
There might be cases where you want to empty an entire folder in Node.js. This article shows you a couple of different ways to do so. The first approach uses self-written code while the second one makes use of a third-party package. You can choose from them which fits your needs.
Using Self-Written Code
This method is a little verbose. First, we’ll find all files in the given folder. Next. we will delete these files one by one with a for loop.
Example
Project structure:
.
├── files
│ ├── a.jpg
│ ├── b.png
│ ├── c.xml
│ ├── d.csv
│ └── e.txt
├── index.js
└── package.json
Screenshot:
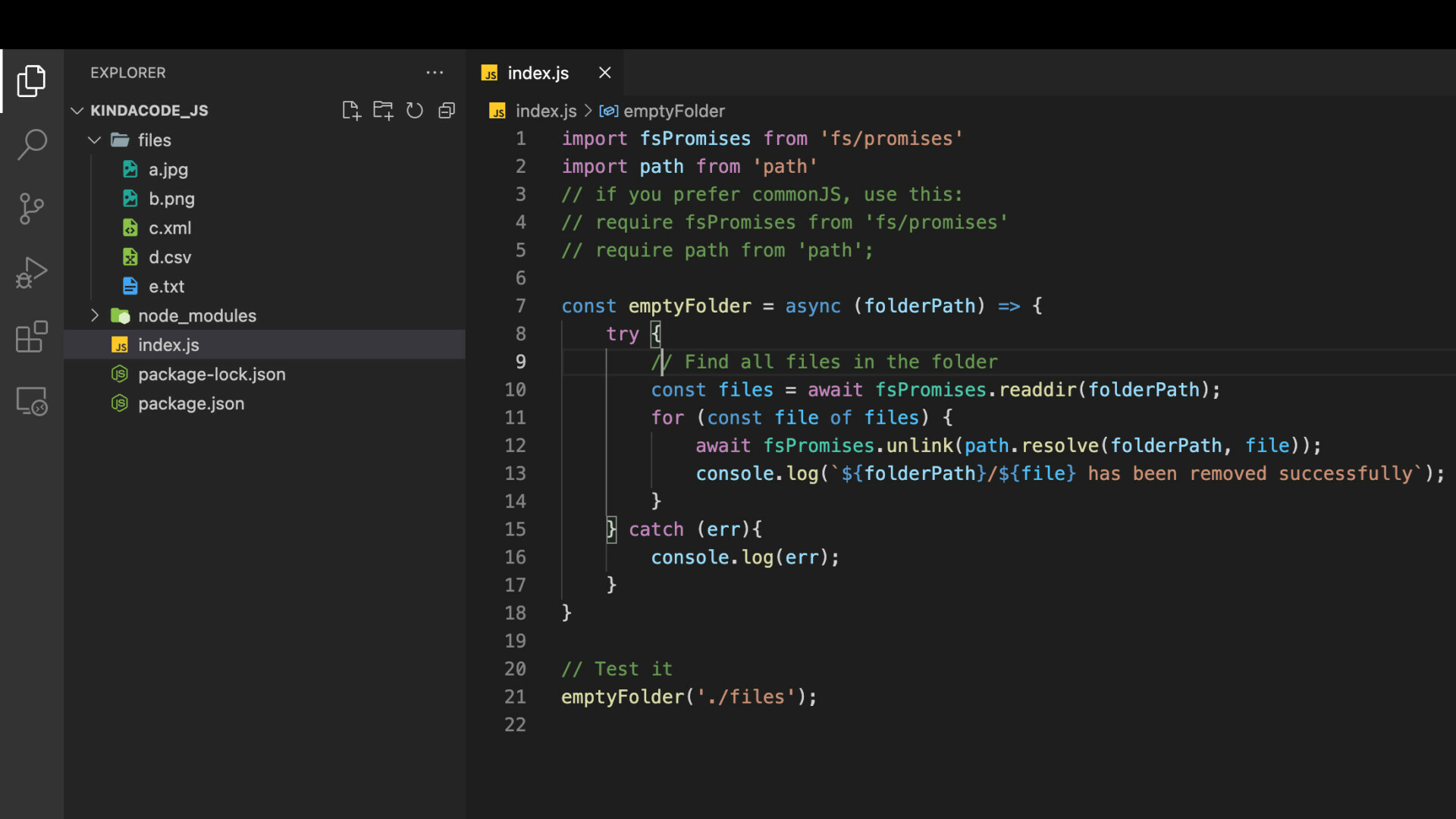
The complete code in index.js:
// kindacode.com
// index.js
import fsPromises from 'fs/promises'
import path from 'path'
// if you prefer commonJS, use this:
// require fsPromises from 'fs/promises'
// require path from 'path';
const emptyFolder = async (folderPath) => {
try {
// Find all files in the folder
const files = await fsPromises.readdir(folderPath);
for (const file of files) {
await fsPromises.unlink(path.resolve(folderPath, file));
console.log(`${folderPath}/${file} has been removed successfully`);
}
} catch (err){
console.log(err);
}
}
// Test it
emptyFolder('./files');
Output:
./files/a.jpg has been removed successfully
./files/b.png has been removed successfully
./files/c.xml has been removed successfully
./files/d.csv has been removed successfully
./files/example.txt has been removed successfully
Using a Third-Party Library
This approach is very quick and we only have to write a single line of code to get the job done. But first, we need to install a package named fs-extra:
npm i fs-extra
Then we can synchronously clear everything in the given folder like this:
// kindacode.com
// index.js
import fsExtra from 'fs-extra'
fsExtra.emptyDirSync('./files');
If you want to asynchronously empty the folder (with async/await), use this code:
// kindacode.com
// index.js
import fsExtra from 'fs-extra'
const emptyFolder = async (folderPath) => {
try {
await fsExtra.emptyDir(folderPath);
console.log('Done!');
} catch (err){
console.log(err);
}
}
// Run the function
emptyFolder('./files');
Conclusion
We’ve walked through two ways to empty a given directory in Node.js. Which one you will use in your project? Please let us know by leaving a comment. If you’d like to explore more new and interesting stuff about modern Node.js, take a look at the following articles:
- 2 Ways to Set Default Time Zone in Node.js
- Node.js: How to Compress a File using Gzip format
- 4 Ways to Read JSON Files in Node.js
- Node.js: Using __dirname and __filename with ES Modules
- Node.js: Generate Images from Text Using Canvas
- Node.js: Ways to Create a Directory If It Doesn’t Exist
You can also check out our Node.js category page for the latest tutorials and examples.