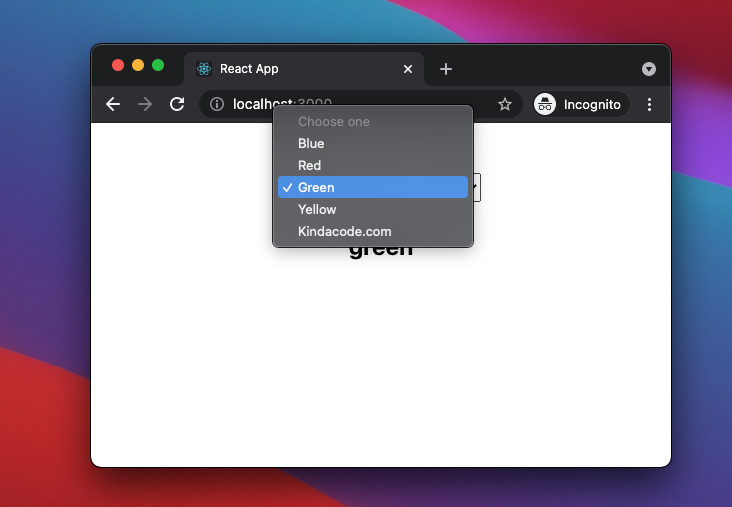
A select element is a drop-down list with multiple options. In this article, we will examine an end-to-end example of handling the onChange event of a select element in a React web app that written with TypeScript.
Table of Contents
The Example
App Preview
What we are going to build is a simple web app that has a select element in the center of the screen. When a user chooses one option from the drop-down list, the selected value will be displayed.
The Complete Code
1. Create a new project with the command below:
npx create-react-app kindacode_example --template typescript
2. Replace all of the default code in your src/App.tsx (or src/App.ts) with the following:
// App.tsx
// Kindacode.com
import React, { useState } from "react";
const App = () => {
const [selectedOption, setSelectedOption] = useState<String>();
// This function is triggered when the select changes
const selectChange = (event: React.ChangeEvent<HTMLSelectElement>) => {
const value = event.target.value;
setSelectedOption(value);
};
return (
<div style={styles.container}>
<select onChange={selectChange} style={styles.select}>
<option selected disabled>
Choose one
</option>
<option value="blue">Blue</option>
<option value="red">Red</option>
<option value="green">Green</option>
<option value="yellow">Yellow</option>
<option value="kindacode.com">Kindacode.com</option>
</select>
{selectedOption && <h2 style={styles.result}>{selectedOption}</h2>}
</div>
);
};
export default App;
// Just some styles
const styles: { [name: string]: React.CSSProperties } = {
container: {
marginTop: 50,
display: "flex",
flexDirection: "column",
alignItems: "center",
},
select: {
padding: 5,
width: 200,
},
result: {
marginTop: 30,
},
};
3. Run the project and go to http://localhost:3000 see the result:
npm start
Conclusion
You’ve learned how to handle the onChange event of a select element in React and TypeScript. If you would like to explore more new and interesting stuff about modern frontend development, take a look at the following articles:
- React + TypeScript: Handling onFocus and onBlur events
- React + TypeScript: Drag and Drop Example
- React & TypeScript: Using useRef hook example
- React + TypeScript: Using setTimeout() with Hooks
- React useReducer hook – Tutorial and Examples
- React Router Dom: Scroll To Top on Route Change
You can also check our React category page and React Native category page for the latest tutorials and examples.