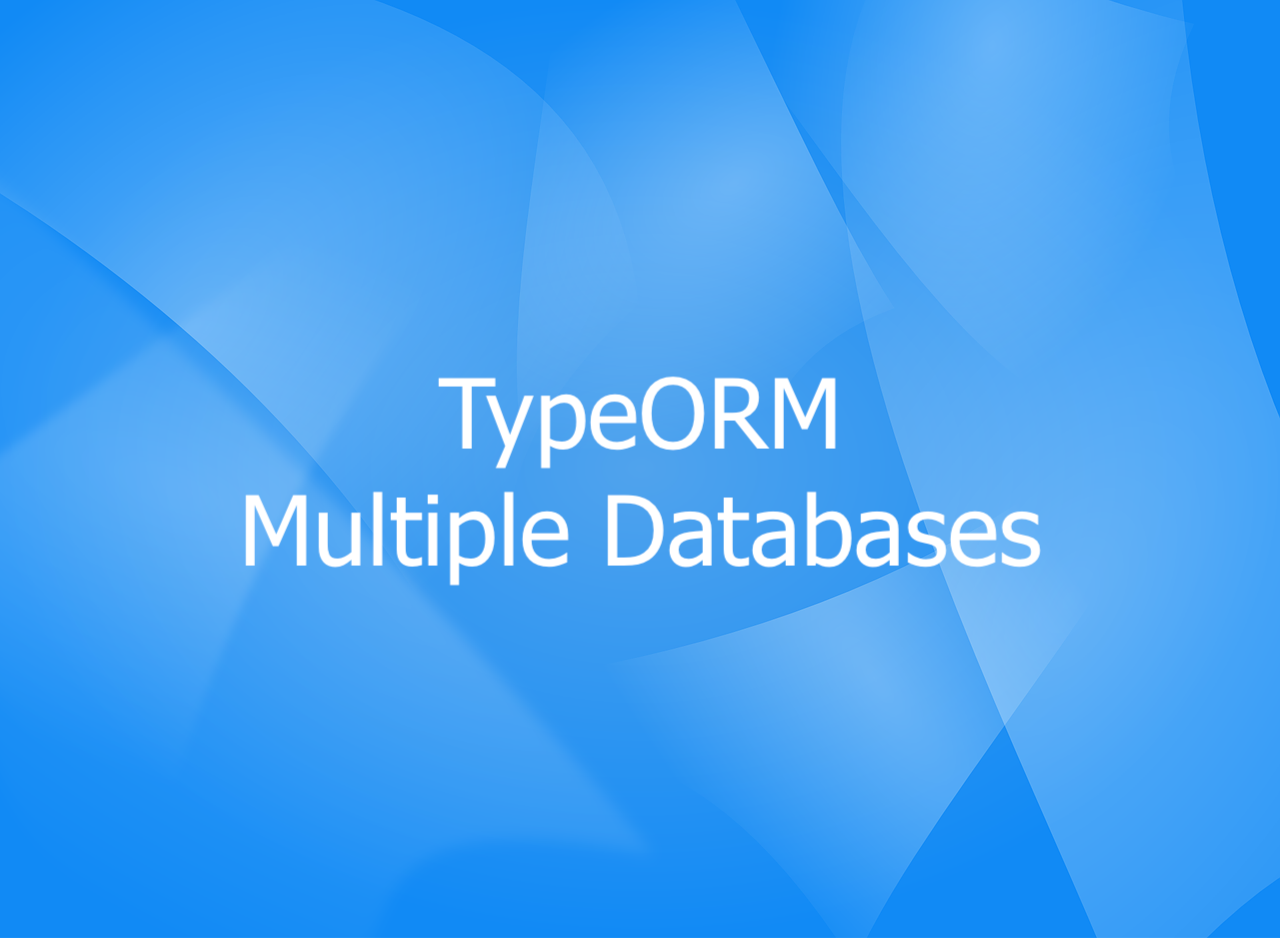
In TypeORM, you can simply connect to multiple databases simultaneously just by creating multiple data sources.
The Steps
1. Setup your data sources:
xport const dataSourceOne = new DataSource({
database: 'kindacode_example',
entities: [User, Post, Comment],
type: 'postgres',
port: 5432,
host: 'localhost',
username: 'snowball',
password: '123456',
synchronize: true,
});
export const dataSourceTwo = new DataSource({
database: 'other_db',
entities: [Product, Order],
type: 'postgres',
port: 5432,
host: 'localhost',
username: 'badman',
password: 'supersecret',
synchronize: true,
});
2. The next step is to initialize the connections (usually placed in the entry file of your project like app.js, index.js, server.js, etc):
import { dataSourceOne } from '...';
import { dataSourceTwo } from '...';
/* Put these lines in an async function */
await dataSourceOne.initialize();
await dataSourceTwo.initialize();
3. And you can do communication with the database as follows:
import { dataSourceOne } from '...';
import { dataSourceTwo } from '...';
/* Place these code lines in an async function */
const userRepository = dataSourceOne.getRepository(User);
const users = await userRepository.find();
console.log(users);
const productRepsoitory = dataSourceTwo.getRepository(Product);
const products = await productRepsoitory.find();
console.log(products);
Note that connected database types can be different (PostgreSQL, MySQL, MongoDB, etc). If you run into errors, try to update typeorm to the latest version.
Further reading:
- TypeORM: ORDER BY Multiple Columns
- TypeORM: How to Execute Raw SQL Queries
- TypeORM Upsert: Update If Exists, Create If Not Exists
- TypeORM: Find Rows Where Column Value is IN an Array
- TypeORM: Selecting Rows with Null Values
You can also check out our database topic page for the latest tutorials and examples.