
In many cases, you will need to implement a confirm dialog to let the user confirm that they are sure they want to perform an action. This helps prevent consequences when a user accidentally clicks the delete button or something similar.
This article walks you through a complete example of making a confirm dialog with Yes/No (or OK/Cancel) buttons in React Native.
Example Preview
The sample app we are going to build is pretty simple. It contains a color box and a “Delete” button. When the user tap the button, a dialog with “Yes” and “No” buttons will show up. If the user selects “Yes”, the box will be removed. If the user selects “No”, things won’t change.
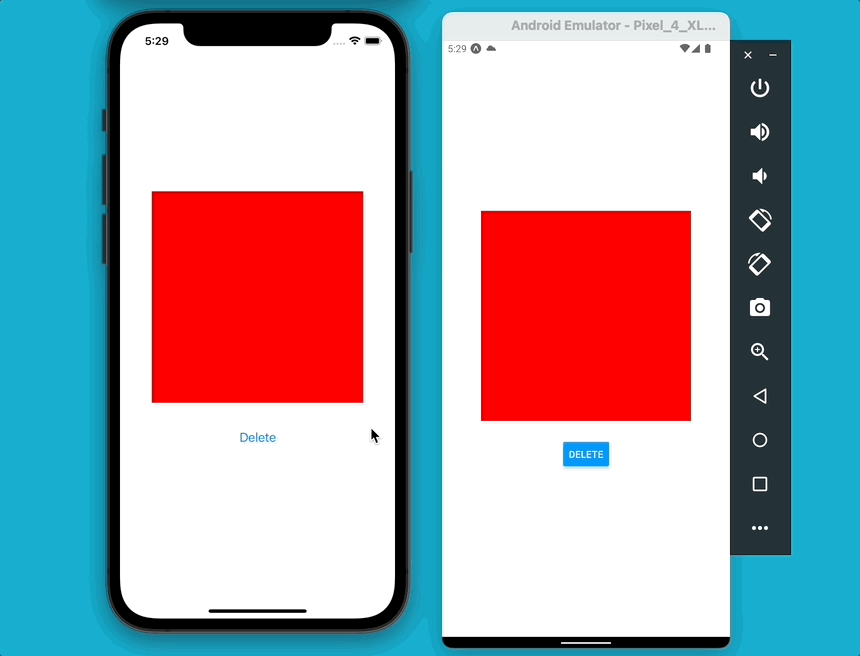
The Code
Replace all the unwanted code in your App.js with the following:
import React, { useState } from "react";
import { View, Button, StyleSheet, Alert } from "react-native";
export default function App() {
const [showBox, setShowBox] = useState(true);
const showConfirmDialog = () => {
return Alert.alert(
"Are your sure?",
"Are you sure you want to remove this beautiful box?",
[
// The "Yes" button
{
text: "Yes",
onPress: () => {
setShowBox(false);
},
},
// The "No" button
// Does nothing but dismiss the dialog when tapped
{
text: "No",
},
]
);
};
return (
<View style={styles.screen}>
{showBox && <View style={styles.box}></View>}
<Button title="Delete" onPress={() => showConfirmDialog()} />
</View>
);
}
// Kindacode.com
// Just some styles
const styles = StyleSheet.create({
screen: {
flex: 1,
justifyContent: "center",
alignItems: "center",
},
box: {
width: 300,
height: 300,
backgroundColor: "red",
marginBottom: 30,
},
text: {
fontSize: 30,
},
});
Final Words
You’ve learned to implement a confirm dialog by using the Alert API in React Native. This knowledge is very helpful to protect your users from accidentally performing an unwanted action. Continue learning more interesting and new stuff by taking a look at the following articles:
- Using Image Picker and Camera in React Native (Expo)
- How to render HTML content in React Native
- How to implement tables in React Native
- Best open-source React Native UI libraries
- React Native FlatList: Tutorial and Examples
- Using Font Awesome Icons in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.
thanks alot thats what im searching for
thanks
THanks