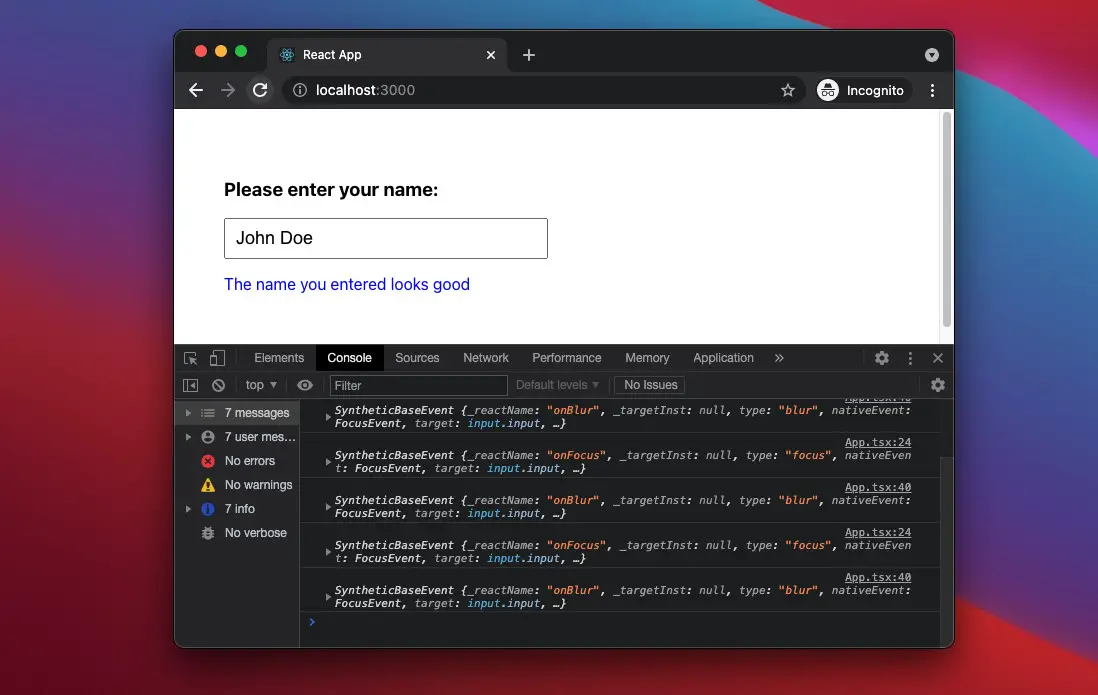
This article walks you through a complete example of handling the onFocus and the onBlur events in a React project that is written in TypeScript.
We are going to use modern React features like hooks and functional components. Therefore, before getting started, make sure your React version is 16.8 or newer.
Table of Contents
Overview
The onFocus event occurs when an element or some element inside it gets focus. The onBlur event is the opposite of the onFocus event. It occurs when an element (or some element inside it) loses focus. Both of these events work on all elements in the React DOM but are most often used with form.
The event object has the type like so:
event: React.FocusEvent<HTMLInputElement>
For more clarity, please see the example below.
Complete Example
Preview
This example demonstrates a common task: validating the name entered by a user.
- When the text field is focused, a hint will show up on the right side to info the user that only letters and spaces are accepted.
- When the onBlur event occurs, the validation logic will run and an error message will appear if the entered name is invalid. Otherwise, you will see a success message.
You can also see information about the event objects in the console.
The Code
1. Initialize a new React project by running:
npx create-react-app kindacode_example --template typescript
2. Replace all of the unwanted code in your src/App.tsx with the below:
// App.js
// Kindacode.com
import React, { useState } from "react";
import "./App.css";
const App = () => {
const [name, setName] = useState("");
const [isValid, setIsValid] = useState(false);
const [isFocus, setIsFocus] = useState(false);
const [isBlur, setIsBlur] = useState(false);
// Handling input onChange event
const changeHandler = (event: React.ChangeEvent<HTMLInputElement>) => {
setName(event.target.value);
};
// Handling input onFocus event
const focusHandler = (event: React.FocusEvent<HTMLInputElement>) => {
setIsFocus(true);
setIsBlur(false);
// Do something with event
console.log(event);
};
// Handling input onBlur event
const blurHandler = (event: React.FocusEvent<HTMLInputElement>) => {
setIsFocus(false);
setIsBlur(true);
// Validate entered name
if(name.match(/^[a-z][a-z\s]*$/i)){
setIsValid(true);
} else {
setIsValid(false);
}
// Do something with event
console.log(event);
};
return (
<div className="container">
<h3>Please enter your name:</h3>
<input
type="text"
onFocus={focusHandler}
onBlur={blurHandler}
value={name}
onChange={changeHandler}
className="input"
placeholder="Please enter your name"
/>
{isFocus && (
<span className="hint">
Only letters and spaces are acceptable
</span>
)}
{isBlur && !isValid && <p className="error">The name you entered is not valid</p>}
{isBlur && isValid && <p className="success">The name you entered looks good</p>}
</div>
);
};
export default App;
3. To make the app look better a little bit, remove all the default code in your src/App.css and add the following:
/*
App.css
Kindacode.com
*/
.container {
padding: 50px;
}
.input {
padding: 8px 10px;
width: 300px;
font-size: 18px;
}
.hint {
margin-left: 20px;
}
.error {
color: red;
}
.success {
color: blue;
}
Conclusion
We’ve gone over an example of working with the onFocus and the onBlur events in React and TypeScript. If you’d like to learn more new and interesting things about React, take a look at the following articles:
- Top 4 React form validation libraries
- React: Passing Parameters to Event Handler Functions
- React + TypeScript: Drag and Drop Example
- React + TypeScript: Handling form onSubmit event
- React + TypeScript: Using setTimeout() with Hooks
- React + TypeScript: setInterval() example (with hooks)
You can also check our React category page and React Native category page for the latest tutorials and examples.