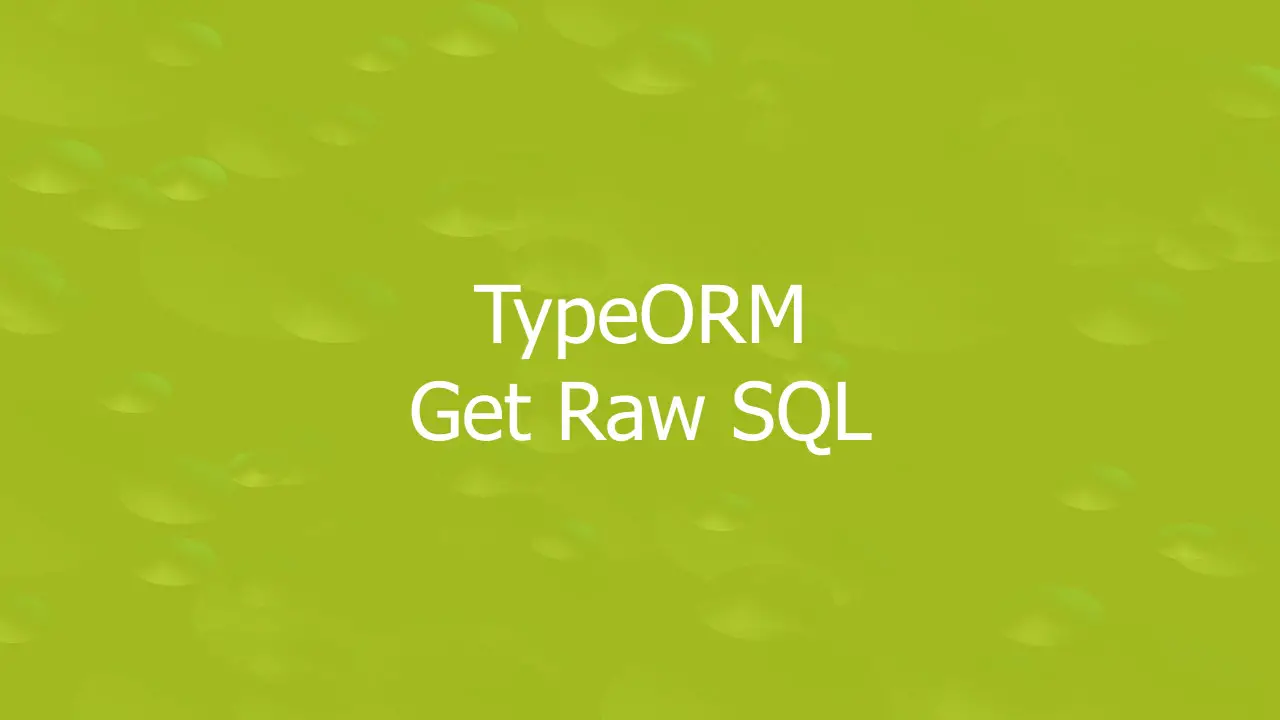
When communicating with databases through TypeORM, you can get raw SQL queries produced by query builders by using the getQuery() or getSql() methods (you can use them synchronously without the await keyword).
Example:
const userRepository = myDataSource.getRepository(User);
const myQueryBuilder = userRepository
.createQueryBuilder('user')
.select(['id', 'name', 'age'])
.where('user.age >= :age', { age: 18 })
.orderBy('user.id', 'DESC')
.take(10)
.skip(5);
const myQuery = myQueryBuilder.getQuery();
const mySql = myQueryBuilder.getSql();
console.log(myQuery);
console.log(mySql);
Output:
SELECT id, name, age FROM "user" "user" WHERE "user"."age" >= :age ORDER BY "user"."id" DESC LIMIT 10 OFFSET 5
SELECT id, name, age FROM "user" "user" WHERE "user"."age" >= $1 ORDER BY "user"."id" DESC LIMIT 10 OFFSET 5
If you have a close look at the output, you’ll notice a slight difference between the result of getQuery() and the result of getSql().
f you have prior experience working with MySQL, PostgreSQL, or SQLite, seeing the SQL generated by TypeORM will be very helpful when debugging projects.
Further reading:
- TypeORM: Selecting DISTINCT Values
- TypeORM: Sort Results by a Relation Column
- TypeORM Upsert: Update If Exists, Create If Not Exists
- TypeORM: Add Columns with Array Data Type
- TypeORM: 2 Ways to Exclude a Column from being Selected
You can also check out our database topic page for the latest tutorials and examples.