirected from HomePage to DestinationPage by clicking on the button or checking the checkbox on HomePage. We also pass some data to and display them on the DestinationPage including a text message and the time of the redirection.
A demo is worth more than a thousand words:
The Code
1. Create a new React project:
npx create-react-app kindacode-example
The name is totally up to you.
2. Install react-router-dom:
npm i react-router-dom
3. Remove all default code in your src/App.js file and add the following:
// KindaCode.com
import { useState } from 'react';
import { BrowserRouter, Routes, Route, Navigate, useLocation, Link } from 'react-router-dom'
function App() {
return (
<BrowserRouter>
<Routes>
<Route path='/' element={<HomePage />} />
<Route path='/destination' element={<DestinationPage />} />
</Routes>
</BrowserRouter>
);
}
// Home page
const HomePage = () => {
const [redirect, setRedirect] = useState(false);
return redirect ?
<Navigate
to="/destination"
replace={true}
state={{
message: 'You was redirected from the Home page to the Destination page',
time: new Date().toLocaleString()
}} />
: <div style={{ padding: 30 }}>
<h1>Home Page</h1>
<p>
<button onClick={() => setRedirect(true)}>
Continue to Destination page
</button>
</p>
<p>
<input
type="checkbox"
checked={redirect}
onChange={() => {
setRedirect(true);
}}
id="my-checkbox" />
<label htmlFor="my-checkbox"> Go to Destination page?</label>
</p>
</div>
}
// Destination page
const DestinationPage = () => {
// Retrieving passed data via the <Navigate> component
const location = useLocation();
const state = location.state;
return <div style={{ padding: 30 }}>
<h1>Destination Page</h1>
<p><Link to="/">Go Home</Link></p>
{/* Displaying passed data */}
{state.message && state.time && <div>
<h3>{state.message}</h3>
<h3>{state.time}</h3>
</div>}
</div>
}
export default App;
To keep this example short, I put all the code in a single file. However, you can organize them better by keeping each component in a separate file.
4. Now get your app up and running:
npm start
Open a web browser and go to http://localhost:3000 to see the result.
Conclusion
You’ve learned almost everything about the Navigate component in React Router and examined an end-to-end example that demonstrates how to use it in action. If you’d like to explore more new and exciting things in the modern React world, take a look at the following articles:
- React: 2 Ways to Open an External Link in New Tab
- React Router: useParams & useSearchParams Hooks
- Working with the useId() hook in React
- React: Get the Position (X & Y) of an Element
- React + TypeScript: Making a Reading Progress Indicator
- React + TypeScript: Multiple Dynamic Checkboxes
You can also check our React category page and React Native category page for the latest tutorials and examples.