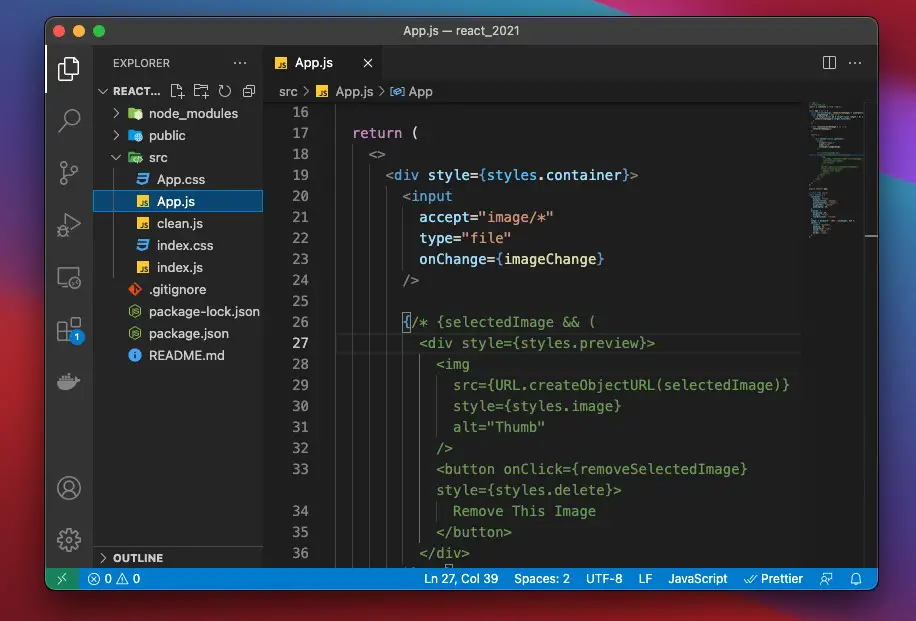
This article shows you a couple of different ways to comment out (and uncomment) a single line or a block of code in React and JSX.
Using Keyboard Shortcuts
If you are using VS Code (Visual Studio Code) to develop your React app then you can easily and quickly make comments by using keyboard shortcuts.
All you need to do is select that code block with your mouse, then press the following key combination:
- Ctrl + K, then press Ctrl + C if you’re using Windows
- Command + K, then press Command + C if you’re on a Mac
To uncomment a block of code, use your mouse to select it and then use the key combination:
- Ctrl + K then Ctrl + U if you’re on Windows
- Command + K, then Command + U if you’re on a Mac
You can also use:
- Ctrl + / (the slash key) to comment and uncomment lines of code on Windows.
- Command + / to comment and uncomment lines of code on Mac.
Demo:
Manually Comment
For JSX code, you can use {/* …*/} syntax to make comments:
return (
<>
<div style={styles.container}>
{/* <input accept="image/*" /> */}
{/* {selectedImage && (
<div style={styles.preview}>
<img
src={URL.createObjectURL(selectedImage)}
style={styles.image}
alt="Thumb"
/>
<button onClick={removeSelectedImage} style={styles.delete}>
Remove This Image
</button>
</div>
)} */}
</div>
</>
);
For non-JSX code (the code outside return or render), you can use // (double-slashes) or /*…*/ syntax to comment out lines of code, like this:
// App.js
// Kindacode.com
import { useState } from "react";
const App = () => {
const [selectedImage, setSelectedImage] = useState();
// const imageChange = (e) => {
// if (e.target.files && e.target.files.length > 0) {
// setSelectedImage(e.target.files[0]);
// }
// };
/*
const removeSelectedImage = () => {
setSelectedImage();
};
*/
return (
<>
<div></div>
</>
);
};
export default App;
Conclusion
We’ve run through a couple of techniques for making comments in React and JSX. If you’d like to explore more about modern React and frontend development, take a look at the following articles:
- React: Create an Animated Side Navigation from Scratch
- React: How to Create an Image Carousel from Scratch
- React Router Dom: Parsing Query String Parameters
- 2 Ways to Render HTML Content in React and JSX
- React + TypeScript: Create an Autosize Textarea from scratch
- 5 best open-source WYSIWYG editors for React
You can also check our React category page and React Native category page for the latest tutorials and examples.