This article shows you how to determine the window size (width and height of the current viewport) in React Native. Without any further ado (like talking about the history of React Native or Javascript), let’s move on to the things that matter.
Note: On phones and smartwatches, your app will run in fullscreen mode, so the window size is also the screen size. On a large device like an iPad, if your app runs in split view, the size of the window will be smaller than the size of the screen.
Overview
In modern React Native, you can get the window size in two different ways. The first approach is to use the useWindowDimensions hook, as shown below:
import { useWindowDimensions } from "react-native";
const size = useWindowDimensions();
const width = size.width;
const height = size.height;
Using this hook is recommended since it updates the returned information as the window’s dimensions update.
The second approach is to use the Dimensions API like this:
import { Dimensions } from 'react-native';
const width = Dimensions.get('window').width;
const height = Dimensions.get('window').height;
In the following example, we will make use of the useWindowDimensions hook.
Complete Example
App Preview
Here is the tiny app we are going to make:
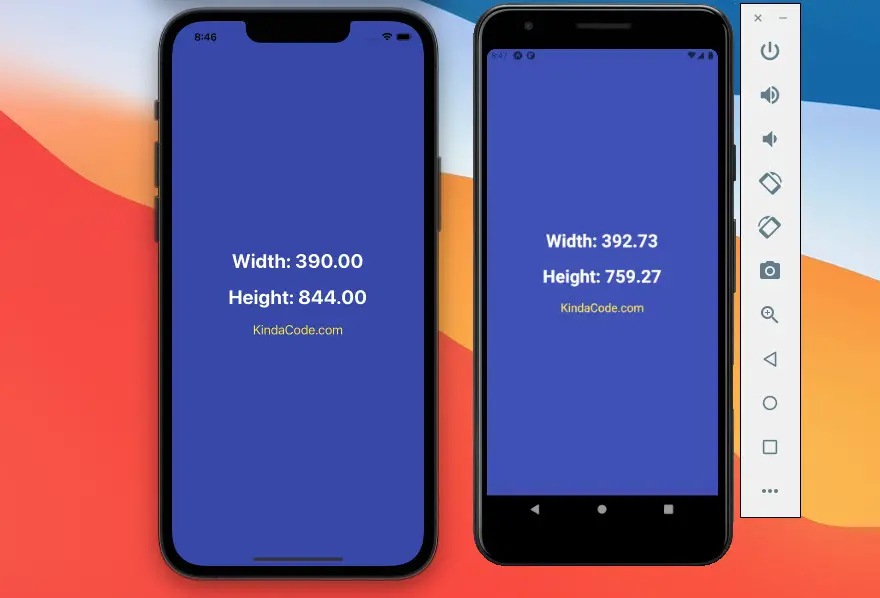
Final Code
The full source code in App.js:
// App.js
import React from "react";
import { useWindowDimensions , StyleSheet, View, Text } from "react-native";
export default function App() {
const size = useWindowDimensions();
return <View style={styles.container}>
<Text style={styles.text}>Width: {size.width.toFixed(2)}</Text>
<Text style={styles.text}>Height: {size.height.toFixed(2)}</Text>
<Text style={styles.textSmall}>KindaCode.com</Text>
</View>;
}
// Kindacode.com
// Just some styles
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: "column",
backgroundColor: "#3f51b5",
alignItems: "center",
justifyContent: "center",
},
text: {
fontSize: 30,
fontWeight: 'bold',
color: '#fff',
marginBottom: 20
},
textSmall: {
fontSize: 20,
color: '#ffeb3b'
}
});
Conclusion
You’ve learned how to read the viewport width and height in a React Native app. If you’d like to explore more new and awesome stuff about the framework, take a look at the following articles:
- React Native: Make a Button with a Loading Indicator inside
- React Native – How to Update Expo SDK
- Top 4 free React Native UI libraries
- How to render HTML content in React Native
- How to Implement a Picker in React Native
- How to Create a Confirm Dialog in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.