Font Awesome is a high-quality icon toolkit that can help you create charming user interfaces. This tutorial will show you how to use Font Awesome icons in a React Native app.
Adding Font Awesome to your project
Install the required packages:
npm i react-native-svg @fortawesome/react-native-fontawesome @fortawesome/fontawesome-svg-core @fortawesome/free-solid-svg-icons
Link your native dependencies by running (iOS only):
npx react-native link
Usage
Import the FontAweasomeIcon component:
import {FontAwesomeIcon} from '@fortawesome/react-native-fontawesome';
Import the icons you need:
import {
// some icons
} from '@fortawesome/free-solid-svg-icons';
To see the full list of available icons, go to:
your_project/node_modules/@fortawesome/free-solid-svg-icons/index.d.ts
Example
Screenshot:
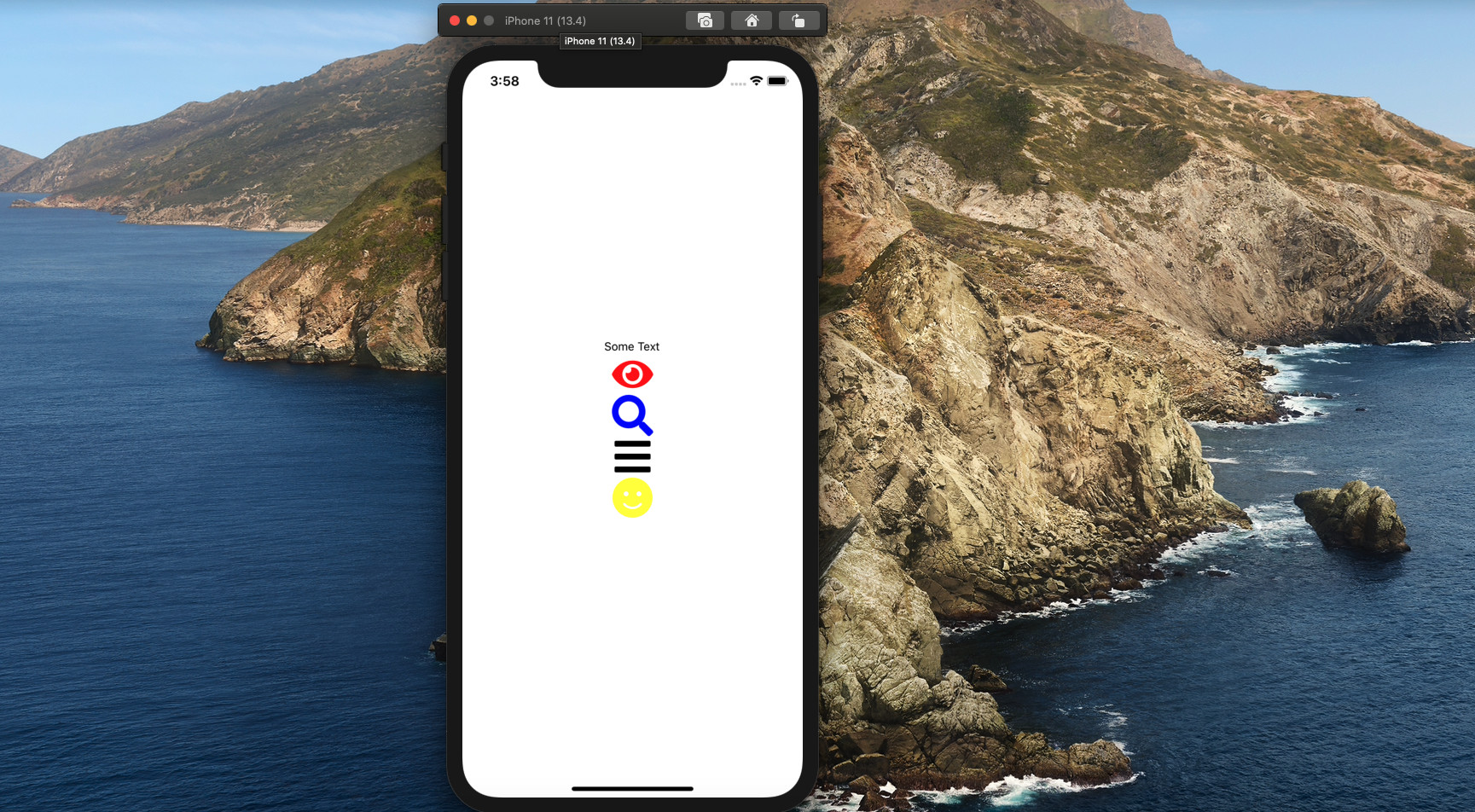
The code:
// App.js
import React from "react";
import { View, Text, StyleSheet } from "react-native";
import { FontAwesomeIcon } from "@fortawesome/react-native-fontawesome";
import {
faEye,
faSearch,
faBars,
faSmile,
} from "@fortawesome/free-solid-svg-icons";
function App() {
return (
<View style={styles.screen}>
<Text>Some Text</Text>
<FontAwesomeIcon icon={faEye} size={50} style={{ color: "red" }} />
<FontAwesomeIcon icon={faSearch} size={50} style={{ color: "blue" }} />
<FontAwesomeIcon icon={faBars} size={50} style={{ color: "black" }} />
<FontAwesomeIcon icon={faSmile} size={50} style={{ color: "yellow" }} />
</View>
);
}
export default App;
/// Just some styles
const styles = StyleSheet.create({
screen: {
flex: 1,
justifyContent: "center",
alignItems: "center",
},
});
I hope this short article will help you a little bit. Happy coding and have a nice day.
Afterword
You can find more information about Font Awesome in its official docs or on GitHub.
If you’d like to learn more new and exciting things about modern React Native, take a look at the following articles:
- Using Switch component in React Native (with Hooks)
- How to Get the Window Width & Height in React Native
- How to Implement a Picker in React Native
- How to render HTML content in React Native
- How to implement tables in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.