When you need to render an array of objects in React, the most popular solution is to use the Array.map() method. However, there are other possible ways to get the same result, such as using the Array.forEach() method or using the for…of statement.
Let’s examine a concrete example for more clarity. In this example, we display an array of users:
const users = [
{ id: 'u1', name: 'John', age: 30 },
{ id: 'u2', name: 'Jane', age: 32 },
{ id: 'u3', name: 'Jack', age: 43 },
{ id: 'u4', name: 'Jill', age: 24 },
{ id: 'u5', name: 'Joe', age: 29 },
];
Screenshot:
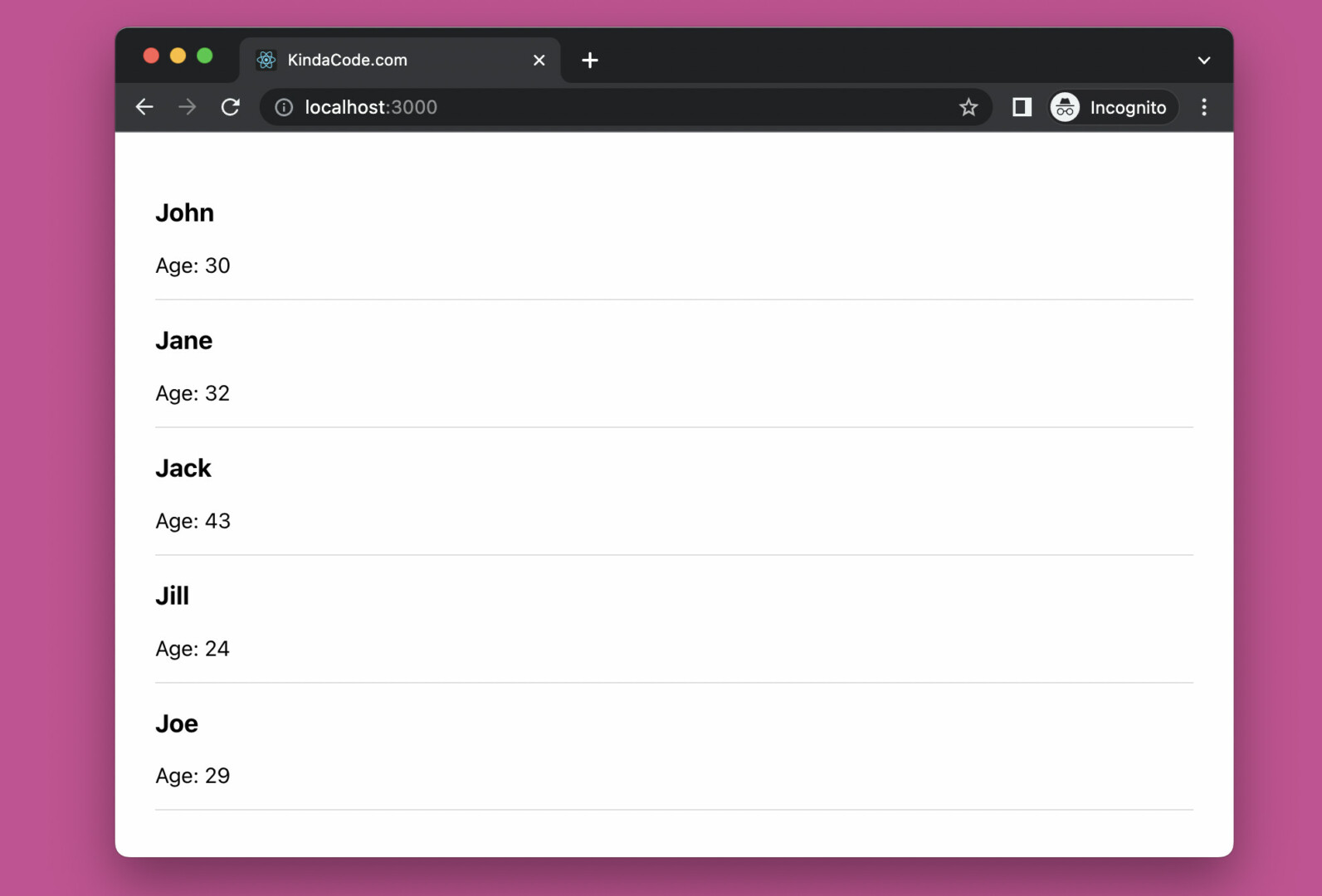
Using forEach():
// KindaCode.com
// src/App.js
function App() {
// an array of user objects
const users = [
{ id: 'u1', name: 'John', age: 30 },
{ id: 'u2', name: 'Jane', age: 32 },
{ id: 'u3', name: 'Jack', age: 43 },
{ id: 'u4', name: 'Jill', age: 24 },
{ id: 'u5', name: 'Joe', age: 29 },
];
// construct JSX from the array of user objects
const renderedUsers = [];
users.forEach((user) =>
renderedUsers.push(
<div key={user.id} style={{borderBottom: '1px solid #ddd'}}>
<h3>{user.name}</h3>
<p>Age: {user.age}</p>
</div>
)
);
return <div style={{ padding: 30 }}>{renderedUsers}</div>;
}
export default App;
Using for..of:
// KindaCode.com
// src/App.js
function App() {
// an array of user objects
const users = [
{ id: 'u1', name: 'John', age: 30 },
{ id: 'u2', name: 'Jane', age: 32 },
{ id: 'u3', name: 'Jack', age: 43 },
{ id: 'u4', name: 'Jill', age: 24 },
{ id: 'u5', name: 'Joe', age: 29 },
];
// construct JSX from the array of user objects
const renderedUsers = [];
for (let user of users) {
renderedUsers.push(
<div key={user.id} style={{ borderBottom: '1px solid #ddd' }}>
<h3>{user.name}</h3>
<p>Age: {user.age}</p>
</div>
);
}
return <div style={{ padding: 30 }}>{renderedUsers}</div>;
}
export default App;
That’s if. Further reading:
- React + TypeScript: Using setTimeout() with Hooks
- React: Load and Display Data from Local JSON Files
- React: How to Check Internet Connection (Online/Offline)
- React: How to Detect Caps Lock is On
- Display Custom Loading Screen when React Isn’t Ready
- 3 Ways to Create Toasts in React
You can also check our React category page and React Native category page for the latest tutorials and examples.