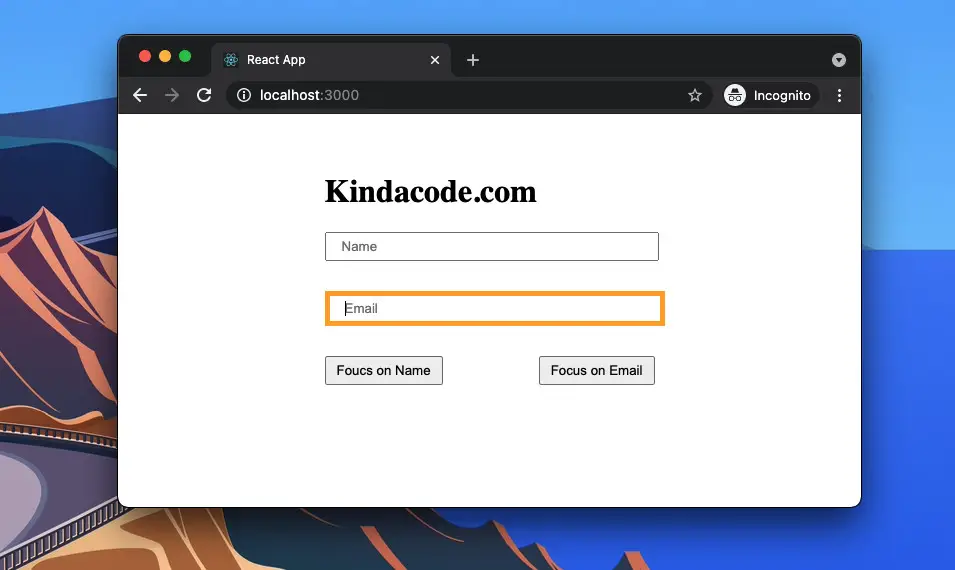
The example below shows you how to properly use the useRef hook with TypeScript in React. This task may sound simple but it can be challenging for people who aren’t familiar with types and functional components. WIthout any further ado, let’s dive right in action.
Example Preview
This example contains 2 text inputs to receive information about name and email. It also has and 2 buttons.
- In the beginning, when no action is executed, the first text input will be focused using the useEffect hook.
- When a button is clicked, the text input corresponding to it will be focused.
The demo below will give you a more intuitive look:
The Code
1. Create a new React project with the command below:
npx create-react-app kindacode_example --template typescript
2. Replace all the default code in your src/App.tsx file with the following:
import React, { useRef, useEffect } from 'react';
import './App.css';
const App = () => {
const nameRef = useRef<HTMLInputElement | null>(null);
const emailRef = useRef<HTMLInputElement | null>(null);
// Focus the name input when the app launches
useEffect(() => {
nameRef.current?.focus();
}, []);
// This function is triggered when the "Focus on Name" button is clicked
const focusonName = () => {
nameRef.current?.focus();
}
// This function is triggered when the "Focus on Email" button is clicked
const focusonEmail = () => {
emailRef.current?.focus();
}
return (
<div className="container">
<h1>Kindacode.com</h1>
<input ref={nameRef} type="text" className="input" placeholder="Name" />
<input ref={emailRef} type="text" className="input" placeholder="Email" />
<div className="btn-wrapper">
<button onClick={focusonName} className="btn">Foucs on Name</button>
<button onClick={focusonEmail} className="btn">Focus on Email</button>
</div>
</div>
);
};
export default App;
3. To make the UI look better, remove the unwanted code in src/App.css and add this:
.container {
margin: auto;
padding-top: 30px;
width: 330px;
}
.input {
margin-bottom: 30px;
padding: 5px 15px;
width: 300px;
}
.input:focus {
border: 5px solid orange;
outline: none;
}
.btn-wrapper {
display: flex;
justify-content: space-between;
}
.btn {
padding: 5px 10px;
cursor: pointer;
}
4. Run the project:
npm start
On your web browser, go to http://localhost:3000 and check the result.
Wrap Up
We’ve walked through a complete example of using the useRef hook with TypeScript. Keep the ball rolling and continue learning more fascinating stuff in React by reading the following articles:
- How to create a Filter/Search List in React
- React useReducer hook – Tutorial and Examples
- React Router useLocation hook – Tutorial and Examples
- React + TypeScript: Handling input onChange event
- ReactJS: Code an image portfolio gallery like Pinterest and Pixabay
You can also check our React topic page and React Native topic page for the latest tutorials and examples.